Convert HTML/URL to PDF with SharePoint and PDF.co
In this short article, we’ll demonstrate source code for how to convert HTML/URL to PDF using SharePoint and PDF.co. Let’s get into source code.
Convert HTML to PDF – Source Code Snippets
Check out the markup for Web Part visual control.
VisualWebPart1UserControl.ascx
<%@ Assembly Name="$SharePoint.Project.AssemblyFullName$" %>
<%@ Assembly Name="Microsoft.Web.CommandUI, Version=15.0.0.0, Culture=neutral, PublicKeyToken=71e9bce111e9429c" %>
<%@ Register Tagprefix="SharePoint" Namespace="Microsoft.SharePoint.WebControls" Assembly="Microsoft.SharePoint, Version=15.0.0.0, Culture=neutral, PublicKeyToken=71e9bce111e9429c" %>
<%@ Register Tagprefix="Utilities" Namespace="Microsoft.SharePoint.Utilities" Assembly="Microsoft.SharePoint, Version=15.0.0.0, Culture=neutral, PublicKeyToken=71e9bce111e9429c" %>
<%@ Register Tagprefix="asp" Namespace="System.Web.UI" Assembly="System.Web.Extensions, Version=4.0.0.0, Culture=neutral, PublicKeyToken=31bf3856ad364e35" %>
<%@ Import Namespace="Microsoft.SharePoint" %>
<%@ Register Tagprefix="WebPartPages" Namespace="Microsoft.SharePoint.WebPartPages" Assembly="Microsoft.SharePoint, Version=15.0.0.0, Culture=neutral, PublicKeyToken=71e9bce111e9429c" %>
<%@ Control Language="C#" AutoEventWireup="true" CodeBehind="VisualWebPart1UserControl.ascx.cs" Inherits="ConvertWebPageToPDFLinkWebPart.VisualWebPart1.VisualWebPart1UserControl" %>
Page url<br />
<asp:TextBox ID="UrlTextBox" runat="server" Width="600px">https://en.wikipedia.org/wiki/Main_Page</asp:TextBox>
<br />
<br />
<asp:Button ID="StartButton" runat="server" OnClick="StartButton_Click" Text="Start" style="width: 610px; padding-left: 0px; margin-left: 0px; padding-right: 0px; padding-right: 0px;"/>
<br />
<br />
Log<br />
<asp:TextBox ID="LogTextBox" runat="server" Height="300px" TextMode="MultiLine" Width="600px"></asp:TextBox>
<br />
This is code behind for Web Part User Control.
VisualWebPart1UserControl.ascx.cs
using Microsoft.SharePoint;
using Newtonsoft.Json;
using Newtonsoft.Json.Linq;
using System;
using System.Collections.Generic;
using System.IO;
using System.Net;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
namespace ConvertWebPageToPDFLinkWebPart.VisualWebPart1
{
public partial class VisualWebPart1UserControl : UserControl
{
public SPWeb CurrentWeb { get; set; }
// The authentication key (API Key).
// Get your own by registering at https://app.pdf.co/documentation/api
string API_KEY = Utils.API_KEY;
// Destination PDF file name
const string DestinationFile = "result.pdf";
const string DestinationLibName = "Shared Documents";
protected void Page_Load(object sender, EventArgs e)
{
}
protected void StartButton_Click(object sender, EventArgs e)
{
// Create standard .NET web client instance
ServicePointManager.Expect100Continue = true;
ServicePointManager.SecurityProtocol = SecurityProtocolType.Tls12;
WebClient webClient = new WebClient();
var SourceUrl = UrlTextBox.Text;
if (String.IsNullOrWhiteSpace(SourceUrl))
{
LogTextBox.Text += "Enter valid web page url \n";
return;
}
// Set API Key
webClient.Headers.Add("x-api-key", API_KEY);
// URL for `Web Page to PDF` API call
string url = "https://api.pdf.co/v1/pdf/convert/from/url";
// Prepare requests params as JSON
Dictionary<string, object> requestBody = new Dictionary<string, object>();
requestBody.Add("name", DestinationFile);
requestBody.Add("url", SourceUrl);
// Convert dictionary of params to JSON
string jsonPayload = JsonConvert.SerializeObject(requestBody);
try
{
// Execute POST request
var response = webClient.UploadString(url, "POST", jsonPayload);
// Parse JSON response
JObject json = JObject.Parse(response);
if (json["error"].ToObject() == false)
{
// Get URL of generated PDF file
string resultFileUrl = json["url"].ToString();
// Download PDF file
var retData = webClient.DownloadData(resultFileUrl);
//Upload file to SharePoint document library
//Read create stream
using (MemoryStream stream = new MemoryStream(retData))
{
//Get handle of library
SPFolder spLibrary = CurrentWeb.Folders[DestinationLibName];
//Replace existing file
var replaceExistingFile = true;
//Upload document to library
SPFile spfile = spLibrary.Files.Add(DestinationFile, stream, replaceExistingFile);
spLibrary.Update();
}
LogTextBox.Text += String.Format("Generated PDF document saved as \"{0}\\{1}\" file. \n", DestinationLibName, DestinationFile);
}
else
{
LogTextBox.Text += json["message"].ToString() + " \n";
}
}
catch (Exception ex)
{
LogTextBox.Text += ex.ToString() + " \n";
}
webClient.Dispose();
LogTextBox.Text += "\n";
LogTextBox.Text += "Done...\n";
}
}
}
Source Code at GitHub
You can explore the full source code for this sample at this GitHub link.
Convert HTML to PDF – Sample Screenshots
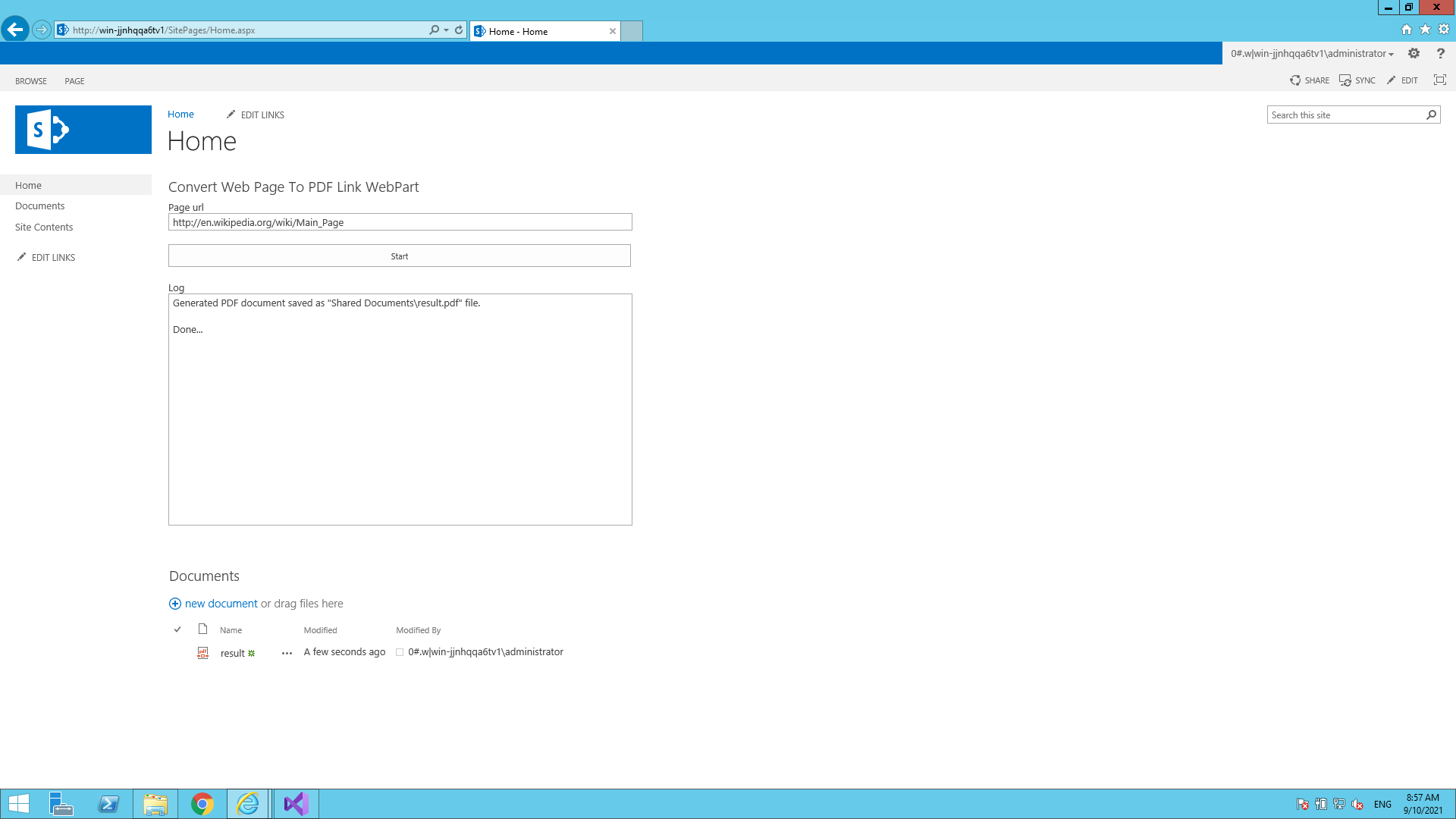
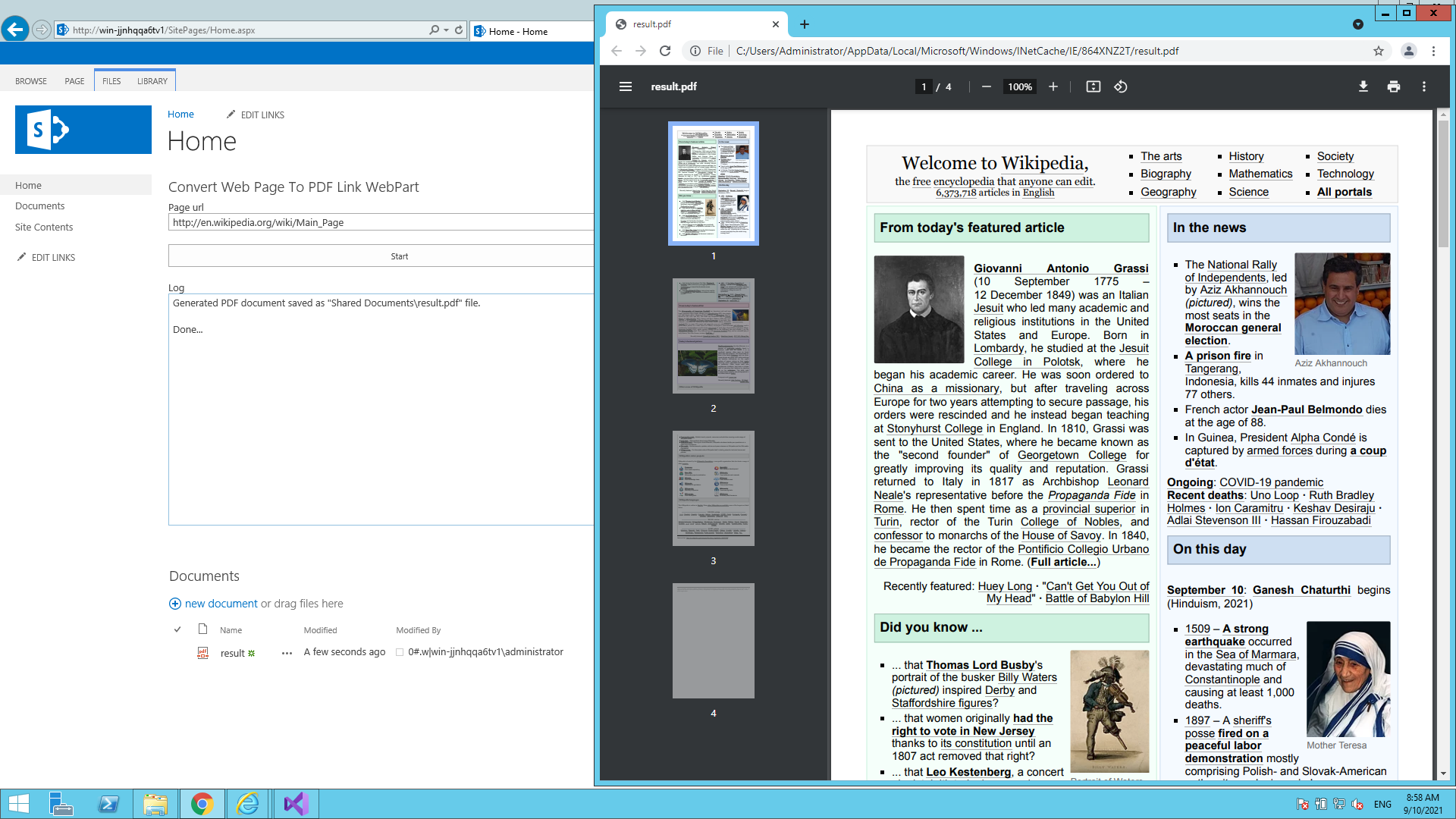
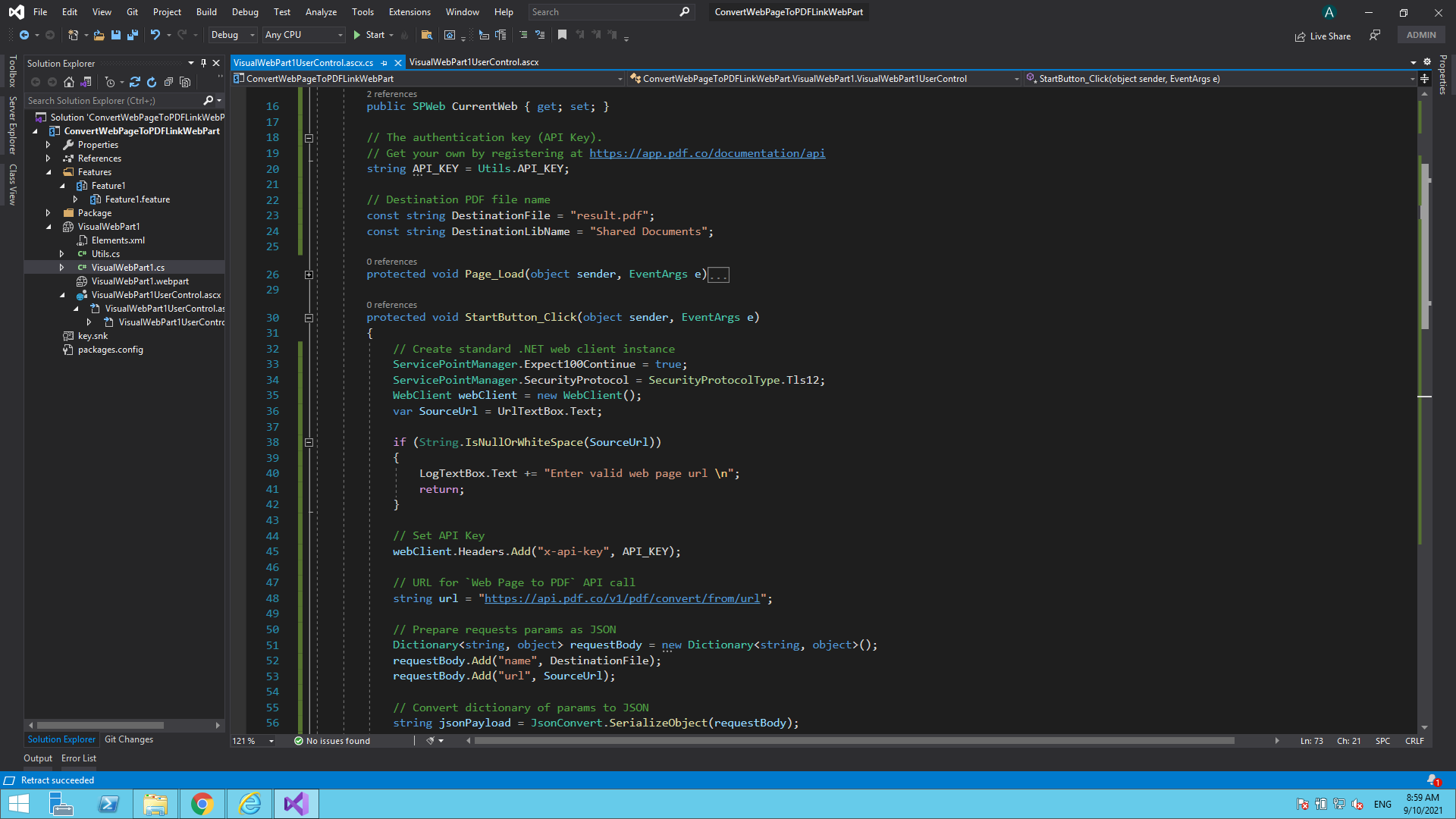
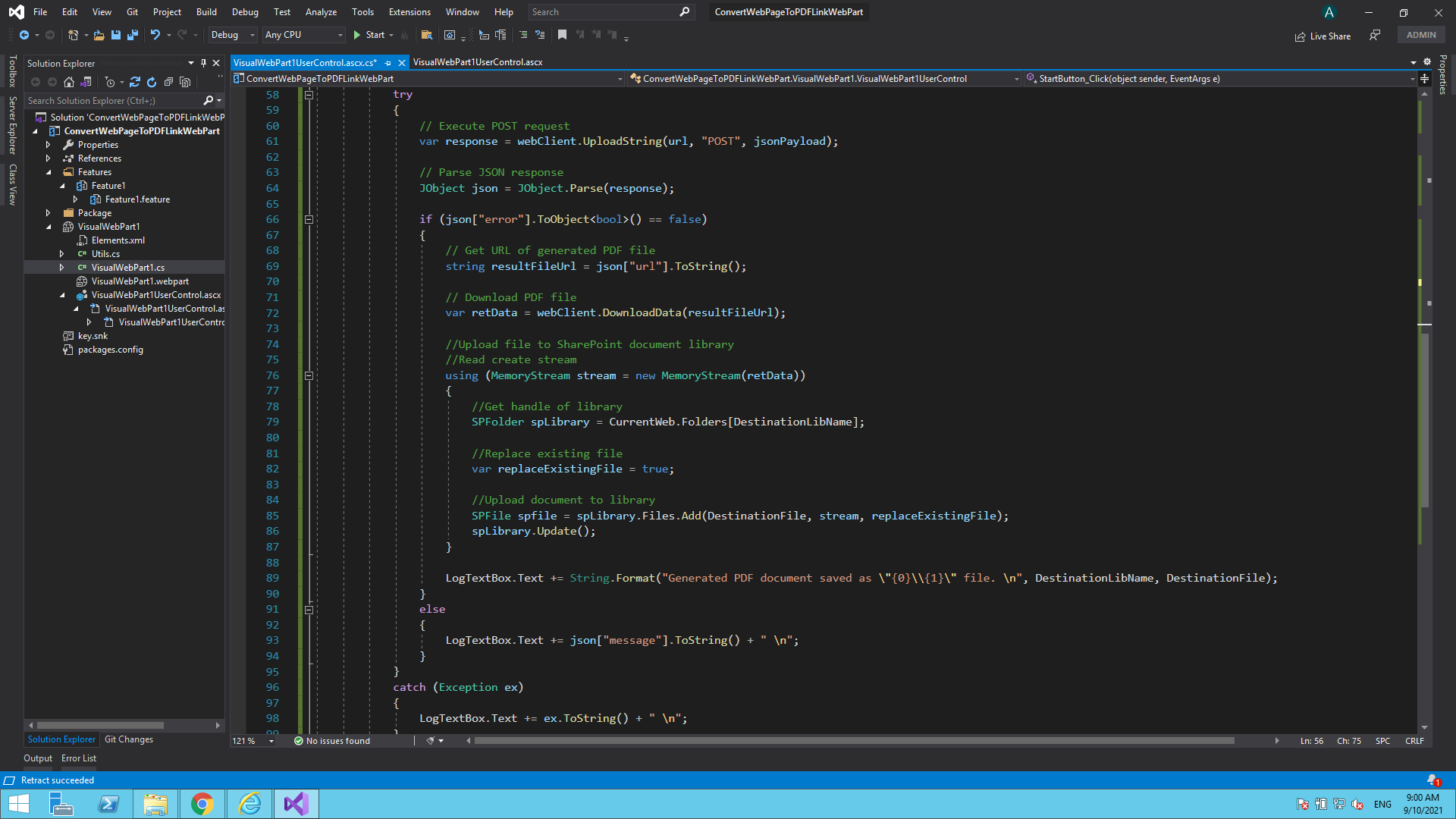
I hope this tutorial and code snippet is useful to you. Please try it yourself to get to know more.