If you get errors like “too many requests” or “access denied,” try adding the parameter cache to enable built-in caching.
How to Optimize PDF using PDF.co Web API in JavaScript
This tutorial demonstrates how to optimize PDF files using the PDF.co Web API, focusing on the benefits of asynchronous processing. Asynchronous processing allows users to initiate tasks without waiting for completion, enabling better resource utilization and responsiveness in applications.
For more details on asynchronous processing, refer to the PDF.co Documentation.
Why Choose Asynchronous Processing?
Asynchronous processing is ideal for handling tasks that may take time to complete, such as processing large files or multiple requests simultaneously. It enables:
- Improved Efficiency: The application can handle other tasks while waiting for a response.
- Scalability: Supports multiple concurrent operations, enhancing overall system performance.
Enhanced User Experience: Reduces application downtime, making it more responsive to user actions.
Features of PDF Optimize Endpoint
You can use the PDF.co web API’s URL endpoint to reduce the size of a PDF file. Just provide the URL of the PDF along with optional settings, and the endpoint will generate a link to the compressed PDF file. You can also download the file using file management tools like “fs” to save it to your local storage.
Endpoint Parameters
Following are the parameters of the PDF Optimize endpoint:
url
(Required): This is the link to the PDF file you want to optimize. The URL can come from Dropbox, Google Drive, or PDF.co’s file storage. If you’re uploading your file via the API, check the file upload section.
httpusername
(Optional): Use this if the source URL requires an HTTP username for authentication.httppassword
(Optional): Use this if the source URL requires an HTTP password for authentication.name
(Optional): This is the name of the output file. By default, it’s set to result.pdf.expiration
(Optional): Sets how long the output link will remain valid, in minutes. The default is 60 minutes. If you need permanent storage, use PDF.co’s built-in file storage.password
(Optional): If the source PDF is password-protected, provide the password here. You can also use PDF.co’s security endpoints to add or remove passwords from PDFs.async
(Optional): Set this totrue
to run the process asynchronously. You’ll receive a job ID and a status URL to track the progress. You can poll the status URL or use webhooks for notifications.encrypt
(Optional): Use true to encrypt the output PDF file. The default value is false.profiles
(Optional): A string for extra settings or custom configurations. Use this to fine-tune your optimization process.
Query Parameter
The query parameters are additional settings you can attach to API endpoint URLs to customize their behavior. However, this particular endpoint does not require any query parameters.
Endpoint Method
This endpoint only supports the API “POST” method.
Sample Code Using Javascript
Here’s an explanation of how the source code works to reduce the size of a PDF using the PDF.co Web API:
- Purpose: The code demonstrates how to optimize a PDF file using its URL. It reduces the file size while enabling asynchronous processing (async=true).
- Steps:
- Input PDF: Provide the URL of the PDF file (e.g., a sample invoice).
- Async Parameter: Set
async=true
to run the optimization process in the background, allowing your application to handle other tasks in the meantime. - Expiration: Use the
expiration
parameter to specify how long the resulting file should remain available for download.
- Output Handling: After the process is complete:
- Retrieve the task status.
- Get the optimized file’s size and URL.
- Use the URL to either view the optimized PDF or download it.
If needed, modules like fs
can help save the file to local storage.
Sample Code
Here is the sample code to optimize the PDF file: Code Sample Here
Output Document
Below is the screenshot of the output file obtained in the API response:
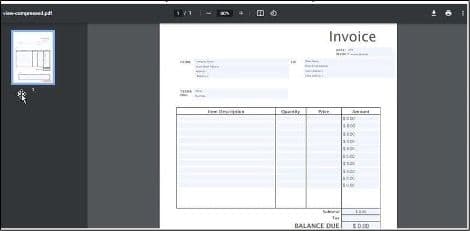
Step-by-Step Guide
- Install Required Modules: Use your package manager (e.g., npm) to install necessary modules like axios or node-fetch. Import them into your code to enable proper functionality.
- Set Up package.json: Configure the package.json file to run and test your JavaScript file seamlessly.
- Declare and Initialize the API Key: Create a variable named
API_KEY
and initialize it with your API key obtained from the PDF.co dashboard. You can also create a .env file to securely store the API key and access it in your code while keeping it hidden. - Set the Source File URL: Declare a variable
fileUrl
and assign it the URL of the source PDF file. This allows you to optimize different files by simply updating the variable without modifying the code or API details. - Define API Options: Declare a variable
options
and initialize it with API options, including the HTTP method, endpoint URL, headers, and form data. The form data should include values for the endpoint parameters. - Enable Asynchronous Processing: Add the
async: true
parameter to the payload, allowing the task to run in the background while your application performs other operations. - Send the Request: Use your chosen HTTP library (e.g., axios or node-fetch) to send the API request. In asynchronous mode, the response will include a
jobId
and astatus URL
. - Monitor Task Progress: Use the
status URL
to poll for updates or set up a webhook to track the operation’s progress. Once the process is complete, you’ll receive the final output details. - Retrieve and Download the File: Use the resulting URL provided in the response to download the optimized file. You can use modules like fs to save the file locally.
File Sizes
Below is the screenshot to demonstrate the size difference of both files.

You can learn more about this API endpoint and explore various code samples here. The samples are available in multiple programming languages, showcasing how the API works with both uploaded files and file URLs, in synchronous and asynchronous modes.
Related Tutorials
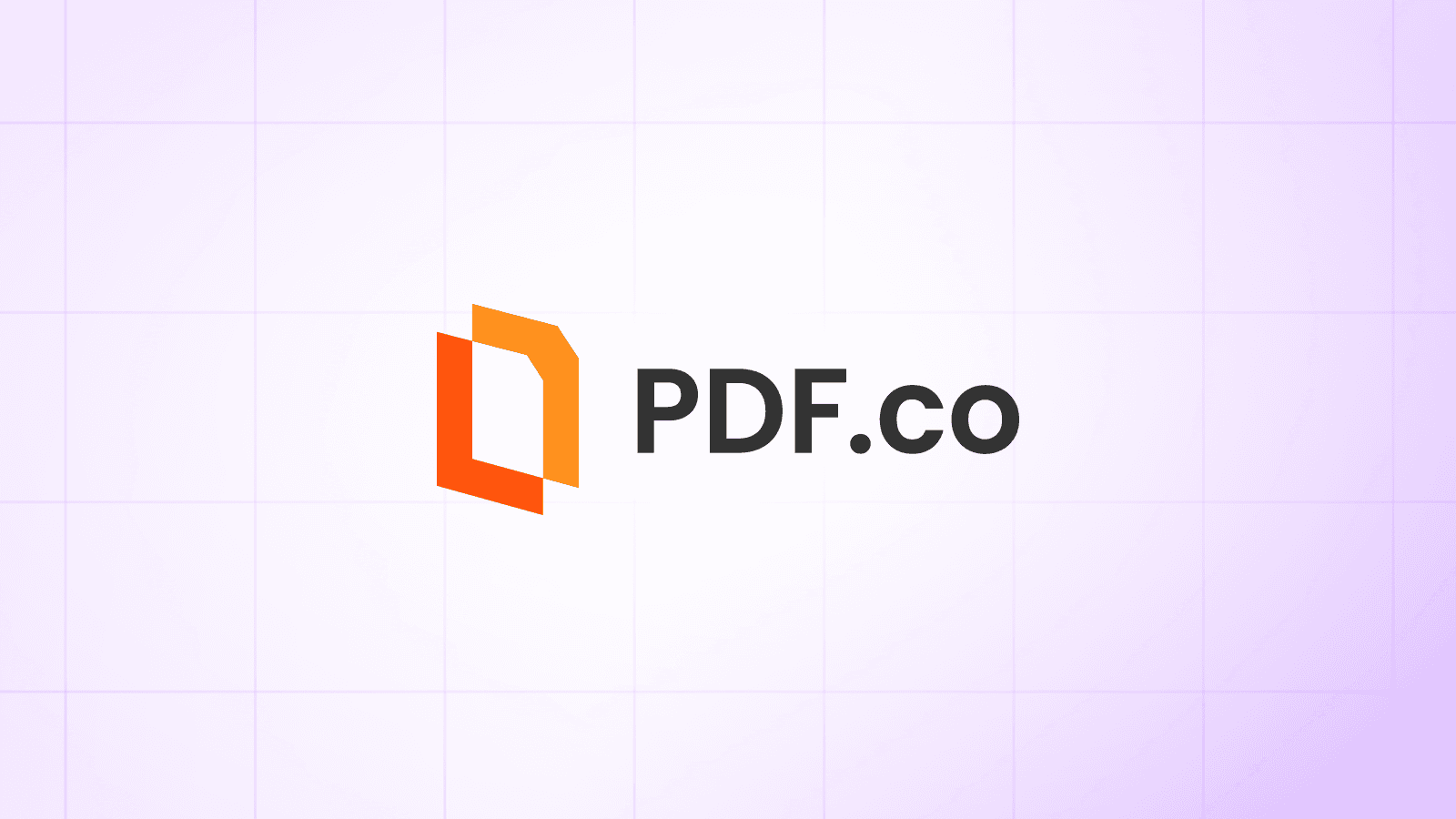
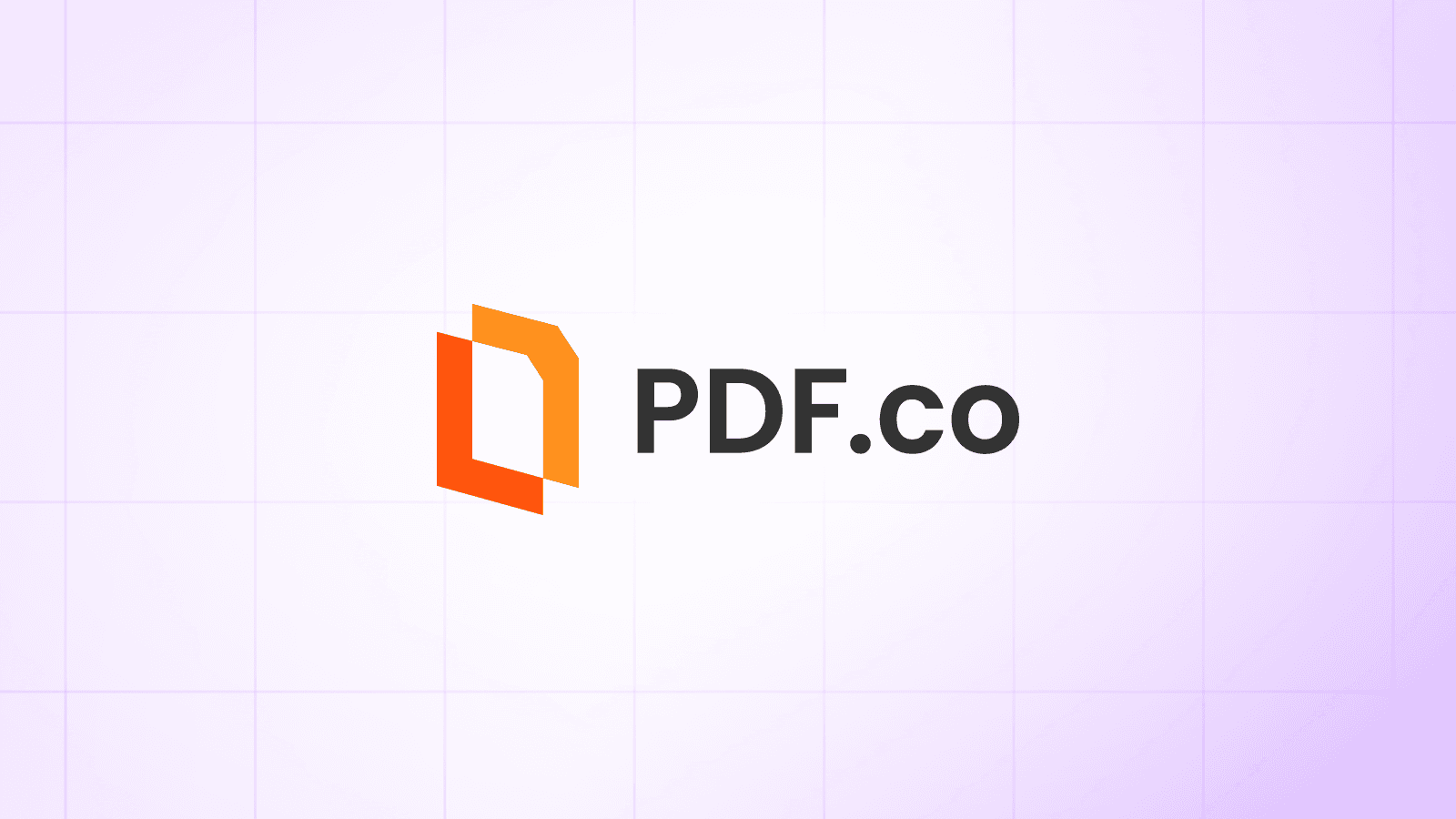
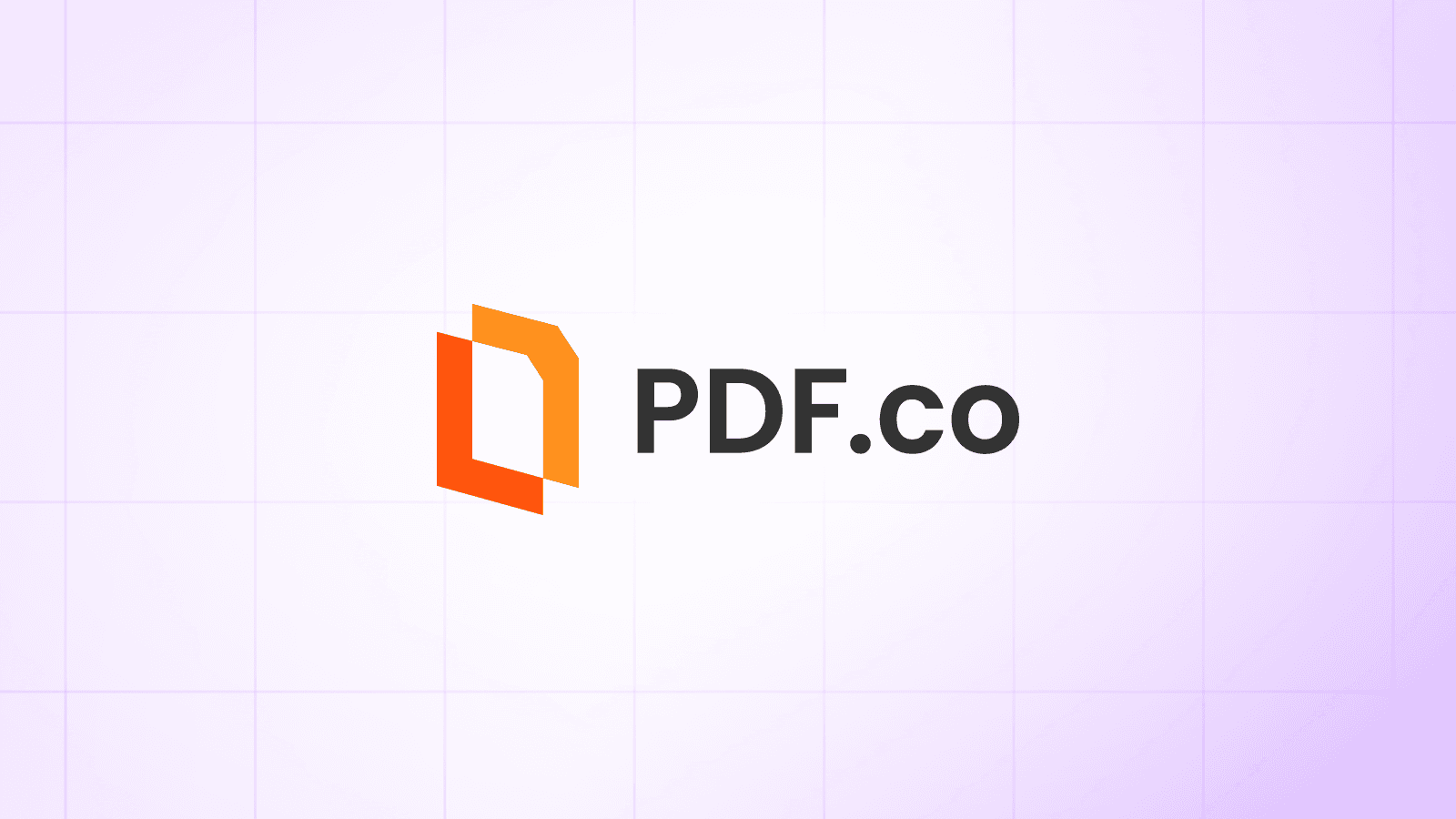
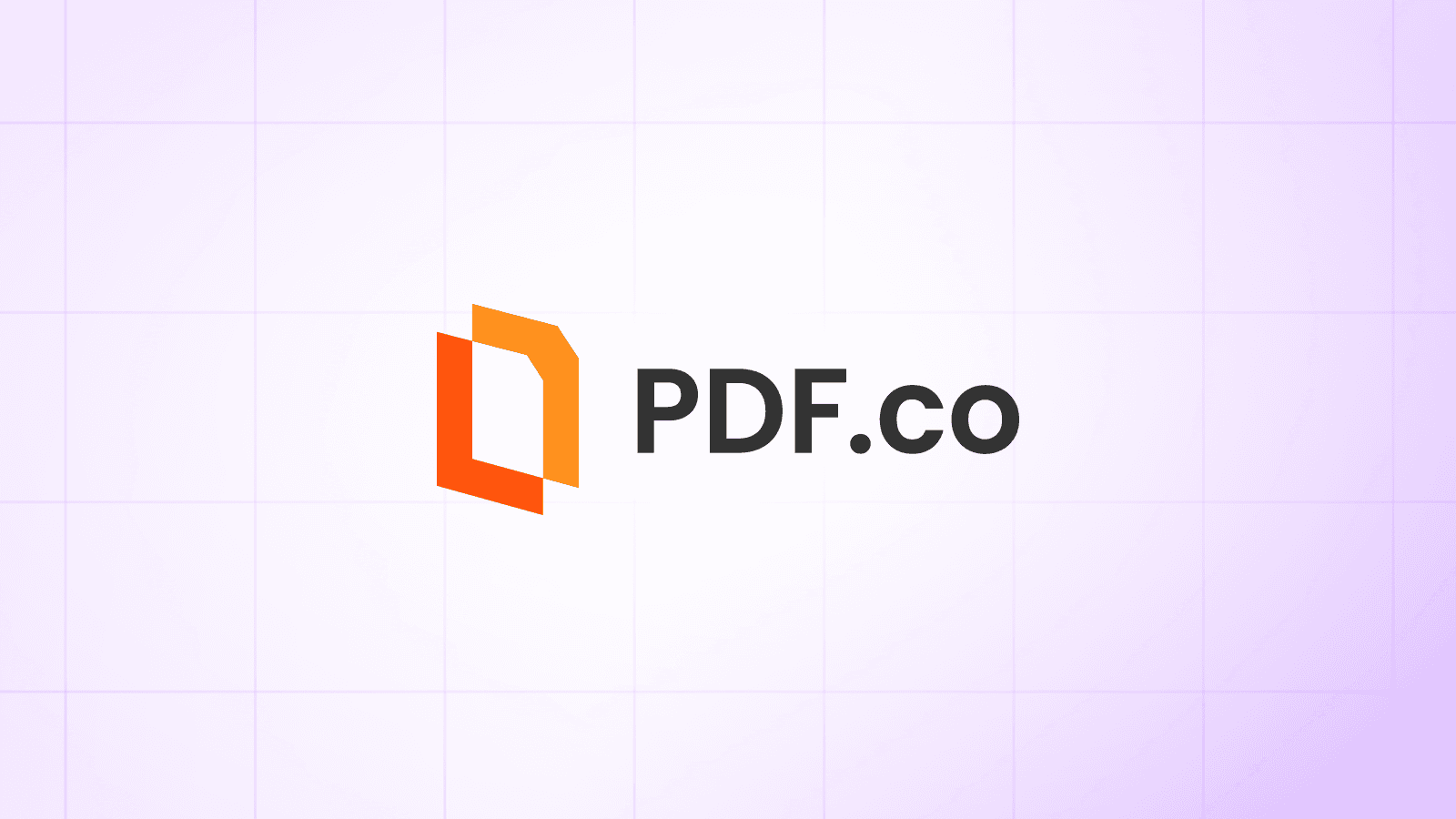