Webpage to PDF in JavaScript using PDF.co Web API
In this tutorial, we will demonstrate how to convert a webpage to PDF in JavaScript using the PDF.co Web API in asynchronous mode. Using asynchronous processing allows handling large files or long-running tasks without blocking other operations.
Benefits of Asynchronous Mode
- Avoids Timeouts: Large files or complex conversions may take time. Asynchronous mode prevents your application from being blocked or timing out during these processes.
- Optimized Workflow: Your application can continue executing other tasks while the server processes the PDF.
- Scalable: Asynchronous processing is ideal for applications handling multiple file conversions simultaneously.
Below is the image of Wikipedia’s main page and its PDF version after conversion.
Webpage Input:
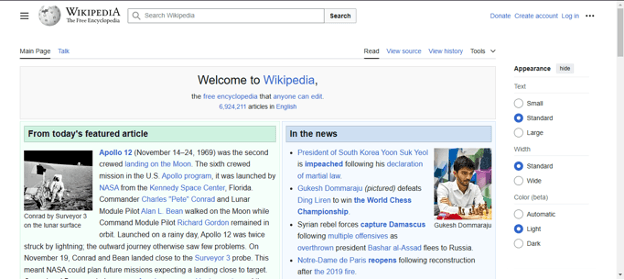
PDF Output:
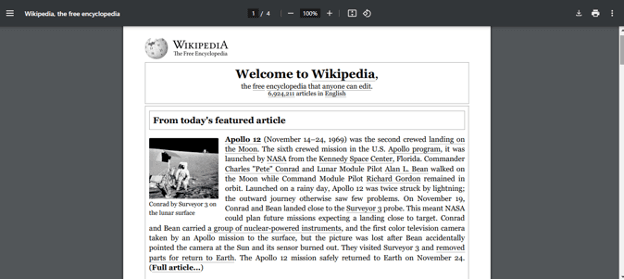
Step 1: Source Code
First, open the source code in your preferred editor and save it as index.js in a folder. You can get a copy of the source code here.
Step 2: Add API Key
Replace the placeholder in line 8 with your PDF.co API Key as the API_Key variable’s value. You can get your API key here.

Step 3: Configure Source and Destination
Specify the URL of the webpage to convert (e.g., Wikipedia’s main page: https://en.wikipedia.org/wiki/Main_Page
) and the name of the output file.
const sourceUrl = "https://en.wikipedia.org/wiki/Main_Page";

const outputFile = "webpage-output.pdf";

Step 4: Run Program
Run the program by typing the following command in the Terminal. Ensure the path is set to where the file is stored.
node index.js

How It Works
Initiating an Asynchronous Conversion:
- The code sends a request to the PDF.co API to start the conversion, with the async option set to
true
to enable asynchronous processing. - The API response contains a job ID and a URL to monitor the conversion status.
Checking Job Status:
- The code uses the job ID to periodically query the job's status by sending requests to the API.
- Once the status changes to "
success
", it indicates that the conversion process is complete, and the result file is ready for download.
Downloading the Result:
- After the conversion is successfully completed, the code retrieves the PDF file from the provided URL and saves it to the specified location on the local system.
In this tutorial, we demonstrated how to convert a webpage to PDF in JavaScript using the PDF.co Web API with asynchronous processing. This method ensures smoother handling of long-running tasks and frees up resources for other operations. For additional details, refer to the PDF.co Asynchronous Processing Documentation.
Related Tutorials
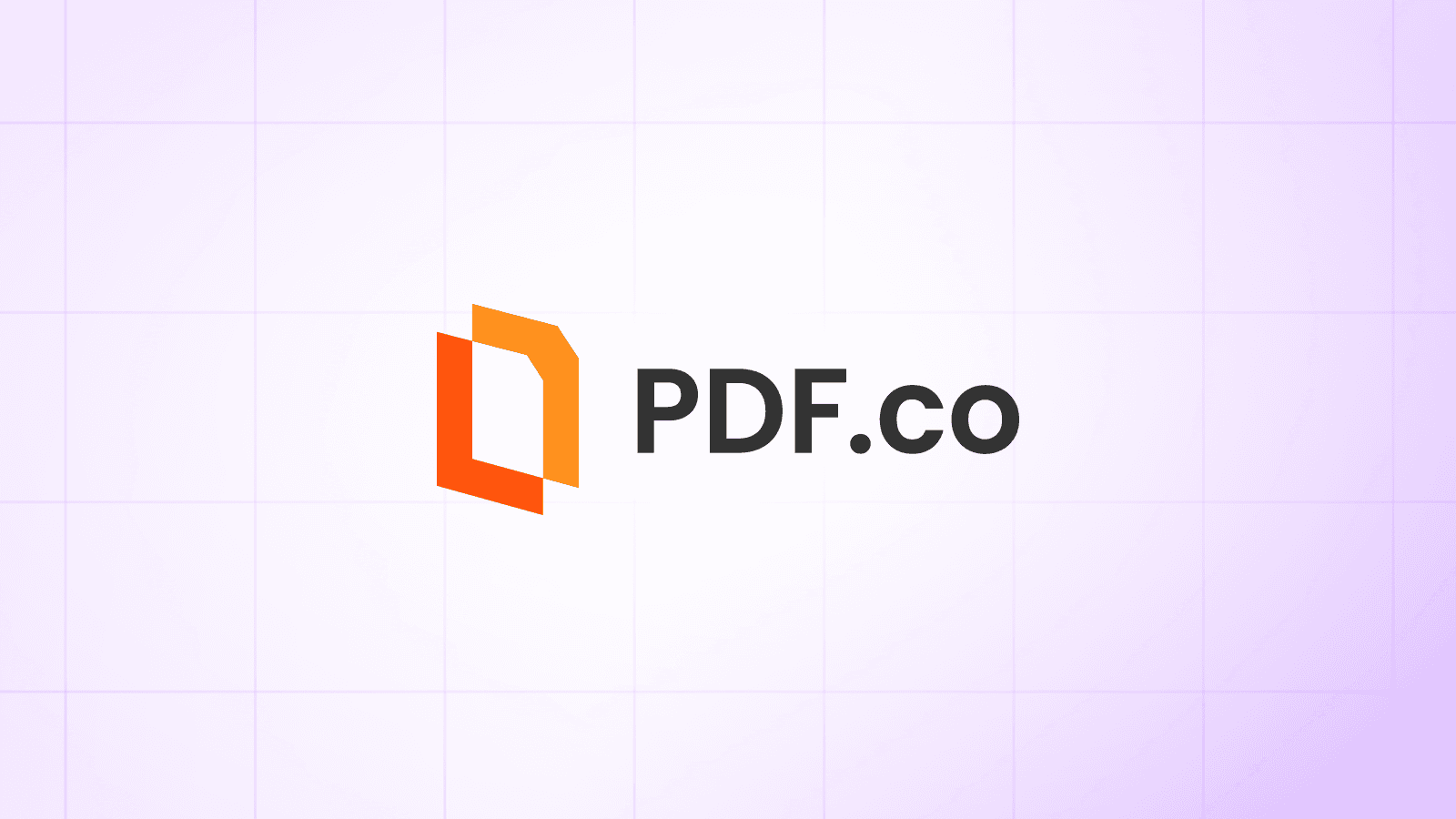
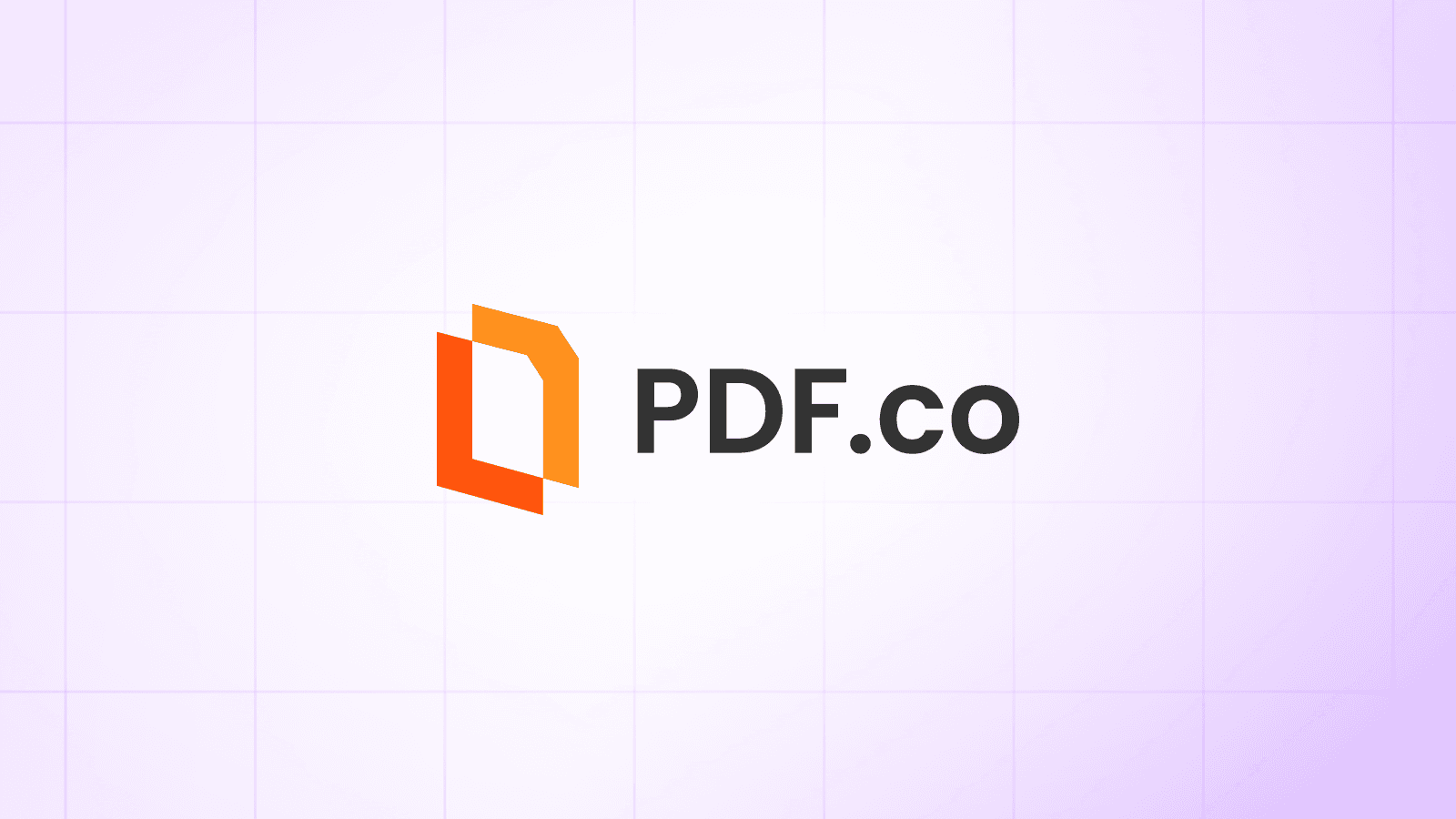
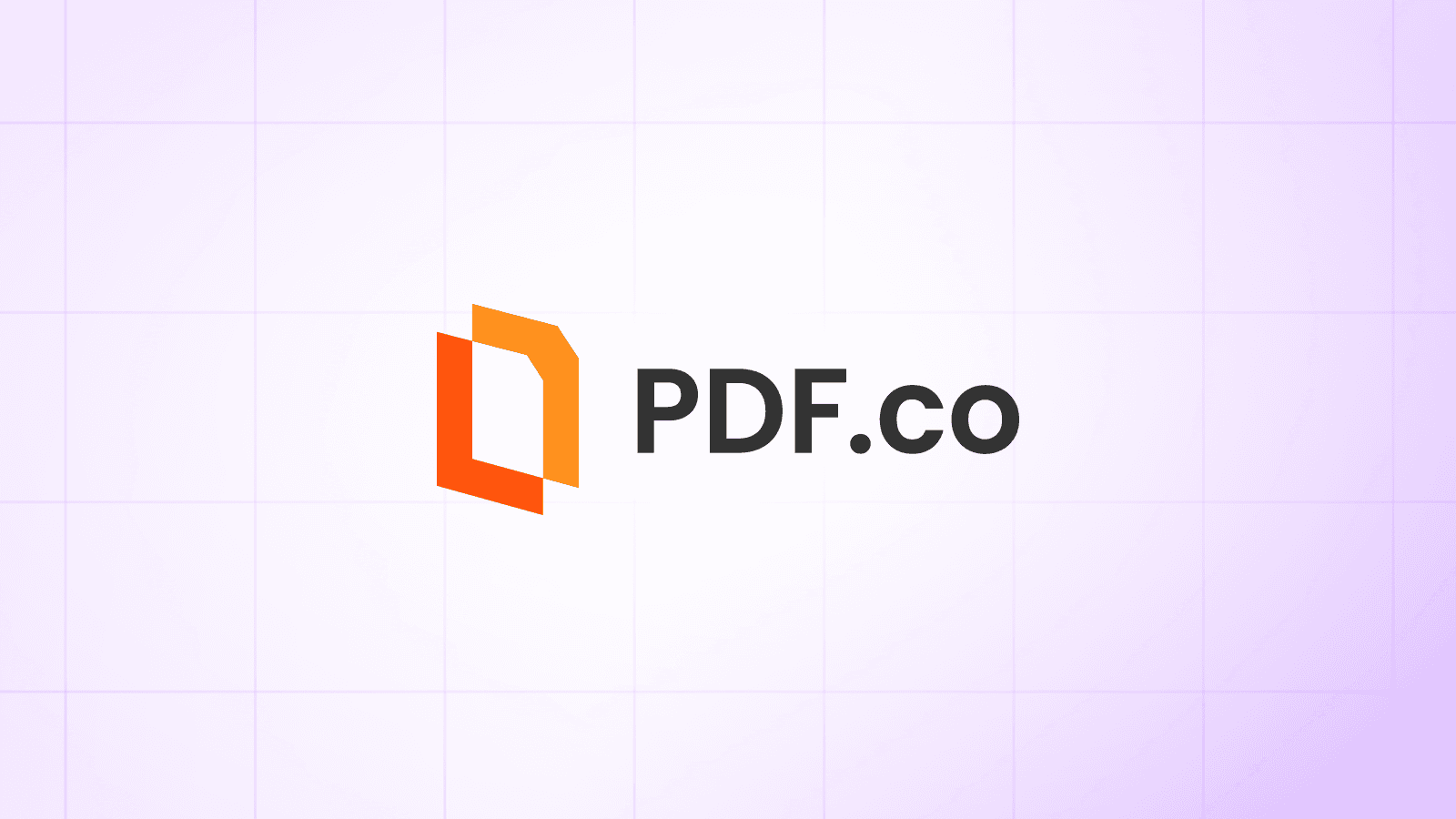
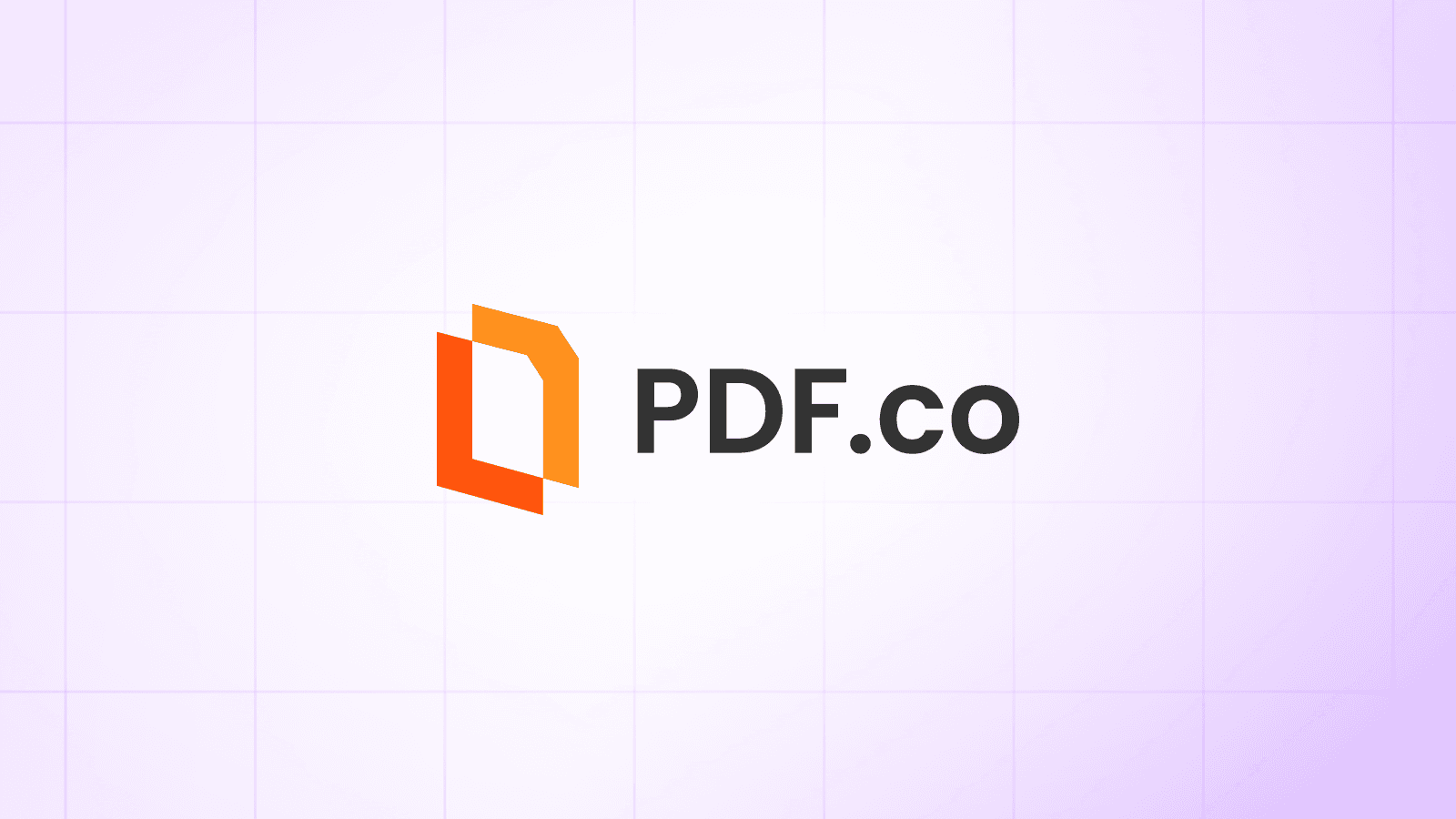