How to Use PDF Template with Built-in Barcodes at PDF.co
This tutorial and the sample source code explain generating a PDF using an HTML template with built-in barcodes. The users can follow this Python tutorial and use PDF.co’s “Convert HTML template to PDF” API adds different kinds of barcodes to their PDFs.
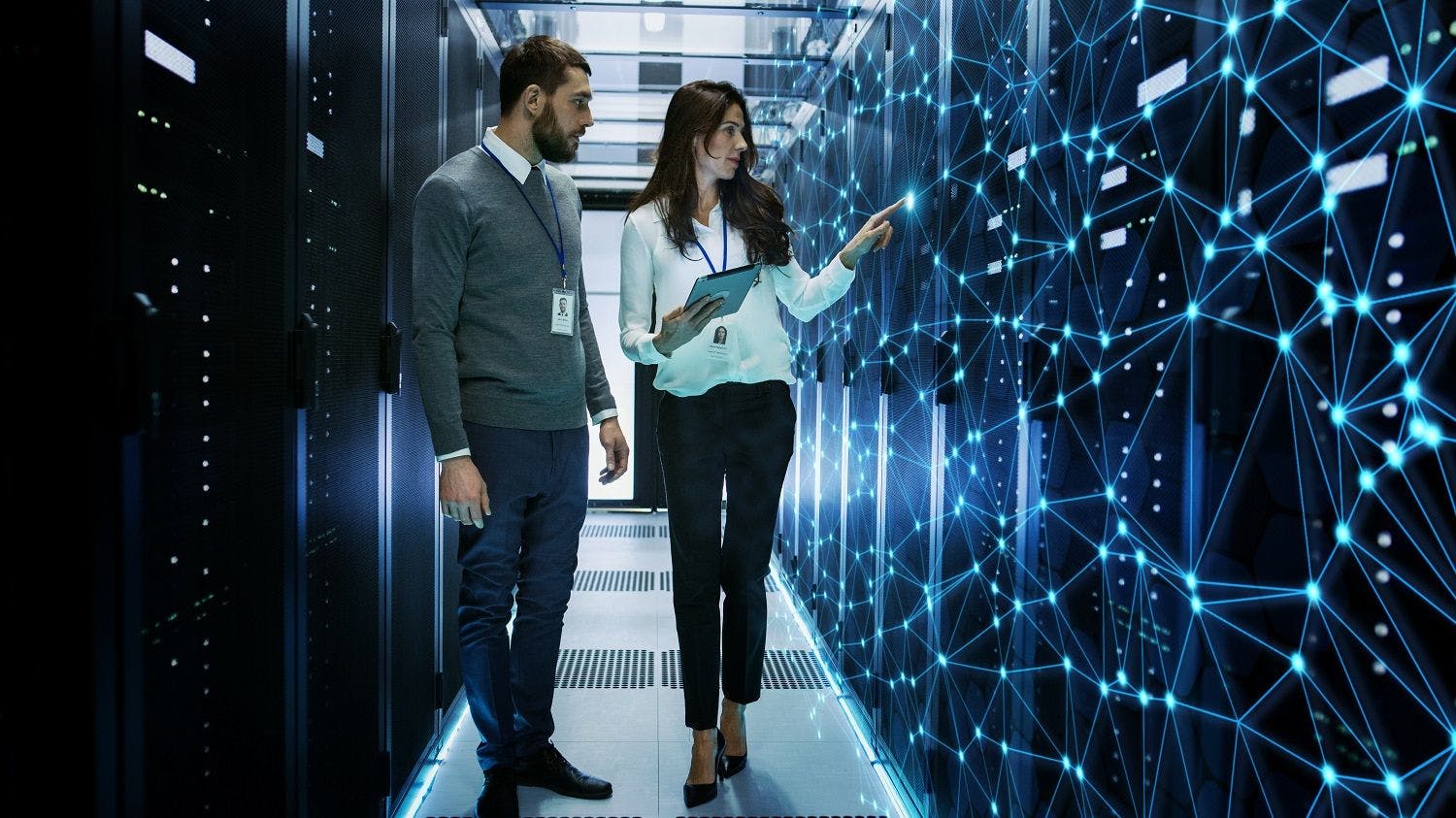
Features of HTML Template to PDF Web API
The API’s name suggests that the users can convert their HTML file or template to PDF using this conversion API. However, it converts the HTML template and provides various programming benefits to the users. Following are the features of this API:
- The users can create and reuse their templates, images, codes such as Javascript, CSS, HTML5 styles, built-in barcodes such as QR Code, Code 39, and Datamatrix, custom fonts, and conditional logic.
- This API provides multiple source support, due to which the users can use this API to generate reports from CSV or JSON data.
- This API provides handlebars and mustache HTML templates. The users can use the special macros available to insert variable data in the HTML template. For instance, in this tutorial, the user inserts a barcode in the HTML template using the barcode macro.
- In addition to the above, it also provides support for custom scripts that the users can use to make the dynamic PDF. For instance, the users can choose to hide or show HTML elements or modify the link functionality in the resulting PDF.
- Most importantly, the API gives the control of choosing the paper size, header, footer, paper margin, and page orientation ( portrait, landscape) to the user to generate the PDF of their choice.
Endpoint Parameters
Following are the parameters of Convert HTML Template to PDF API:
HTML
- The only required parameter accepts HTML templates with Mustache and Handles templates. The users can use a separate HTML file and read them in the code or add the inline HTML directly in the parameter. The users will be creating an HTML element using this parameter to add barcodes in this tutorial.margins
- It is an optional parameter that lets the user set margins in CSS style such as 2x 2x 2x. 2x for top, right, bottom, and left, respectively.orientation
- The users can set the page orientation to portrait or landscape using this optional parameter. Its value is “portrait” by default.paperSize
- It is an optional parameter that lets the users set the paperSize of the resulting PDF. Its value is set to “letter” by default. The users can select legal, tabloid, letter, A0, A1, A2, A3, A4, A5, A6, or any custom size. These custom sizes can be in pixels, millimeters, centimeters, and inches.printBackground
- It is an optional parameter that lets the users enable or disable background printing. It is set to “true” by default.header
- It is an optional parameter that lets the users add header HTML for the PDF file.footer
- It is an optional parameter in which the users add the footer section to every page of the resulting PDF file.async
- It is an optional parameter that lets the user choose whether they want to perform this job asynchronously or not. Its value is “false” by default.templateData
- An optional parameter lets the user insert values into the HTML macros. The users will be using this parameter to insert the barcode value to the barcode variable in this tutorial.profiles
- It is an optional parameter that lets the user set custom configurations in a string.
Use PDF.co to Convert HTML File to PDF and to Add Built-in Barcodes
The following python source code is a sample example to demonstrate the use of the PDF.co “Convert HTML Template to PDF file” in inserting barcodes into the PDF files. The user is using the basic inline HTML to create an image tag in which he has to insert the built-in barcode. It is important to note that, as discussed earlier, the users can use any barcode type such as QR Code, Code 39, Datamatrix, and various others while calling this API. However, in this tutorial, the user generates the QR Code to show the barcode usage.
Moreover, this code shows how the users can use the macros to make the templates slightly dynamic. This code lets the users add the barcode value using the endpoint’s templateData parameter. Therefore, they can easily modify its value whenever needed without changing the actual HTML code.
Additionally, the code runs asynchronously and uses the HTML template to create a PDF file, which the users can save on their computer or can open it using the provided link. The PDF.co Convert HTML Template to PDF API takes in the HTML and the templateData endpoint and inserts a barcode in the PDF file.
Sample Code Snippet
Following is an example of a PDF template using a built-in code snippet:
import os
import requests # pip install requests
# The authentication key (API Key).
# Get your own by registering at https://app.pdf.co
API KEY = "***************"
# Base URL for PDF.co Web API requests
BASE_URL = "https://api.pdf.co/v1"
# Runs processing asynchronously. Returns Use JobId that you may use with /job/check to check state of the processing (possible states: working, failed, aborted and success). Must be one of: true, false.
Async = "False"
# Values to fill out pdf fields with built-in pdf form filler.
# To fill fields in PDF form, use the following format page;fieldName;value for example: 0;editbox1;text is here. To fill checkbox, use true, for example: 0;checkbox1;true. To separate multiple objects, use | separator. To get the list of all fillable fields in PDF form please use /pdf/info/fields endpoint.
def main(args = None):
convertFromHTML()
def convertFromHTML():
"""Converts HTML template to PDF using PDF.co Web API"""
# Prepare requests params as JSON
# See documentation: https://apidocs.pdf.co
parameters = {}
parameters["html"] ="<div><img src=\" [[ barcode:QRCode {{variable1}}
]]\" /></div> "
parameters["templateData"] = "{\"variable1\": \"12345\"}"
parameters["name"] = "result.pdf"
parameters["async"] = Async
# Prepare URL for 'Convert from HTML' API request
url = "{}/pdf/convert/from/html".format(BASE_URL)
# Execute request and get response as JSON
response = requests.post(url, data=parameters, headers={ "x-api-key":
API_KEY })
if (response.status_code == 200):
json = response.json()
print(json)
else:
print(f"Request error: {response.status_code} {response.reason}")
if (__name__ == '__main__'):
main()
Step-by-Step Guide to Use Barcode in PDF Template
Below is the step-by-step guide to insert barcode in the HTML template and convert it to PDF:
- Import the necessary modules to make API requests. Mostly, python does not have requests modules by default. Therefore, the users may need to install it using the “pip install requests” command.
- Declare and initialize the needed variables such as API_Key, async, and BASE_URL. The users can insert these values directly in the API requests or declare them at the top of the code to make modifying these variables easier. In this way, they can change these variables any time in the future without reading the full code or altering the API request. Moreover, each user has their own unique API_KEY, which he must insert to make an API request. They can easily get these keys by signing up/into their PDF.co account by going on this link.
- Define the conversion method to call the “Convert HTML to PDF” API. Write inline HTML to create an image element to display the barcode and use macros for the barcode value. The sample code is explicitly mentioning the barcode type as “QRCode”. Then, give the barcode value in the templateData in the format defined in the example code. Moreover, name the resulting PDF file as “result.pdf” and set the “async” value to “true” to run the program asynchronously. Lastly, define the success and failure cases of the API and end the method.
- Call this method in the main function, which will call the PDF.co conversion API and generate a PDF from the HTML template containing the barcode.
Output File
Below is the resulting PDF file, named “result.pdf”:
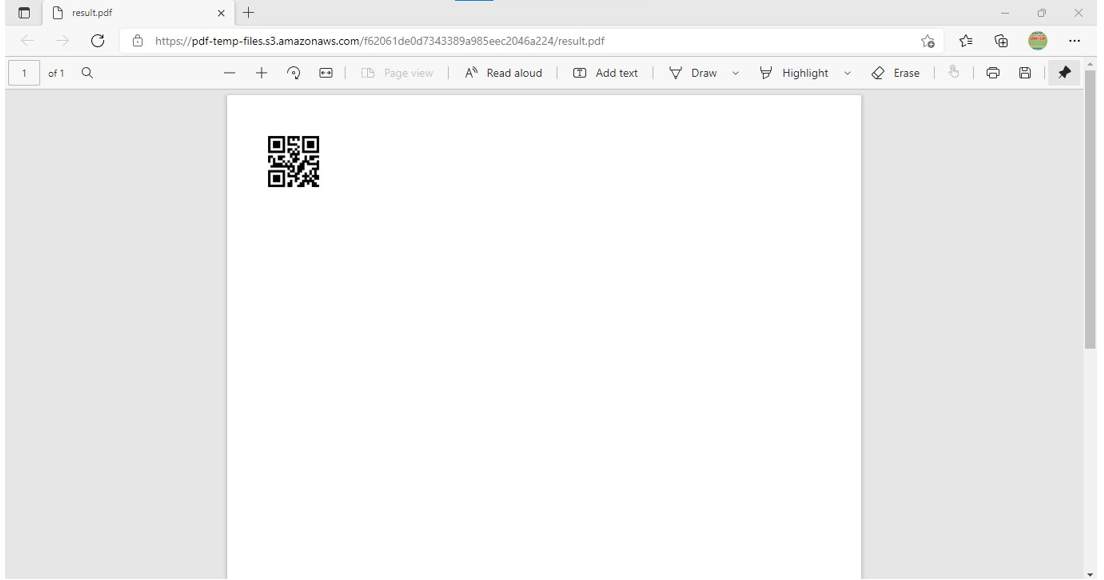