If the users are working with the JSON input, they have to make sure to escape it using “JSON.stringify(dataObject) in JavaScript. Escaping means replacing every “ with \”. Most programming languages perform it automatically. However, the users must ensure this replacement step while working with JavaScript.
Create PDF with JavaScript using HTML Invoice Template
Learn about a fast and nice way to create PDF with JavaScript using HTML invoice template functionality from PDF.co Web API. Using JSON, users can use PDF from HTML template API to efficiently create a PDF document of the invoice regarding their respective data.
Features of PDF from HTML Template API Endpoint
The PDF.co Web API has beneficial tools and functionalities to generate an invoice from specific user data provided effectively. The PDF.co API provides support for {{Mustache}} style HTML templates. The Mustache templates got their name from the syntax where they contain tag names in curly brackets “{{ }}” resembling a mustache. These templates contain a model object having the data for the template.
The users can use JSON to set their “templateData” property. For example, the users can use “{ invoice: { company: \”Test\”}}” syntax, and the template will change the “{{invoice.company}}” to the Test company. This API endpoint provides automation and scaling to generate PDF by loading the data from the “templateData” parameter and using that data to fill the HTML template.
Apart from the working of the API, the most critical thing to consider here is the PDF.co Web API provides its consumers with a highly secure platform. The API is secure because it transmits the user’s data via encrypted connections. The users can verify the security protocol here.
Endpoint Parameters for PDF from HTML Feature
Following are the PDF parameters from HTML Template API:
- templateId: It is a required parameter. This parameter is set to the ID of a user’s HTML template. The users can find and copy a specific ID from the HTML templates section in the application here.
- templateData: It is also a required parameter. This parameter stores a string. Users must set this parameter to a string with the input JSON data. The users can also utilize the recommended JSON data as the input, or they can use the data from the csv.
- margins: It is an optional parameter. It is set to ss styles for all the sides or in the order of top, right, bottom, and left. For example, “5px 5px 5px 5px”.
- orientation: It is an optional parameter. It is set to Portrait by default, but the users can change it to Landscape.
- paperSize: It is an optional parameter. It is set to Letter by default. The users can use other options like Legal, Ledger, Tabloid, A0, A1, A2, A3, A4, A5, A6, or any customized size. The customized size could be in pixels or inches with customized width and height.
- printBackground: It is an optional parameter. The parameter is set to true by default, but the users can change it to false to disable the background printing.
- mediaType: It is an optional parameter. It is set to print by default. Here, the print option is used to convert the HTML as it appears for printing, the screen option is used to convert HTML as it appears in a browser, and the none option sets the parameter to none.
- header: It is an optional parameter. It is set to HTML for the header to be applied on every page at the top.
- footer: It is an optional parameter. It is set to HTML for the footer to be applied on every page at the bottom.
Create PDF Invoice – Example using JavaScript
The following source code explains to the users how to create a PDF invoice using the PDF.co PDF from HTML Templates API. The sample code in JavaScript explains to the users how to create their HTML template and get the ID of the new template.
The next thing is to prepare the request for the API endpoint. Here, the file Invoice_data.json contains vital information and data about a client. The general template file will use JSON data to create a customer’s PDF invoice.
Moreover, the code.js file will connect the JSON file and the HTML template by making the API call. This process will generate the result.pdf file containing the invoice for a specific user regarding their customized data. Following are the files for JSON and JavaScript for the users’ clarification.
NOTE: In case you’re interested, we have a similar tutorial for creating PDF invoices using HTML templates in C#.
Sample Code for PDF Invoice Generation
Following is the sample code in JavaScript to create a PDF invoice using the HTML template:
var https = require("https");
var path = require("path");
var fs = require("fs");
// Get your key by registering at https://app.pdf.co
const API_KEY = "***********************************";
// Data to fill the template
const templateData = "./invoice_data.json";
// Destination PDF file name
const DestinationFile = "./result.pdf";
/*
Follow below steps to create your own HTML Template and get "templateId".
Add new html template in app.pdf.co/templates/html
Copy paste your html template code into this new template. Sample HTML templates can be found at "https://github.com/bytescout/pdf-co-api-samples/tree/master/PDF%20from%20HTML%20template/TEMPLATES-SAMPLES"
Save this new template
Copy it’s ID to clipboard
Now set ID of the template into “templateId” parameter
*/
// HTML template using built-in template
// see https://app.pdf.co/templates/html/2/edit
const template_id = 398;
// Prepare request to `HTML To PDF` API endpoint
var queryPath = `/v1/pdf/convert/from/html?name=${path.basename(DestinationFile)}`;
var reqOptions = {
host: "api.pdf.co",
path: encodeURI(queryPath),
method: "POST",
headers: {
"x-api-key": API_KEY,
"Content-Type": "application/json"
}
};
var requestBody = JSON.stringify({
"templateId": template_id,
"templateData": fs.readFileSync(templateData, "utf8")
});
// Send request
var postRequest = https.request(reqOptions, (response) => {
response.on("data", (d) => {
// Parse JSON response
var data = JSON.parse(d);
if (data.error == false) {
// Download PDF file
var file = fs.createWriteStream(DestinationFile);
https.get(data.url, (response2) => {
response2.pipe(file)
.on("close", () => {
console.log(`Generated PDF file saved as "${DestinationFile}" file.`);
});
});
}
else {
// Service reported error
console.log(data.message);
}
});
}).on("error", (e) => {
// Request error
console.log(e);
});
// Write request data
postRequest.write(requestBody);
postRequest.end();
Below is the sample data for a user invoice in JSON format:
{
"invoice_id": "1234567",
"invoice_date": "April 30, 2016",
"invoice_dateDue": "May 15, 2016",
"paid": false,
"issuer_name": "Acme Inc",
"issuer_company": "Acme International",
"issuer_address": "City, Street 3rd",
"issuer_email": "support@example.com",
"issuer_website": "https://example.com",
"client_name": "Food Delivery Inc.",
"client_company": "Food Delivery International",
"client_address": "New York, Some Street, 42",
"client_email": "client@example.com",
"items": [
{
"name": "Setting up new web-site",
"price": 250
},
{
"name": "Website Content Addition",
"price": 700
},
{
"name": "Database Setup",
"price": 200
},
{
"name": "Record Digitalization",
"price": 1800
},
{
"name": "Cloud Storage",
"price": 500
},
{
"name": "Short Messages",
"price": 35
},
{
"name": "Search Engine Optimization",
"price": 200
},
{
"name": "Priority Support",
"price": 75
},
{
"name": "Configuring mail server and mailboxes",
"price": 50
}
],
"tax": 0.065,
"discount": 0.01,
"note": "Thank You For Your Business!"
}
Step-by-Step Guide to Creating PDF Invoice using HTML Templates
- The code imports the necessary packages to make the API request and download and store the output file on the local storage, i.e., https, path, and fs packages.
- It then declares and initiates the API_KEY and template id variables needed to make the API request to PDF.co Web API. The users can get their API Key by signing up or logging in to the PDF.co website.
- Template id is the HTML template the user wants to use to generate the PDF invoice. The users can add their customized templates here and use the generated id in the API request to create the API invoice. PDF.co provides some sample templates from which the code uses the template with id “2”.
- The invoice_data.json file contains all the data the user wants to fill in the template parameters to customize for each customer. The user has to change the JSON file to modify the data in the invoice, keeping the same template.
- The code specifies the destination file, i.e., the output PDF template file, the template data file, i.e., invoice_data.json, and then the template id, i.e., the HTML template file containing the invoice template.
- It then sends the POST request to the API endpoint with the above variables in its payload and receives the API response. It checks for any error and prints it on the console. Otherwise, it downloads the file using file stream and stores it on the user’s local storage as the result.pdf and ends the request.
PDF Invoice Generated – Output
Below is the screenshot of the output PDF invoice file generated using HTML templates:
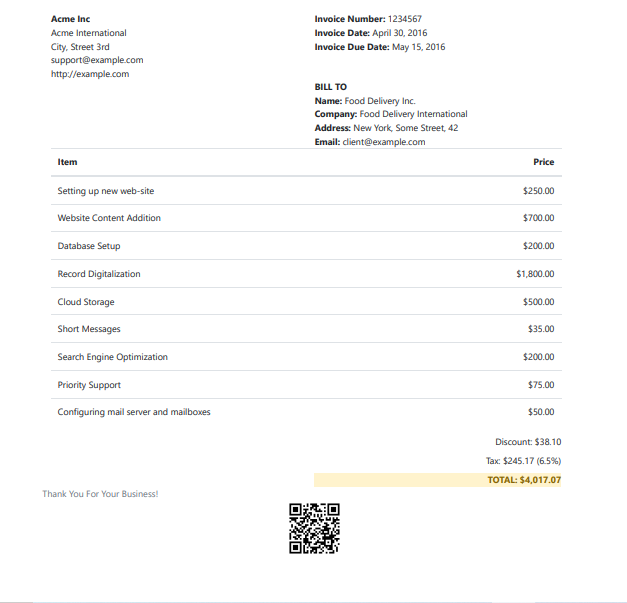
If you’ll notice above, the invoice has a QR code. Another cool functionality of the PDF.co app is that enables you to generate invoices with a barcode or QR code. Click on the link if want to know more about this feature.
Related Tutorials
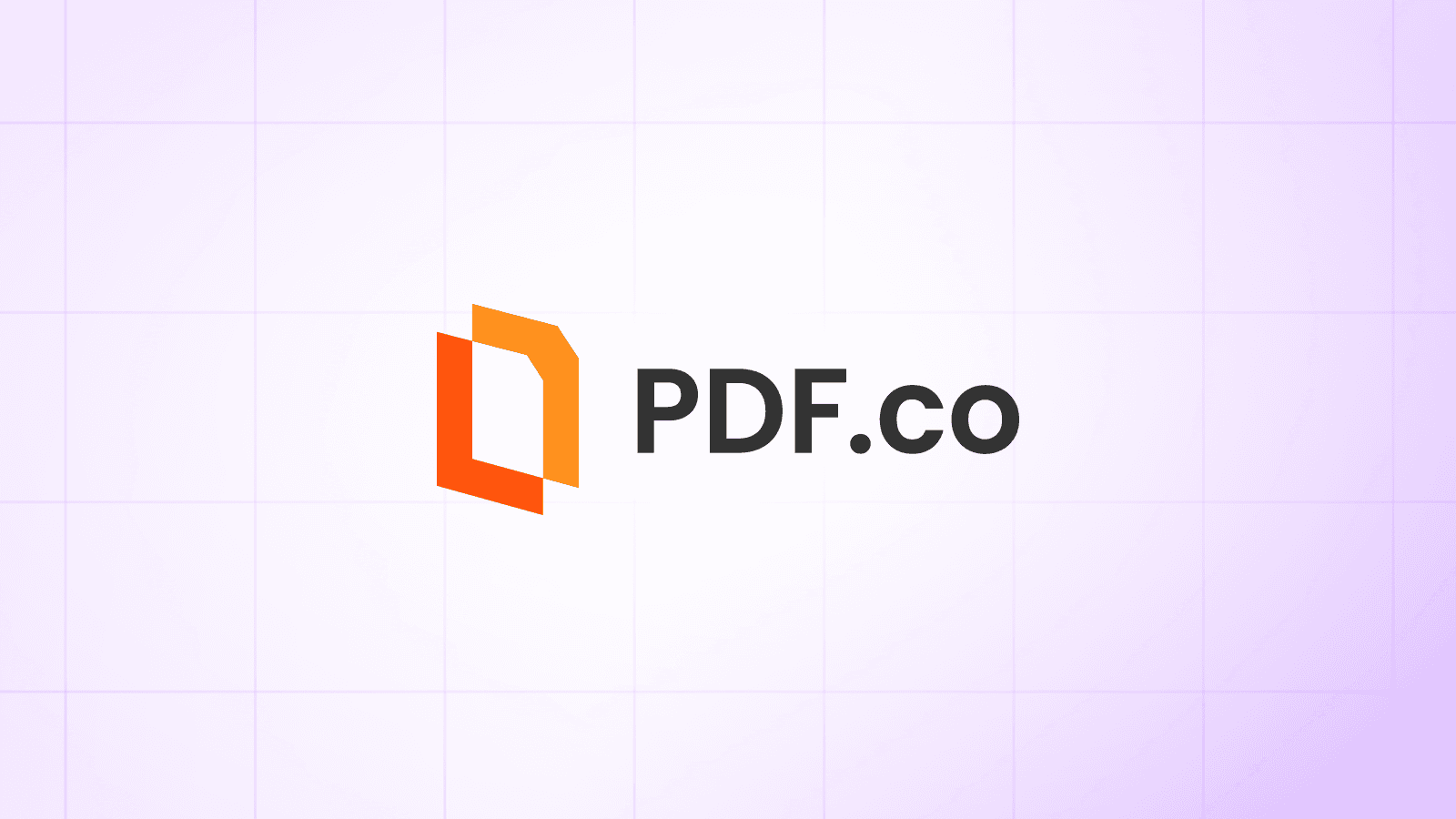
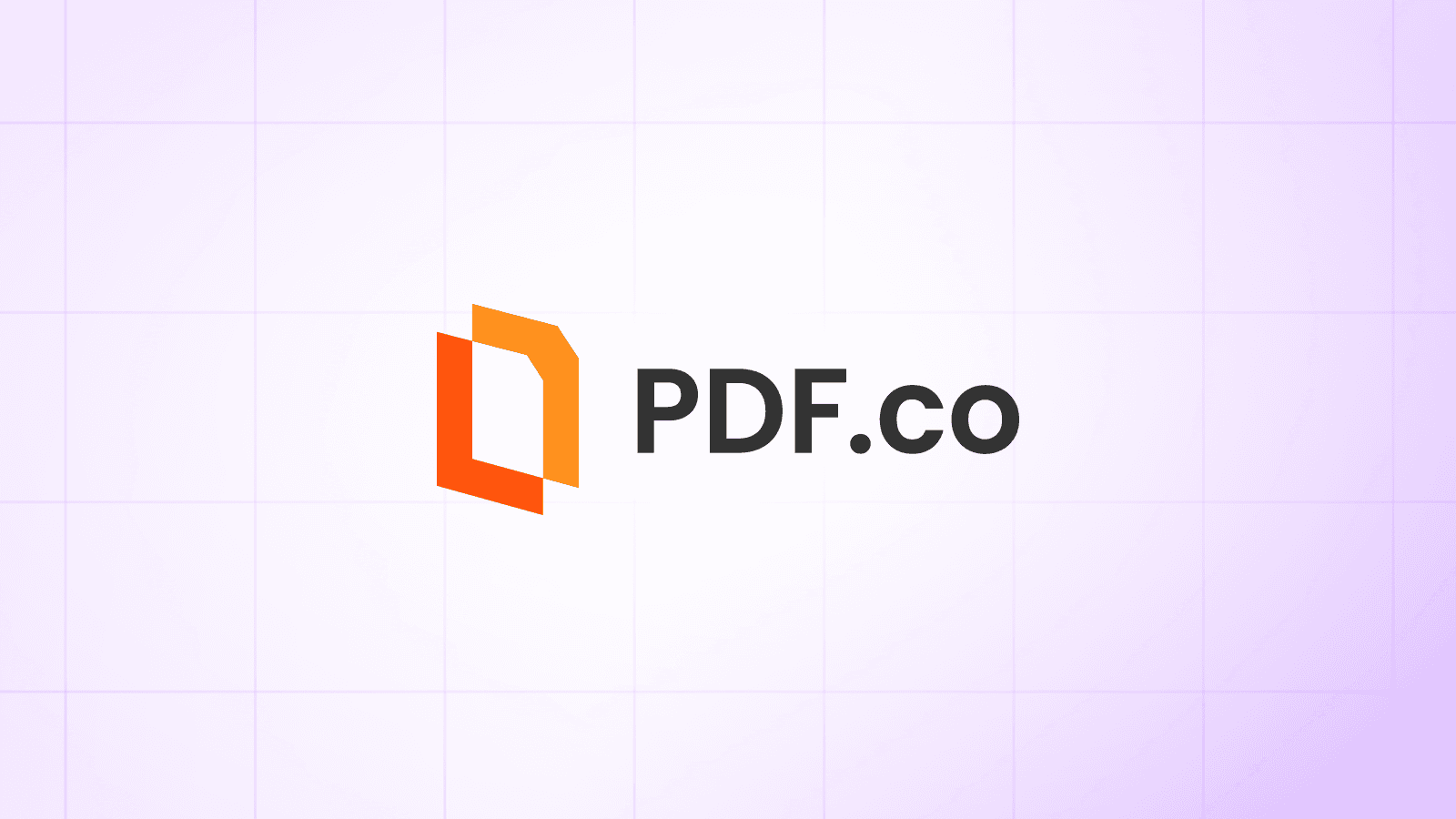
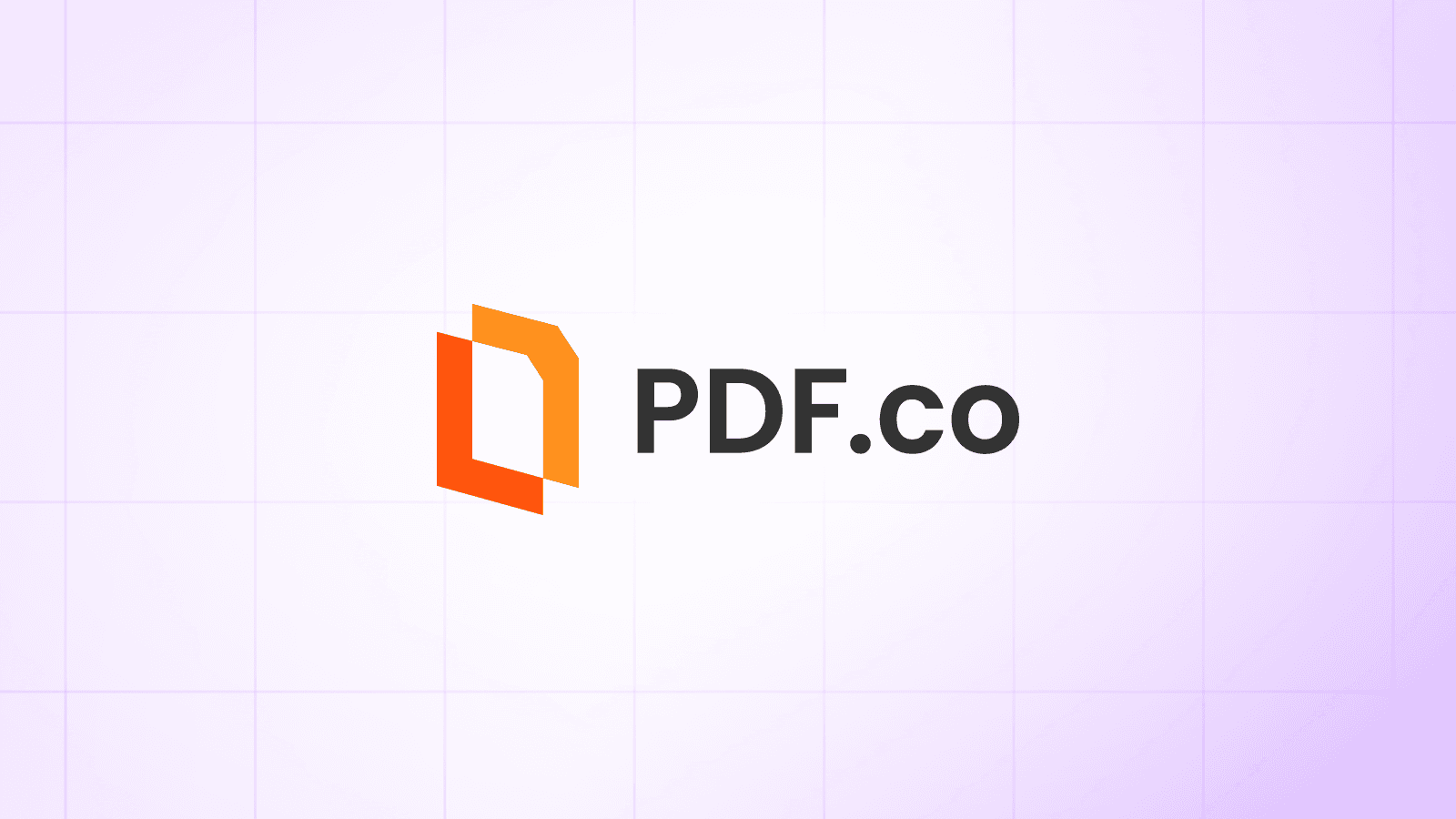
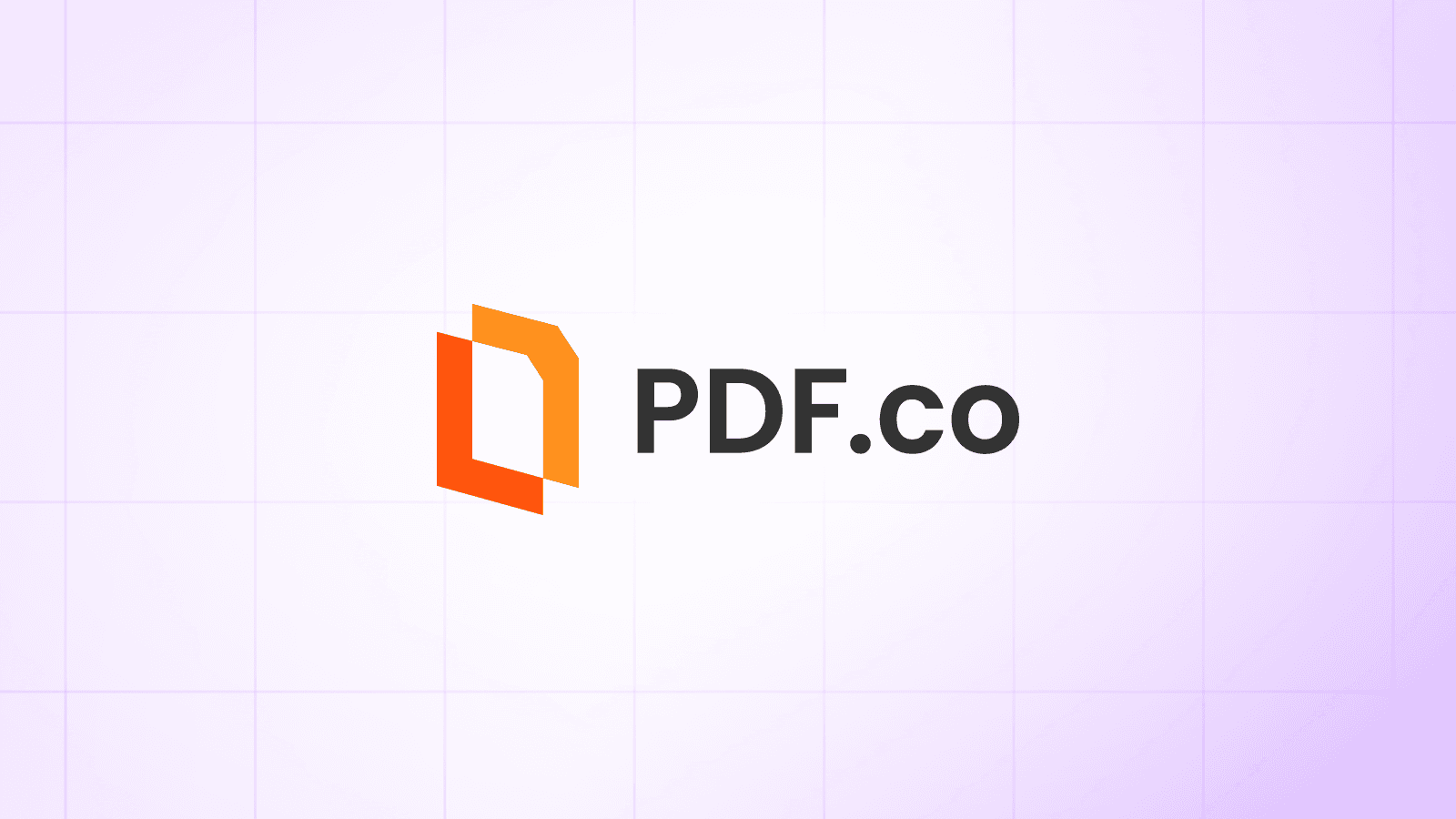