How to Add Password/Protection to PDF File in Salesforce Apex using PDF.co
In this step-by-step guide, we’ll briefly see steps on how to add passwords or protection to PDF files in Salesforce Apex using PDF.co. In the beginning, we’ll go through all the basic steps of Salesforce integration. Later we’ll also see the code and demo video. Let’s get started!
Step 1: Create Remote Site Settings
Create three remote site settings in the Salesforce Org like below:
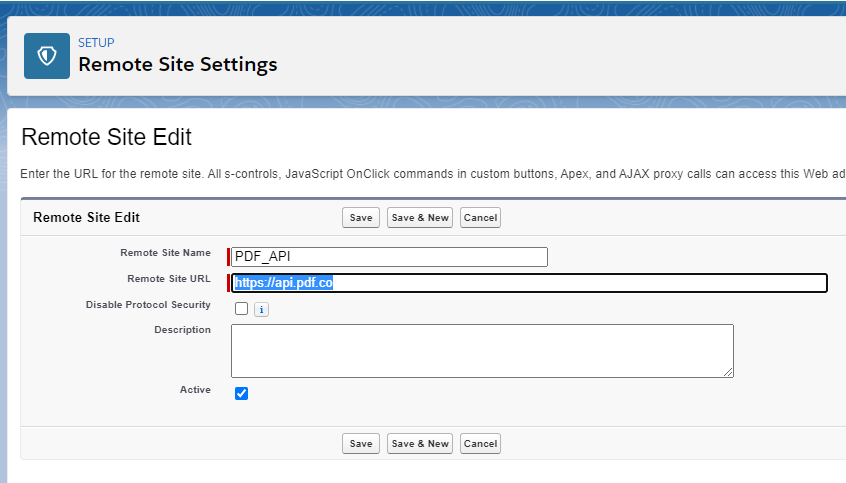
Please note that the URL is “https://api.pdf.co”.
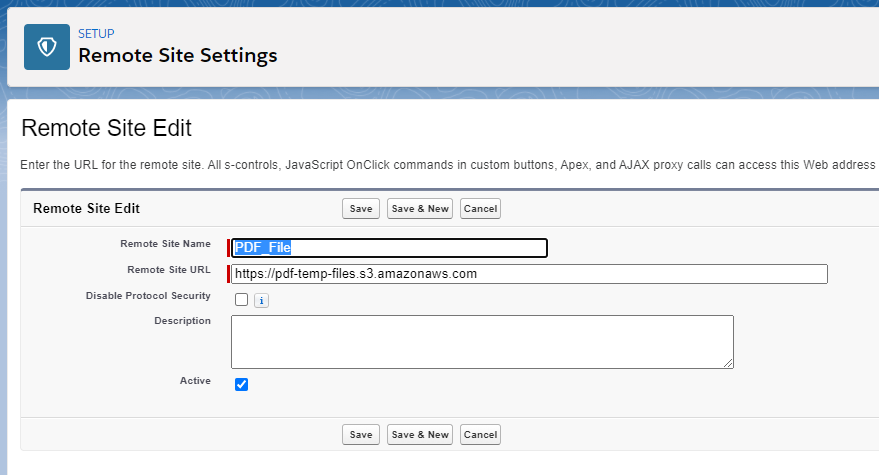
Please note that the URL is “https://pdf-temp-files.s3.amazonaws.com”.
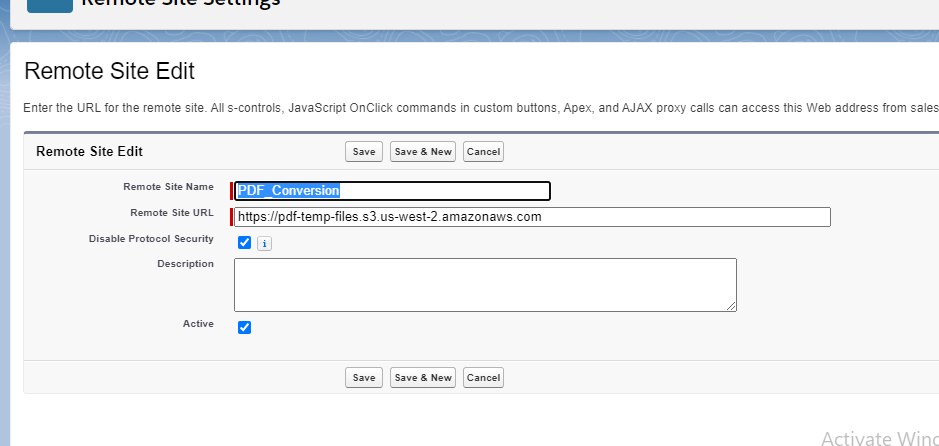
Please note that the URL is “https://pdf-temp-files.s3.us-west-2.amazonaws.com”.
Step 2: Create Apex Class in Salesforce
Create an apex class in Salesforce like below and paste the code there.
Once you login to the Salesforce org, you will see the screen like below and click on “Developer Console”
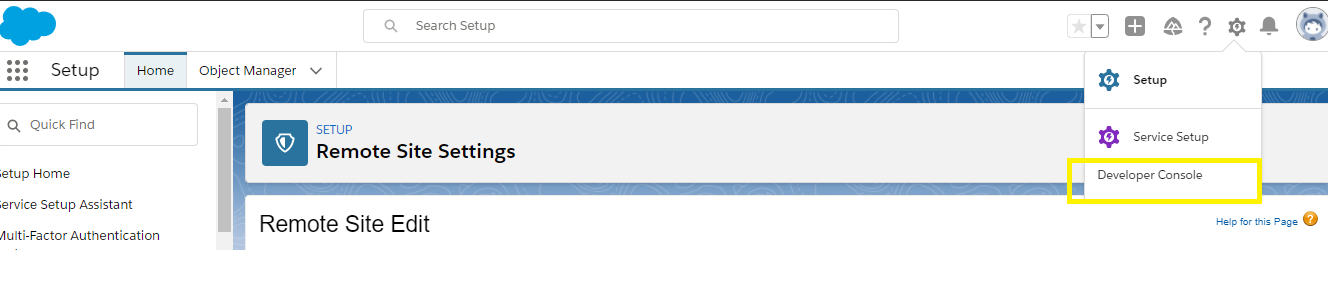
Create an apex class. For this, click on “Files” then “New” then “Apex Class”.
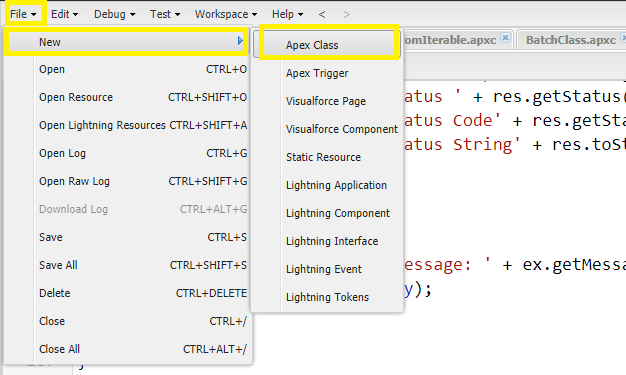
Write the class name “ProtectPDFDocument” and click “Ok”. Now copy the ProtectPDFDocument code in this file.
Similarly, create a new file with the name “ProtectPDFDocumentTest” and copy the code.
Step 3: Add the Key in the File
In the ProtectPDFDocument file, please add the key by replacing the ‘******************************************’;.
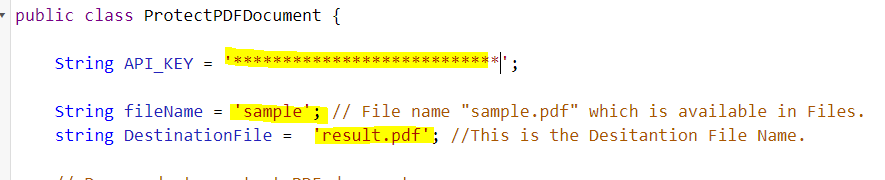
Then please add the fileName which we imported in Salesforce and the Destination file name which you want as a pdf.
Step 4: Verify the Code
To Verify the code, please open the execute Anonymous window and call the method like below:
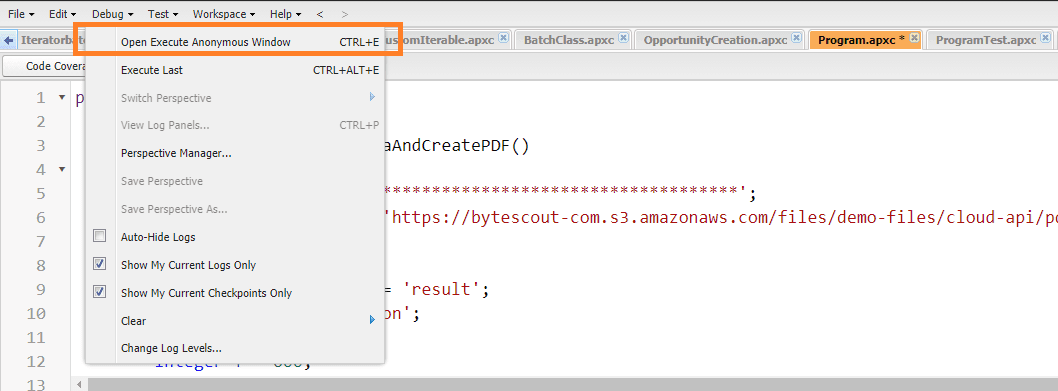
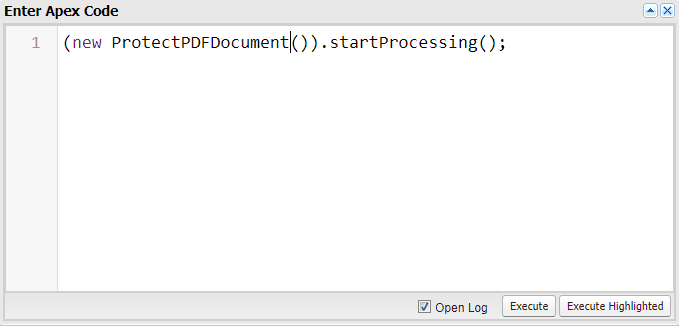
Then Click on “Execute”.
Step 5: Search Files
5. Now, Search Files from the “App Launcher”
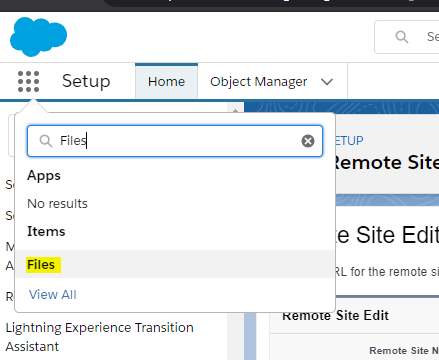
You will see your protected pdf file there.
Step 6: Source Code Files:
ProtectPDFDocument.cls
public class ProtectPDFDocument {
String API_KEY = '***************************';
String fileName = 'sample'; // File name "sample.pdf" which is available in Files.
string DestinationFile = 'result.pdf'; //This is the Desitantion File Name.
// Passwords to protect PDF document
// The owner password will be required for document modification.
// The user password only allows to view and print the document.
string OwnerPassword = '123456';
string UserPassword = '654321';
// Encryption algorithm.
// Valid values: 'RC4_40bit', 'RC4_128bit', 'AES_128bit', 'AES_256bit'.
string EncryptionAlgorithm = 'AES_128bit';
public void startProcessing()
{
ContentVersion cv = [select Title, VersionData from ContentVersion where Title = :fileName limit 1];
Blob SourceFile = cv.VersionData;
try
{
//1. Prepare URL for "Get Presigned URL" API call
string url = 'https://api.pdf.co/v1/file/upload/get-presigned-url?contenttype=application/octet-stream&name=:fileName';
HttpRequest req = new HttpRequest();
req.setHeader('x-api-key', API_KEY);
req.setEndpoint(url);
req.setMethod('GET');
req.setTimeout(60000);
Http http = new Http();
HTTPResponse res = http.send(req);
if(res.getStatusCode() == 200)
{
System.Debug('res ' + res);
Map<String, Object> deserializedBody = (Map<String, Object>)JSON.deserializeUntyped(res.getBody());
Boolean isError = Boolean.ValueOf(deserializedBody.get('error'));
if(isError == false)
{
// Get URL to use for the file upload
String uploadUrl = String.ValueOf(deserializedBody.get('presignedUrl'));
// Get URL of uploaded file to use with later API calls
String uploadedFileUrl = String.ValueOf(deserializedBody.get('url'));
// 2. UPLOAD THE FILE TO CLOUD.
if(uploadFile(API_KEY, uploadUrl, SourceFile))
{
// 3. Add Password to Uploaded file and download.
AddProtectionToInputPDF(API_KEY, DestinationFile, uploadedFileUrl, OwnerPassword, UserPassword, EncryptionAlgorithm);
}
}
}
else
{
System.debug('Error Response ' + res.getBody());
System.Debug(' Status ' + res.getStatus());
System.Debug(' Status Code' + res.getStatusCode());
System.Debug(' Response String' + res.toString());
}
}
catch(Exception ex)
{
String errorBody = 'Message: ' + ex.getMessage() + ' -- Cause: ' + ex.getCause() + ' -- Stacktrace: ' + ex.getStackTraceString();
System.Debug(errorBody);
}
}
@TestVisible
public static boolean uploadFile(String API_KEY, String url, Blob sourceFile)
{
HttpRequest req = new HttpRequest();
req.setHeader('x-api-key', API_KEY);
req.setHeader('Content-Type', 'application/octet-stream');
req.setEndpoint(url);
req.setMethod('PUT');
req.setTimeout(60000);
req.setBodyAsBlob(sourceFile);
Http http = new Http();
HTTPResponse res = http.send(req);
if(res.getStatusCode() == 200)
{
System.Debug(res);
return true;
}
else
{
System.debug('Error Response ' + res.getBody());
System.Debug(' Status ' + res.getStatus());
System.Debug(' Status Code' + res.getStatusCode());
System.Debug(' Response String' + res.toString());
return false;
}
}
public static void AddProtectionToInputPDF(String API_KEY, String DestinationFile, String uploadedFileUrl, String ownerPassword, String userPassword, String encryptionAlgorithm)
{
Map<string, object> parameters = new Map<string, object>();
parameters.put('name', DestinationFile);
parameters.put('url', uploadedFileUrl);
parameters.put('ownerPassword', ownerPassword);
parameters.put('userPassword', userPassword);
parameters.put('encryptionAlgorithm', encryptionAlgorithm);
string jsonPayload = Json.serialize(parameters);
String url = 'https://api.pdf.co/v1/pdf/security/add';
HttpRequest req = new HttpRequest();
req.setHeader('x-api-key', API_KEY);
req.setHeader('Content-Type', 'application/json');
req.setEndpoint(url);
req.setMethod('POST');
req.setTimeout(60000);
req.setBody(jsonPayload);
Http http = new Http();
HTTPResponse res = http.send(req);
if(res.getStatusCode() == 200)
{
System.Debug(res);
Map<String, Object> deserializedBody = (Map<String, Object>)JSON.deserializeUntyped(res.getBody());
Boolean isError = Boolean.ValueOf(deserializedBody.get('error'));
if(isError == false)
{
String resultFileUrl = String.ValueOf(deserializedBody.get('url'));
downloadPDFAndStore(resultFileUrl, DestinationFile);
}
}
else
{
System.debug('Error Response ' + res.getBody());
System.Debug(' Status ' + res.getStatus());
System.Debug(' Status Code' + res.getStatusCode());
System.Debug(' Response String' + res.toString());
}
}
@TestVisible
private static void downloadPDFAndStore(String extFileUrl, String DestinationFile)
{
Http h = new Http();
HttpRequest req = new HttpRequest();
extFileUrl = extFileUrl.replace(' ', '%20');
req.setEndpoint(extFileUrl);
req.setMethod('GET');
req.setHeader('Content-Type', 'application/pdf');
req.setCompressed(true);
req.setTimeout(60000);
//Now Send HTTP Request
HttpResponse res = h.send(req);
if(res.getStatusCode() == 200)
{
blob fileContent = res.getBodyAsBlob();
ContentVersion conVer = new ContentVersion();
conVer.ContentLocation = 'S'; // to use S specify this document is in Salesforce, to use E for external files
conVer.PathOnClient = DestinationFile + '.pdf'; // The files name, extension is very important here which will help the file in preview.
conVer.Title = DestinationFile; // Display name of the files
conVer.VersionData = fileContent;
insert conVer;
System.Debug('Success');
}
else
{
System.debug('Error Response ' + res.getBody());
System.Debug(' Status ' + res.getStatus());
System.Debug(' Status Code' + res.getStatusCode());
System.Debug(' Response String' + res.toString());
}
}
}
ProtectPDFDocumentTest.cls
@isTest
private class ProtectPDFDocumentTest
{
@TestSetup
static void makeData(){
ContentVersion conVer = new ContentVersion();
conVer.ContentLocation = 'S'; // to use S specify this document is in Salesforce, to use E for external files
conVer.PathOnClient = 'DestinationFile.pdf'; // The files name, extension is very important here which will help the file in preview.
conVer.Title = 'Sample'; // Display name of the files
conVer.VersionData = Blob.ValueOf('fileContent');
insert conVer;
}
private testmethod static void teststartProcessing()
{
Test.setMock(HttpCalloutMock.class, new ProtectPDFDocumentTest.ProtectPDFDocumentMock());
ProtectPDFDocument dc = new ProtectPDFDocument();
Test.startTest();
dc.startProcessing();
Test.stopTest();
List cv = [select Id from ContentVersion];
System.assertEquals(2, cv.size());
}
private testmethod static void testuploadFile()
{
Test.setMock(HttpCalloutMock.class, new ProtectPDFDocumentTest.ProtectPDFDocumentErrorMock());
Test.startTest();
Boolean result = ProtectPDFDocument.uploadFile('abc', 'https://www.google.com', Blob.ValueOf('fileContent'));
Test.stopTest();
System.assertEquals(false, result);
}
private testmethod static void teststartProcessingError()
{
ProtectPdfDocument dc = new ProtectPdfDocument();
Test.startTest();
dc.startProcessing();
Test.stopTest();
List cv = [select Id from ContentVersion];
System.assertEquals(1, cv.size());
}
public class ProtectPDFDocumentMock implements HttpCalloutMock {
public HTTPResponse respond(HTTPRequest req) {
HttpResponse res = new HttpResponse();
String testBody = '{"presignedUrl":"https://pdf-temp-files.s3-us-west-2.amazonaws.com/0c72bf56341142ba83c8f98b47f14d62/test.pdf?X-Amz-Expires=900&X-Amz-Algorithm=AWS4-HMAC-SHA256&X-Amz-Credential=AKIAIZJDPLX6D7EHVCKA/20200302/us-west-2/s3/aws4_request&X-Amz-Date=20200302T143951Z&X-Amz-SignedHeaders=host&X-Amz-Signature=8650913644b6425ba8d52b78634698e5fc8970157d971a96f0279a64f4ba87fc","url":"https://pdf-temp-files.s3-us-west-2.amazonaws.com/0c72bf56341142ba83c8f98b47f14d62/test.pdf?X-Amz-Expires=3600&x-amz-security-token=FwoGZXIvYXdzEGgaDA9KaTOXRjkCdCqSTCKBAW9tReCLk1fVTZBH9exl9VIbP8Gfp1pE9hg6et94IBpNamOaBJ6%2B9Vsa5zxfiddlgA%2BxQ4tpd9gprFAxMzjN7UtjU%2B2gf%2FKbUKc2lfV18D2wXKd1FEhC6kkGJVL5UaoFONG%2Fw2jXfLxe3nCfquMEDo12XzcqIQtNFWXjKPWBkQEvmii4tfTyBTIot4Na%2BAUqkLshH0R7HVKlEBV8btqa0ctBjwzwpWkoU%2BF%2BCtnm8Lm4Eg%3D%3D&X-Amz-Algorithm=AWS4-HMAC-SHA256&X-Amz-Credential=ASIA4NRRSZPHEGHTOA4W/20200302/us-west-2/s3/aws4_request&X-Amz-Date=20200302T143951Z&X-Amz-SignedHeaders=host;x-amz-security-token&X-Amz-Signature=243419ac4a9a315eebc2db72df0817de6a261a684482bbc897f0e7bb5d202bb9","error":false,"status":200,"name":"test.pdf","remainingCredits":98145}';
res.setHeader('Content-Type', 'application/json');
res.setBody(testBody);
res.setStatusCode(200);
return res;
}
}
public class ProtectPDFDocumentErrorMock implements HttpCalloutMock {
public HTTPResponse respond(HTTPRequest req) {
HttpResponse res = new HttpResponse();
String testBody = '{"presignedUrl":"https://pdf-temp-files.s3-us-west-2.amazonaws.com/0c72bf56341142ba83c8f98b47f14d62/test.pdf?X-Amz-Expires=900&X-Amz-Algorithm=AWS4-HMAC-SHA256&X-Amz-Credential=AKIAIZJDPLX6D7EHVCKA/20200302/us-west-2/s3/aws4_request&X-Amz-Date=20200302T143951Z&X-Amz-SignedHeaders=host&X-Amz-Signature=8650913644b6425ba8d52b78634698e5fc8970157d971a96f0279a64f4ba87fc","url":"https://pdf-temp-files.s3-us-west-2.amazonaws.com/0c72bf56341142ba83c8f98b47f14d62/test.pdf?X-Amz-Expires=3600&x-amz-security-token=FwoGZXIvYXdzEGgaDA9KaTOXRjkCdCqSTCKBAW9tReCLk1fVTZBH9exl9VIbP8Gfp1pE9hg6et94IBpNamOaBJ6%2B9Vsa5zxfiddlgA%2BxQ4tpd9gprFAxMzjN7UtjU%2B2gf%2FKbUKc2lfV18D2wXKd1FEhC6kkGJVL5UaoFONG%2Fw2jXfLxe3nCfquMEDo12XzcqIQtNFWXjKPWBkQEvmii4tfTyBTIot4Na%2BAUqkLshH0R7HVKlEBV8btqa0ctBjwzwpWkoU%2BF%2BCtnm8Lm4Eg%3D%3D&X-Amz-Algorithm=AWS4-HMAC-SHA256&X-Amz-Credential=ASIA4NRRSZPHEGHTOA4W/20200302/us-west-2/s3/aws4_request&X-Amz-Date=20200302T143951Z&X-Amz-SignedHeaders=host;x-amz-security-token&X-Amz-Signature=243419ac4a9a315eebc2db72df0817de6a261a684482bbc897f0e7bb5d202bb9","error":false,"status":200,"name":"test.pdf","remainingCredits":98145}';
res.setHeader('Content-Type', 'application/json');
res.setBody(testBody);
res.setStatusCode(201);
return res;
}
}
}
Why Protect Your PDF?
Protecting your PDF file can be important for several reasons, including:
Preventing unauthorized access
If your PDF file contains sensitive or confidential information, you may want to protect it to prevent unauthorized access by individuals who are not authorized to view or use the information.
Preventing copying or modification
If you want to retain control over the content of your PDF file, you may want to protect it to prevent others from copying or modifying it without your permission.
Preserving the integrity of the document
Protecting your PDF file can help ensure that the document remains intact and unaltered, which can be important if the document is legal or official in nature.
Protecting against malware
PDF files can be used to distribute malware or viruses, so protecting your PDF file can help prevent others from using it for malicious purposes.
By setting a password, restricting access to specific users or groups, and using encryption to protect the document’s contents, you can safely protect your PDF data.
Video Guide
Related Tutorials
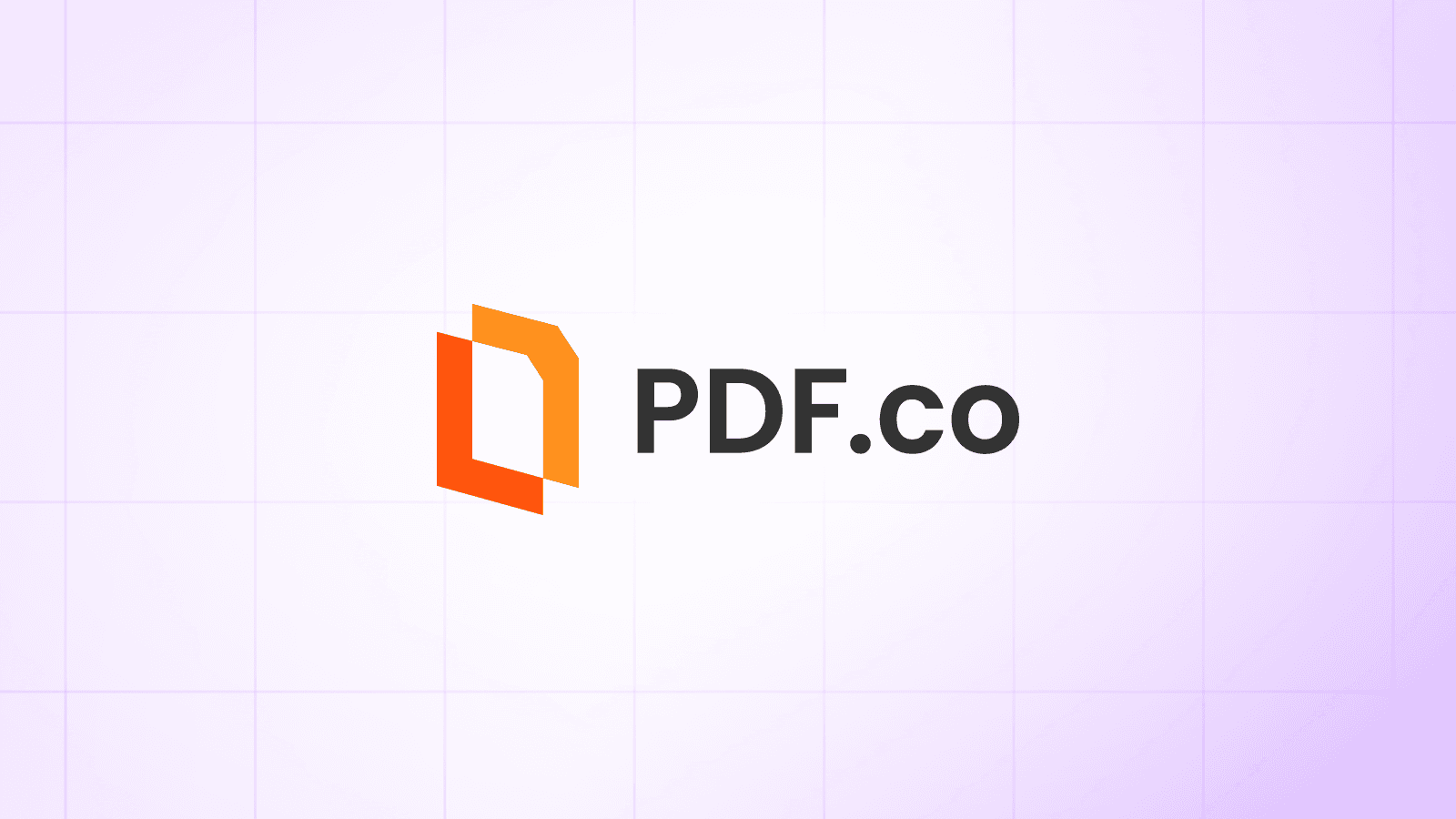
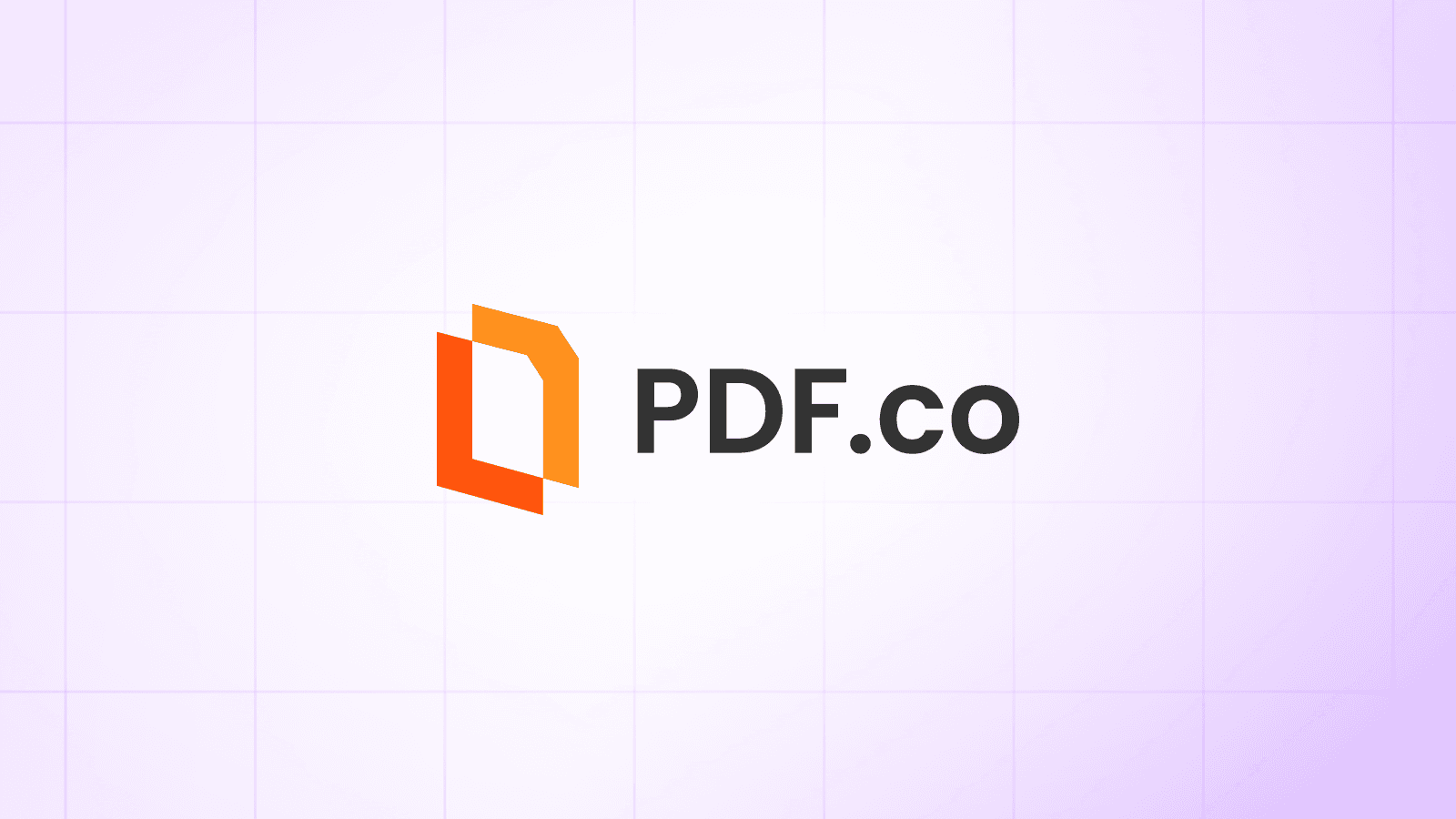