Generate PDF with QR Code from HTML Template in C#
In this article, you will see how to generate a PDF file with a QR code using an HTML template. To do so, you will be using the PDF.CO Web API with the C# client application.
The article consists of two parts:
- In the first part, you will see how to create an HTML template with placeholders using the PDF.CO web application.
- In the second part, you will see how to generate a PDF document using the HTML template that you created in the first part. You will also see how the PDF.CO Web API can be used to fill the placeholder in the HTML document.
How to Create an HTML Template with Placeholders
To create an HTML template with placeholders, go to the following link.
You need to log in to PDF.CO to create HTML templates with placeholders. Once you login into the above link, you will see some default HTML templates. You can create your own HTML template by clicking the “create new template” button, as shown in the screenshot below.
For this article, a sample HTML template named “QR_Code_Template” is created. The ID for this template is 260 as you can see in the following screenshot.
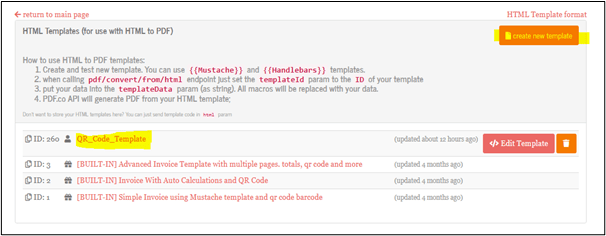
The body of our sample HTML template “QR_Code_Template” is as follows:
You can see that the HTML template contains one division with an H2 heading. Inside the H2 heading, we have two placeholders {{invoice_id}} and {{client_name}}.
A QR code is also generated using the values passed to the {{invoice_id}} and {{client_name}} placeholders.
<!doctype html>
<html lang="en-US">
<head>
<title>Invoice {{invoice_id}} {{client_name}}</title>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@4.6.1/dist/css/bootstrap.min.css" integrity="sha384-zCbKRCUGaJDkqS1kPbPd7TveP5iyJE0EjAuZQTgFLD2ylzuqKfdKlfG/eSrtxUkn" crossorigin="anonymous">
</head>
<body>
<div class="container">
<h2>File contains QR code with Invoice number {{invoice_id}} from client {{client_name}} </h2>
<center>
<img src="[[barcode: QRCode Invoice {{invoice_id}} {{client_name}} ]]" />
</center>
</div>
</body>
</html>
We have created our HTML template with placeholders. In the next section, you will see how you can use the PDF.CO Web API in C# to generate a PDF document using this HTML template. You will also see how to pass values for the {{invoice_id}} and {{client_name}} placeholders.
How to Generate PDF Using HTML Template with Placeholders
You will create a C# console application in this section that calls the PDF.CO Web API to generate PDF documents using HTML templates.
By the way, we have a similar tutorial where you can learn how to create a PDF invoice in C# using PDF and HTML templates as well.
Going back to our discussion: The first step is to import the required libraries as shown in the following script:
using System;
using System.Collections.Generic;
using System.IO;
using System.Net;
using Newtonsoft.Json;
using Newtonsoft.Json.Linq;
The Microsoft .NET framework contains all the above packages by default except the “Newtonsoft” library.
To install this library from Microsoft Visual Studio ID, go to “Tools-> NuGet Package Manager”. Search for “Newtonsoft” library. You will see the following packages. Select the first package and click the “Install” button.
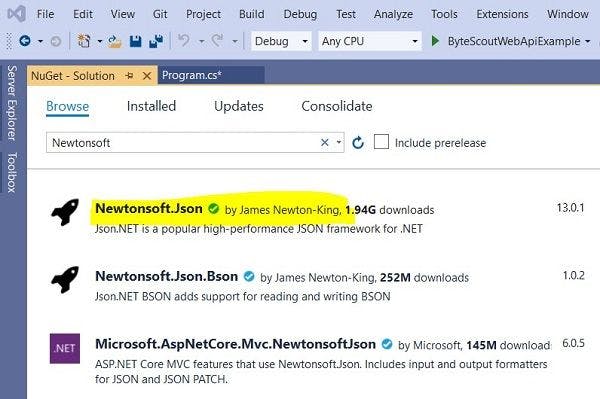
Inside the class, just before the “Main” method, declare two variables: one for the API key from PDF.CO, and the other for storing the destination path for the generated PDF document.
Note: You can get your API key by registering at https://app.pdf.co
const String API_KEY = "*******************************";
const string DestinationFile = @".\newQRDocument.pdf";
The rest of the script is executed inside the “Main” method.
To make requests to the PDF.CO Web API via C#, you need to create an object of the WebClient class. Pass your PDF.CO API key to the header of the WebClient object.
WebClient webClient = new WebClient();
webClient.Headers.Add("x-api-key", API_KEY);
Next, you need to define the API call that converts HTML templates to PDF using the PDF.CO API. The “url” variable in the following script stores the API call:
string url = "https://api.pdf.co/v1/pdf/convert/from/html";
The next step is to create a parameter dictionary that contains parameter values for your API call. The details of the parameters are available at the official documentation link.
The following script defines the parameter dictionary. The script also serializes the parameter dictionary.
Dictionary<string, object> parameters = new Dictionary<string, object>();
parameters.Add("templateId", 260);
parameters.Add("name", Path.GetFileName(DestinationFile));
parameters.Add("margins", "40px 20px 20px 20px");
parameters.Add("paperSize", "Letter");
parameters.Add("orientation", "Portrait");
parameters.Add("header", "");
parameters.Add("printBackground", true);
parameters.Add("footer", "");
parameters.Add("async", false);
parameters.Add("encrypt", false);
parameters.Add("templateData", "{\"invoice_id\": \"1002002\", \"client_name\": \"ABCD Electronics\"}");
string jsonPayload = JsonConvert.SerializeObject(parameters);
The most important parameters in the above script are the “templateId”, the “name”, and the “templateData”.
The “templateId” is the ID of the HTML template that you generated in the previous section and that you want to convert into a PDF document. In our case, the Id of the HTML template is 260.
The “name” parameter stores the destination path for the generated PDF document.
The “templateData” parameter contains a JSON dictionary in the form of C# string. This dictionary is used to fill data in the placeholders of the HTML template. For instance, the HTML template had place holders {{invoice_id}} and {{client_name}}. The “templateData” parameter passes values 1002002 and ABCD Electronics for these placeholders, respectively. Here backslash \” are used to escape the double quotations in a C# string.
The rest of the process is standard and should be executed inside a Try/Catch block.
You have to execute the call to the PDF.CO API using the “UploadString()” method of the WebClient class object. The response from the API call is parsed as a JSON object.
If the response doesn’t contain any error, the “URL” attribute of the JSON object is printed on the console. This URL contains the generated pdf document. The generated PDF file is downloaded from the URL.
In case the response contains an error, the error message is printed on the console. Here is the script for executing the API call and for parsing the response:
try
{
string response = webClient.UploadString(url, jsonPayload);
JObject json = JObject.Parse(response);
if (json["error"].ToObject<bool>() == false)
{
string resultFileUrl = json["url"].ToString();
Console.WriteLine(resultFileUrl);
webClient.DownloadFile(resultFileUrl, DestinationFile);
Console.WriteLine("Generated PDF file saved as \"{0}\" file.", DestinationFile);
}
else
{
Console.WriteLine(json["message"].ToString());
}
}
catch (WebException e)
{
Console.WriteLine(e.ToString());
}
Finally, you have to dispose of the WebClient object to destroy the connection between the C# client and the PDF.CO Web API.
The complete code for generating the PDF document using the HTML template is as follows:
Complete Code
using System;
using System.Collections.Generic;
using System.IO;
using System.Net;
using Newtonsoft.Json;
using Newtonsoft.Json.Linq;
namespace ByteScoutWebApiExample
{
class Program
{
const String API_KEY = "usmanmalik57@gmail.com_8df5671cedb0fb37ec9610eada409f110c38";
const string DestinationFile = @".\newQRDocument.pdf";
static void Main(string[] args)
{
WebClient webClient = new WebClient();
webClient.Headers.Add("x-api-key", API_KEY);
string url = "https://api.pdf.co/v1/pdf/convert/from/html";
Dictionary<string, object> parameters = new Dictionary<string, object>();
parameters.Add("templateId", 260);
parameters.Add("name", Path.GetFileName(DestinationFile));
parameters.Add("margins", "40px 20px 20px 20px");
parameters.Add("paperSize", "Letter");
parameters.Add("orientation", "Portrait");
parameters.Add("header", "");
parameters.Add("printBackground", true);
parameters.Add("footer", "");
parameters.Add("async", false);
parameters.Add("encrypt", false);
parameters.Add("templateData", "{\"invoice_id\": \"1002002\", \"client_name\": \"ABCD Electronics\"}");
string jsonPayload = JsonConvert.SerializeObject(parameters);
try
{
string response = webClient.UploadString(url, jsonPayload);
JObject json = JObject.Parse(response);
if (json["error"].ToObject<bool>() == false)
{
string resultFileUrl = json["url"].ToString();
Console.WriteLine(resultFileUrl);
webClient.DownloadFile(resultFileUrl, DestinationFile);
Console.WriteLine("Generated PDF file saved as \"{0}\" file.", DestinationFile);
}
else
{
Console.WriteLine(json["message"].ToString());
}
}
catch (WebException e)
{
Console.WriteLine(e.ToString());
}
webClient.Dispose();
Console.WriteLine();
Console.WriteLine("Press any key...");
Console.ReadKey();
}
}
}
When you run the above script, a PDF file named “newQRDocument.pdf” will be generated in your local directory. The PDF file will look like this:
Output
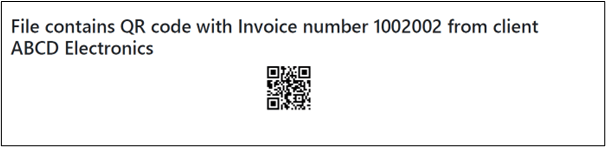
You can see that the {{invoice_id}} and the {{client_name}} placeholders in the HTML template are replaced by the client id and the client name that you passed via the API call to the PDF.CO Web API. Also, a barcode is generated using the values passed to the {{invoice_id}} and the {{client_name}} placeholders.
To practice, you can try to add more placeholders in your HTML template and then use the PDF.CO Web API in C# to generate PDF documents by passing values for the placeholders in the HTML template.
We also have other related tutorials to this subject that can teach you other cool stuff you can do with PDF.co Web API in C# such as generating PDFs by merging different file formats, and a lot more.
Related Tutorials
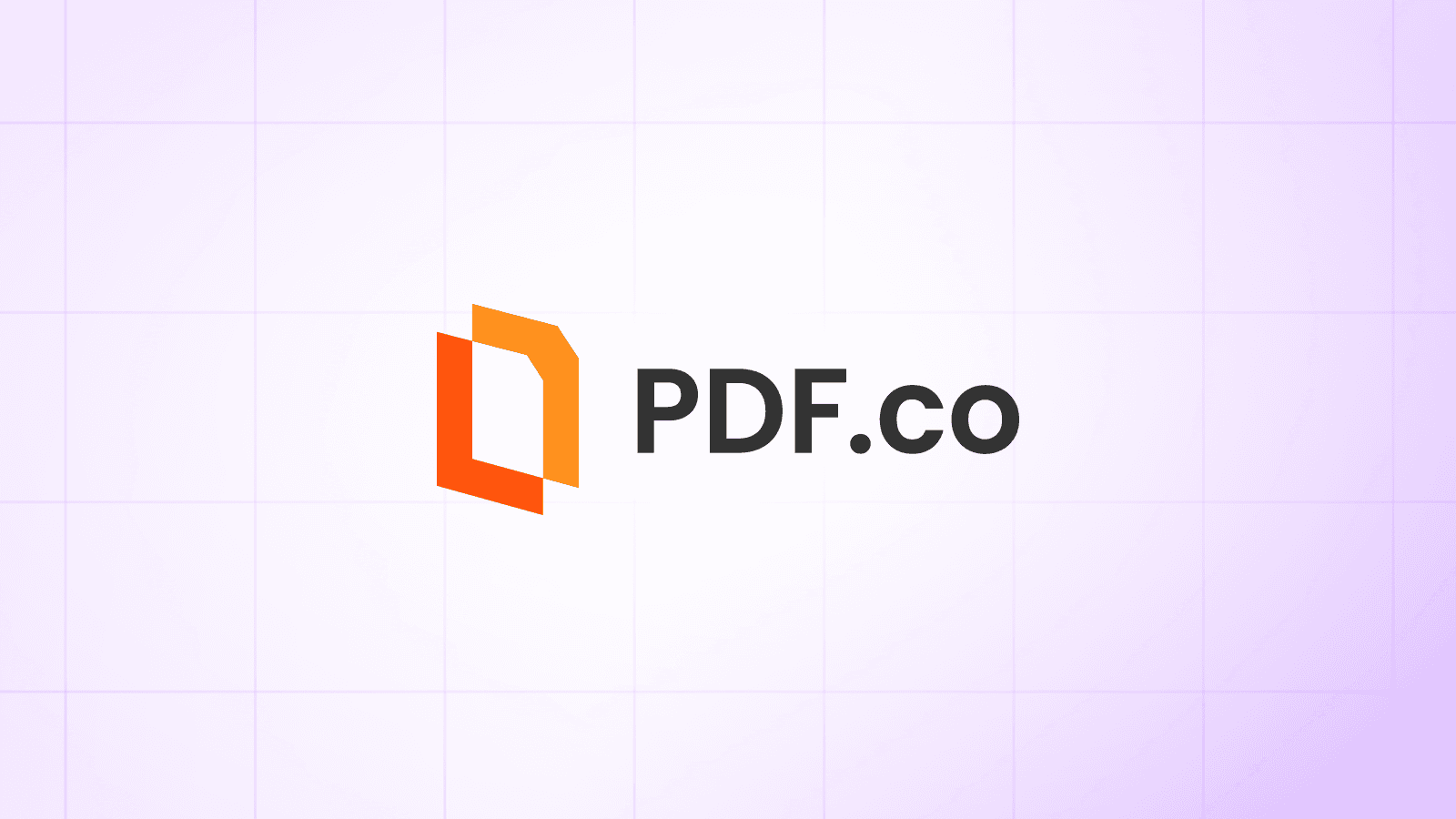
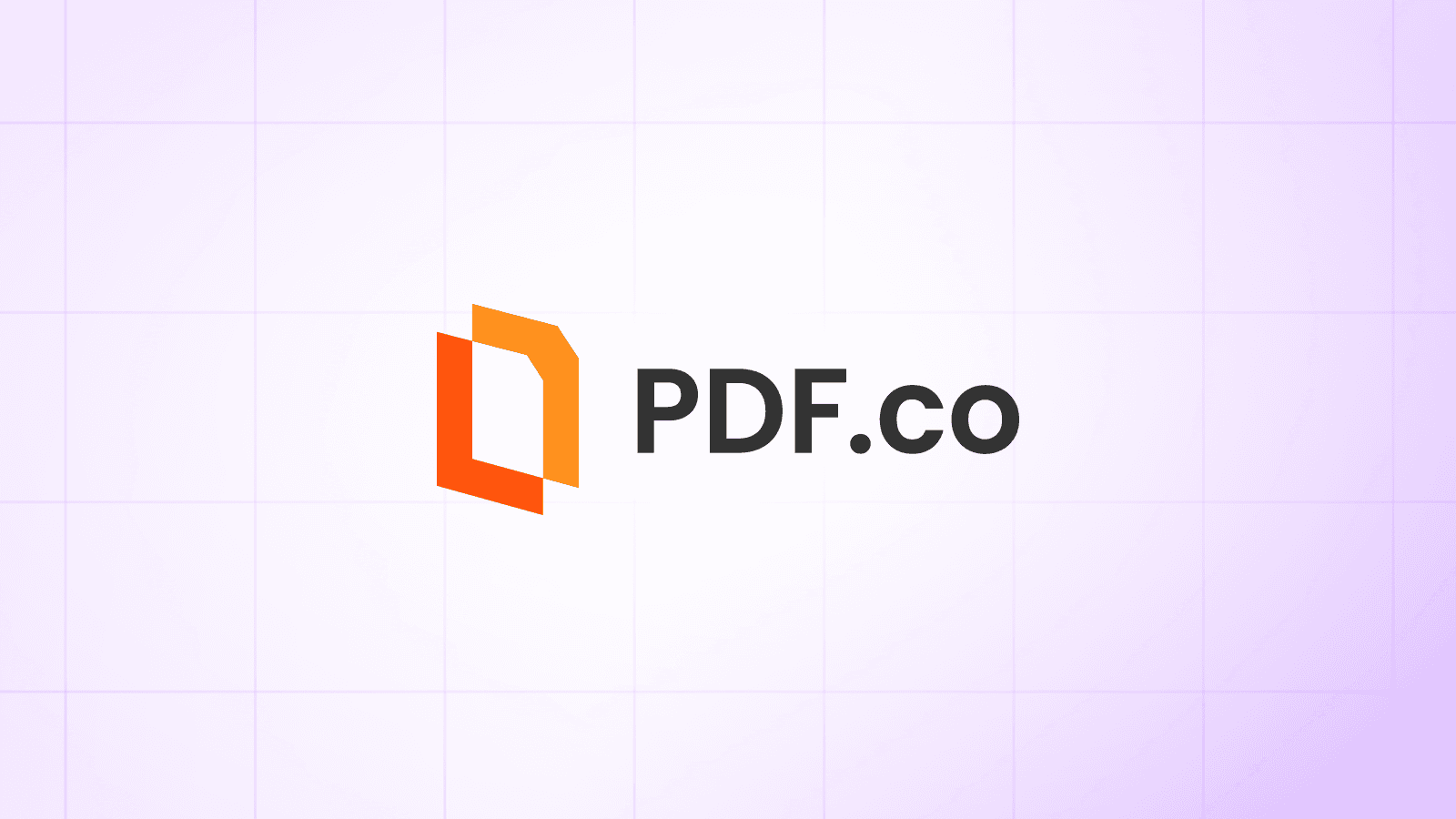
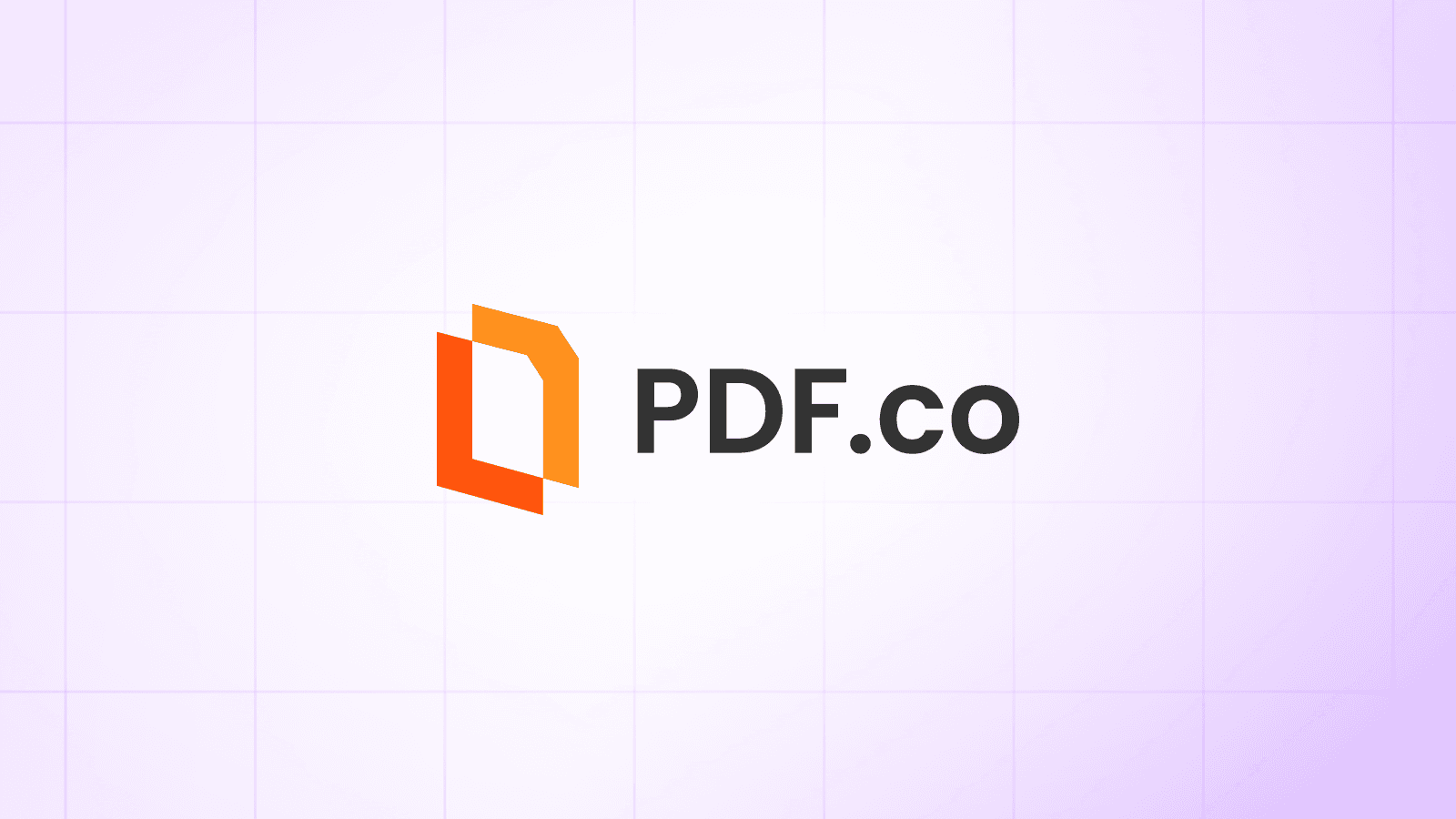
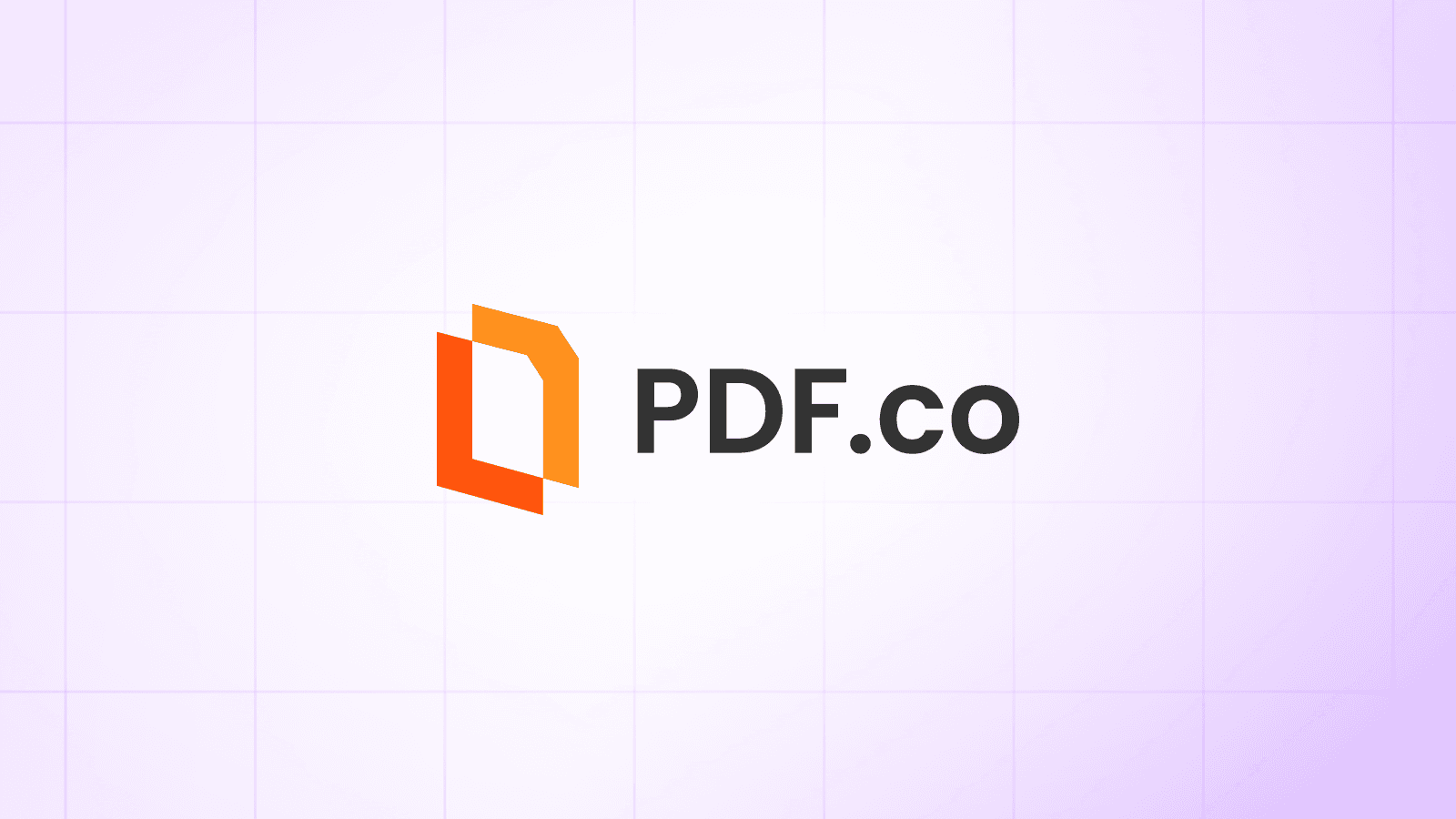