Securing PDF Files with PDF.co Web API in JavaScript
This tutorial shows you how to add or remove passwords and security restrictions from PDF files using asynchronous processing with the PDF.co Web API in JavaScript. Asynchronous processing allows tasks to run independently, so you can make your workflow more efficient by avoiding unnecessary waiting times.
Why Asynchronous Processing?
Asynchronous processing is crucial for handling tasks that take longer to complete, like processing large files, performing complex computations, or interacting with external APIs. Instead of pausing the entire program, it lets other tasks run simultaneously, enhancing performance and keeping your application responsive.
For more details on asynchronous processing, refer to the PDF.co Documentation.
Features of PDF Password and Security API Endpoint
The PDF.co Web API makes it easy to edit PDF documents across multiple programming languages, ensuring compatibility with various platforms and meeting your needs. The API allows you to modify PDFs by adding or removing passwords and security restrictions. Most importantly, it provides a secure system with encrypted connections for transmitting data files, ensuring your information stays protected. Additionally, the automated process saves you time and costs when editing documents.
Below are the details of the PDF Password and Security API, along with a demo that demonstrates these features using a simple example and sample code.
Endpoint Parameters
Here’s an easy-to-understand explanation of the parameters for the PDF Password and Security API. These parameters help you add or remove security features in PDF files:
url
: A required string containing the URL to the source file. Supported URLs include publicly accessible links (e.g., Dropbox, Google Drive, or PDF.co’s built-in storage).ownerPassword
: A required parameter for the primary password needed to add or remove security restrictions or encryption.userPassword
: An optional password required for viewing or printing the document.encryptionAlgorithm
: Specifies the encryption type for the process. Examples include RC4_40bit, RC4_128bit, and AES_128bit.allowAccessibilitySupport
: Enables or restricts content extraction for accessibility (true or false).allowAssemblyDocument
: Allows or prohibits assembling (reorganizing) the document (true or false).allowPrintDocument
: Enables or disables printing the document (true or false).allowFillForms
: Permits or blocks filling interactive forms, including signature fields (true or false).allowModifyDocument
: Allows or restricts modifying the document (true or false).allowContentExtraction
: Permits or prohibits copying content (true or false).allowModifyAnnotations
: Enables or blocks interaction with text annotations and forms (true or false).printQuality
: Defines the allowed printing quality, either HighResolution or LowResolution.encrypt
: Enables (true) or disables (false) encryption for the output file stored in the cloud.name
: Specifies a name for the output file.async
: Runs the process asynchronously if set totrue
. The response includes a job ID and a status URL to track progress. You can poll the status URL or set up a webhook for notifications when the task completes.expiration
: (Optional) Sets how long the output file remains available before deletion.profiles
: (Optional) Configures additional custom settings for the API operation.
Example using JavaScript
Here are sample source codes that demonstrate how to add or remove passwords from PDF documents using the PDF.co Password and Security API. These examples, written in JavaScript, show how to use key API parameters to perform these tasks. The implementation uses asynchronous processing with Axios, making the handling of requests and responses smooth and efficient.
To use this API, you need to provide the URL of the document you want to modify. Additionally, you must include your personalized API key, which is generated for you when you log in to the PDF.co platform.
Once you run the program, the API processes the document and provides a link to the modified PDF file. You can use this link to access the updated document and review the changes made to its password or security settings.
Sample Code Snippet for Password Addition
The following source code contains the program to add the password to an existing PDF document:
Code Sample: Here
Sample Code Walkthrough
The following step-by-step guide explains the source code to add the password to a document:
- The require parameter includes all the essential modules in the application. It loads critical files such as the Node.js, community, and local modules. These modules enable HTTP requests, file system management, and path handling, facilitating seamless communication with external APIs, processing of file paths, and saving the results to the local filesystem.
- The next thing is to provide the request method, the URL, and an API key to access the API functionalities. You have to provide the API key in the header for authentication. After logging in to the PDF.co platform, you can obtain your specific API key.
- The next step is to provide relevant information for alterations. You have to provide information in the payload, such as the URL of the document, ownerPassword, userPassword, EncryptionAlgorithm, and the name of the output file.
- Add the async:true parameter to the payload. This allows the process to run in asynchronous mode.
- Send the request and handle the response. In asynchronous mode, the response includes a jobId and a status URL. You can poll the status URL or set up a webhook to monitor progress and retrieve the final output.
- Once the status changes to "success", it indicates that the conversion process is complete, and the result file is ready for download.
- After the process is successfully completed, the code retrieves the PDF file from the provided URL and saves it to the specified location on the local system.
Output
Sample PDF Document
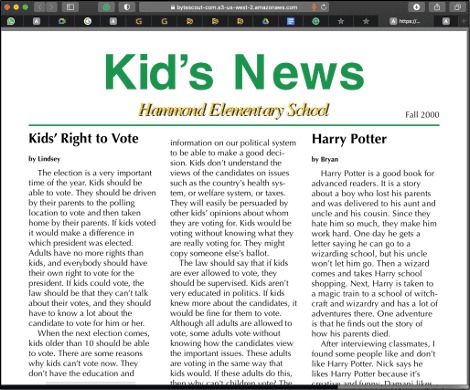
Secure PDF Output with Password
Below is the screenshot of the edited document after adding the password using the PDF Password And Security API.
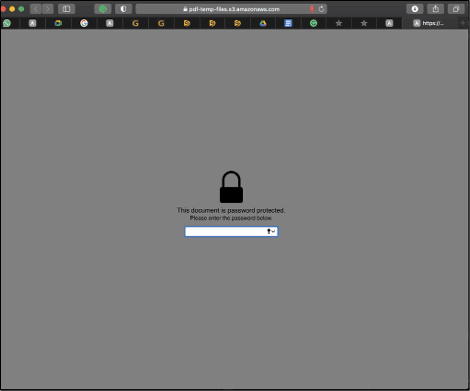
Sample Code Snippet for Password Removal
The following source code contains the program to remove the password from an existing PDF document:
Code Sample: Here
Sample Code Walkthrough
Below is the step-by-step guide for an explanation of the source code to remove the password from a document:
- The first thing is to add the required modules.
- The next thing is to provide the request method, the URL, and an API key to access the API functionalities.
- The next step is to provide relevant information for alterations. You have to provide information in the payload, such as the document URL, password, and output file name.
- Add the async: true parameter to the payload. This allows the process to run in asynchronous mode.
- Send the request and handle the response. In asynchronous mode, the response includes a jobId and a status URL. Users can poll the status URL or set up a webhook to monitor progress and retrieve the final output.
- Send the request and handle the response. In asynchronous mode, the response includes a jobId and a status URL. You can poll the status URL or set up a webhook to monitor progress and retrieve the final output.
- Once the status changes to "success", it indicates that the conversion process is complete, and the result file is ready for download.
- After the process is successfully completed, the code retrieves the PDF file from the provided URL and saves it to the specified location on the local system.
Related Tutorials
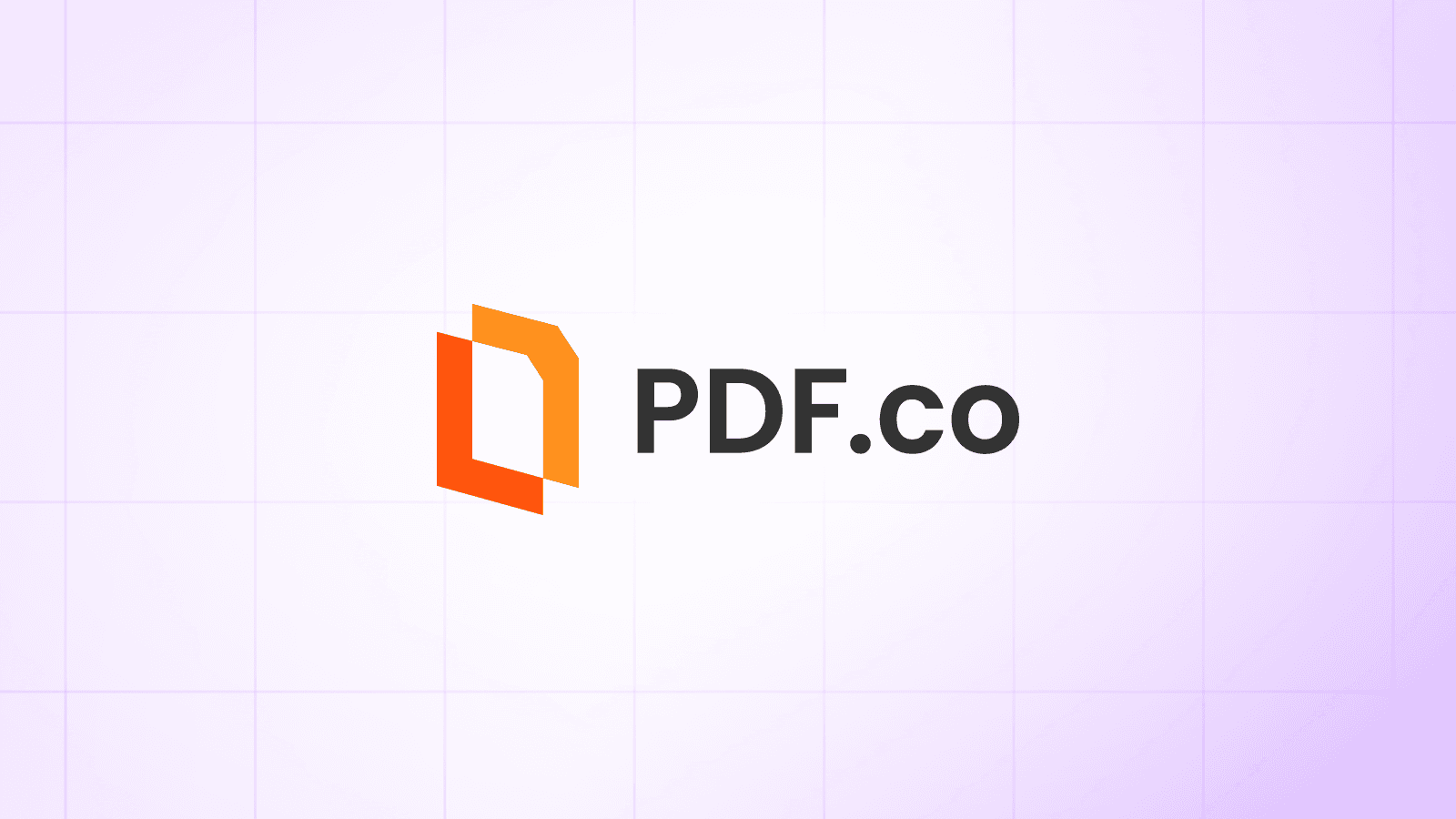
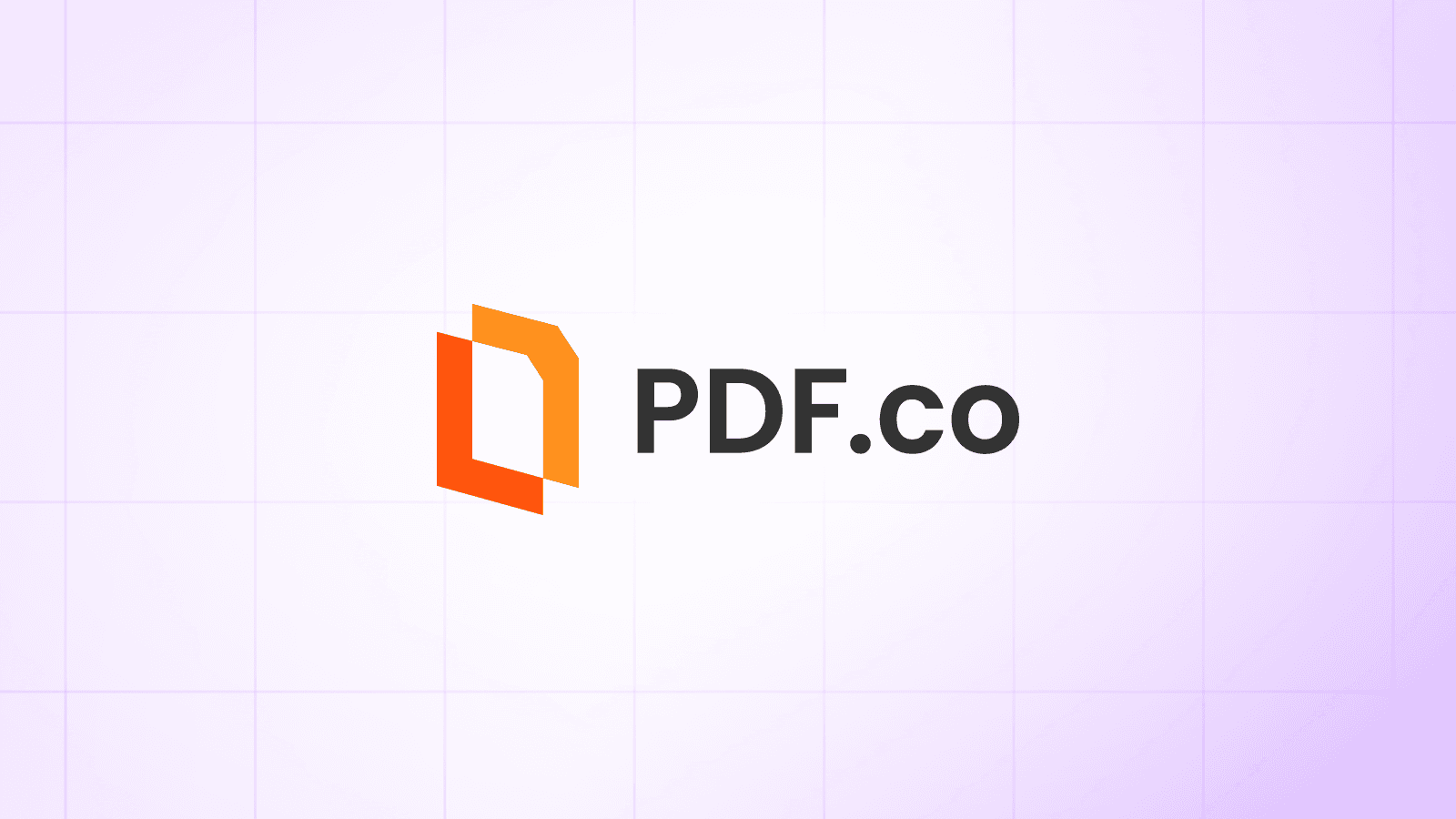
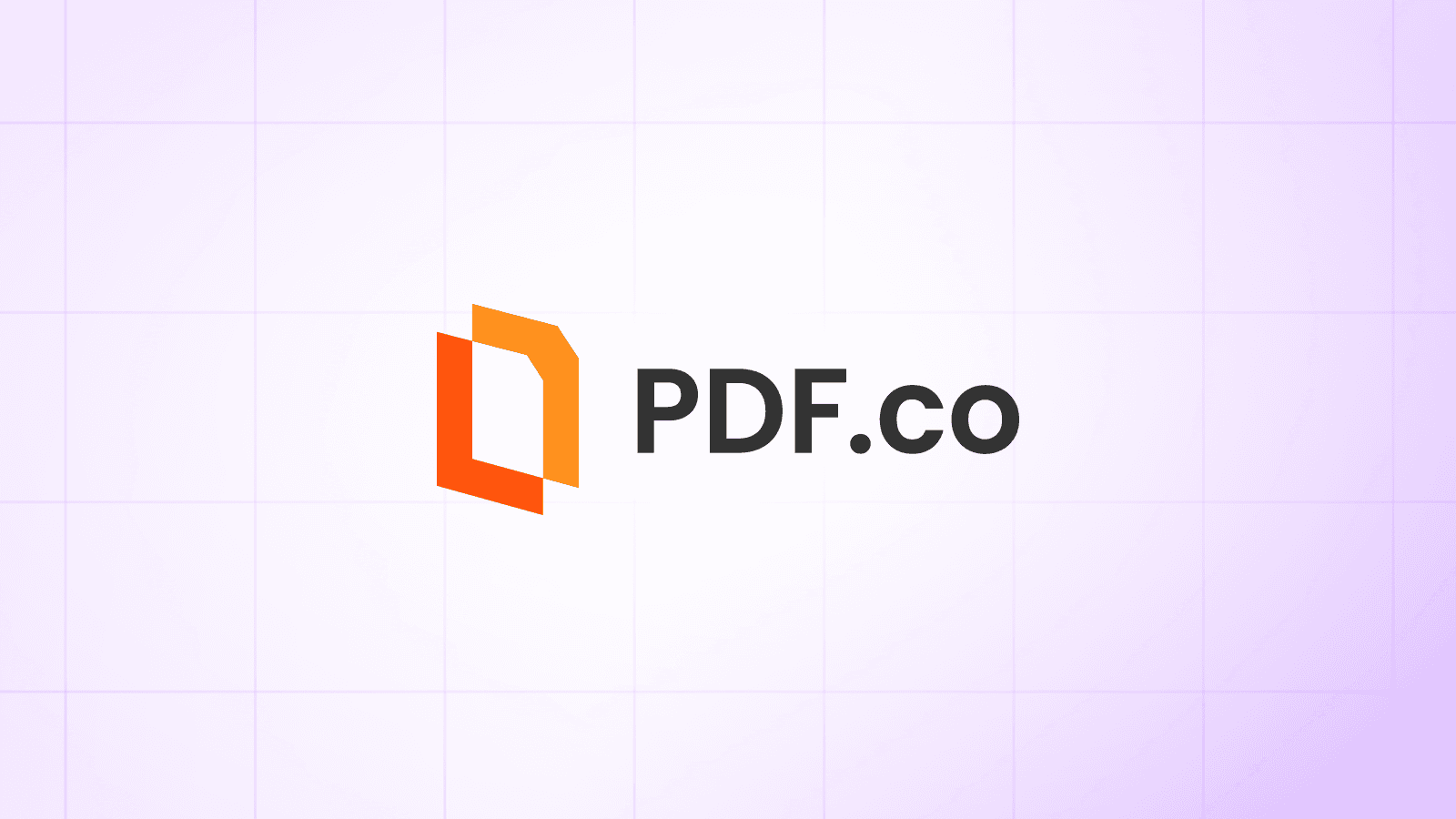
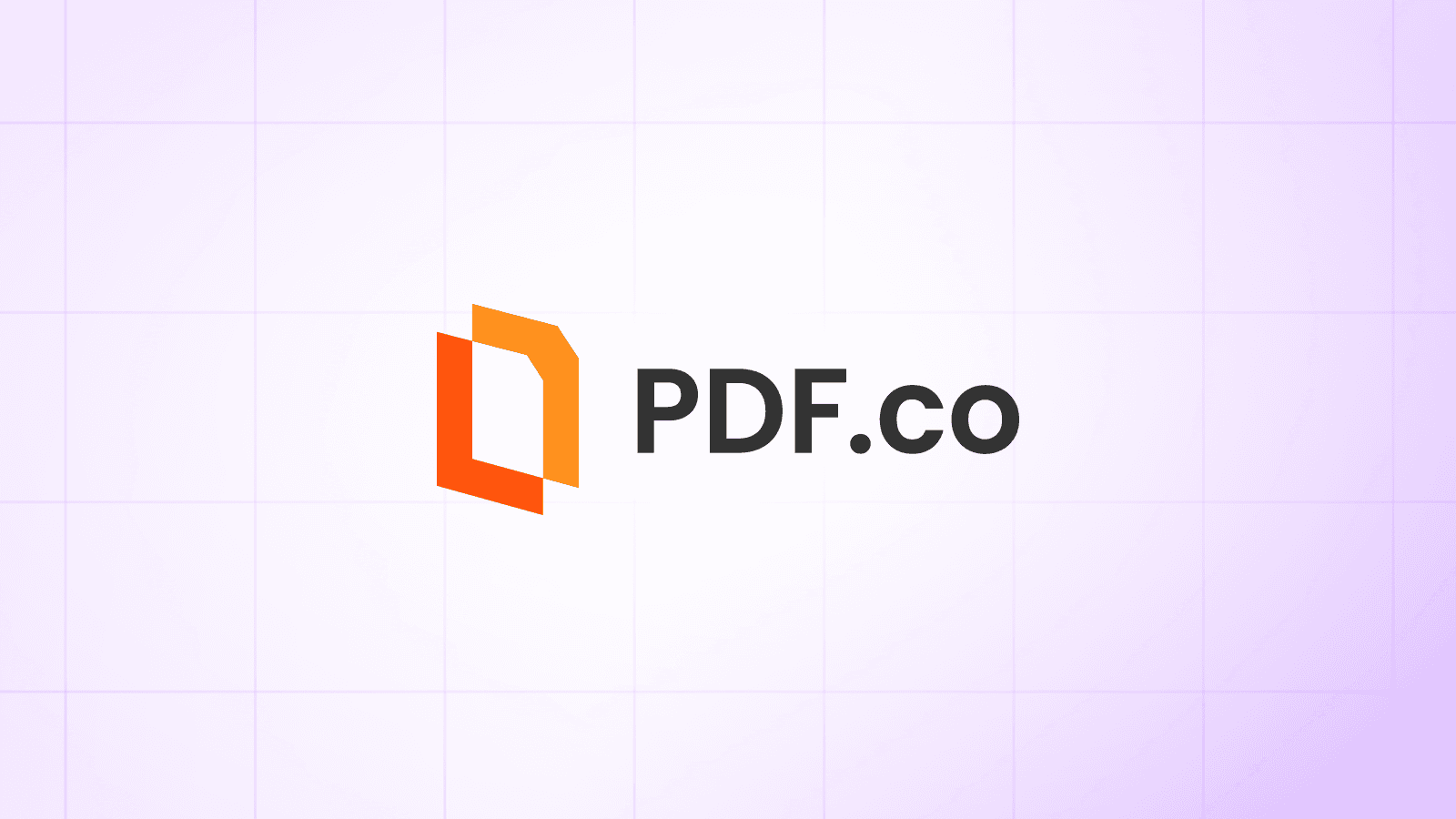