How to Convert PDF to CSV in Salesforce Apex using PDF.co
In this tutorial, we will show you how to convert a PDF document to CSV in Salesforce Apex using PDF.co
Step 1: Create Remote Site Settings
Create a Remote Site Settings in the Salesforce Org like below. Add the https://api.pdf.co
link as the Remote Site URL.
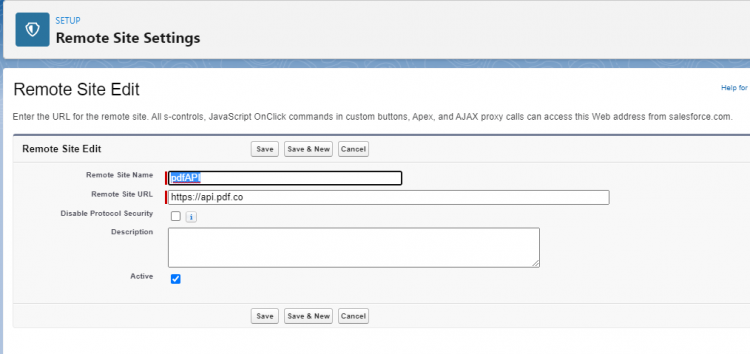
Step 2: Create Apex Class in Salesforce
Click the Gear icon on the upper right corner and open the Developer Console.
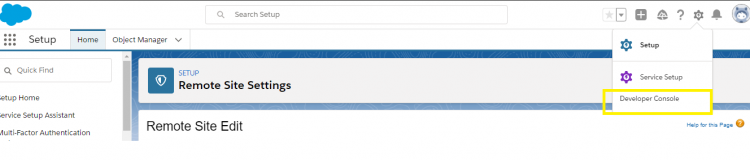
In the Developer Console window, click on File –> New –> Apex Class. Write the class name DocumentParserOutputAsCSV and click OK. Now copy the DocumentParserOutputAsCSV code in this file.
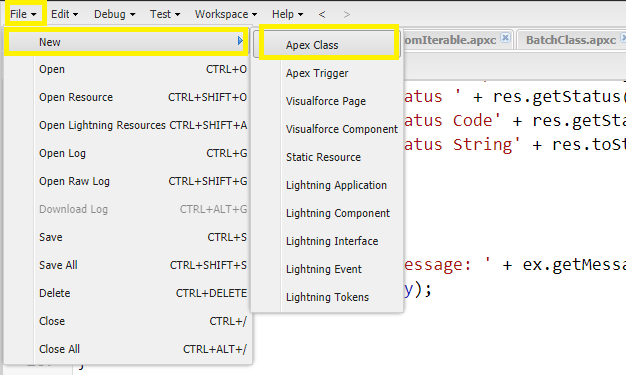
Similarly, create a new file with the name DocumentParserOutputAsCSVTest and copy the code.
Step 3: Add API Key in the File
In the DocumentParserOutputAsCSVTest file, add your PDF.co API Key. You can change the Destination file name which you want as a CSV.
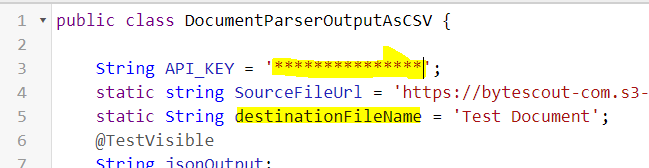
Step 4: Verify Code
To verify the code, open the execute Anonymous window and call the method below.
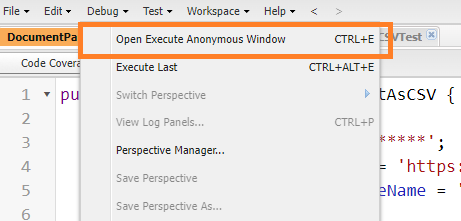
Then, click on Execute.
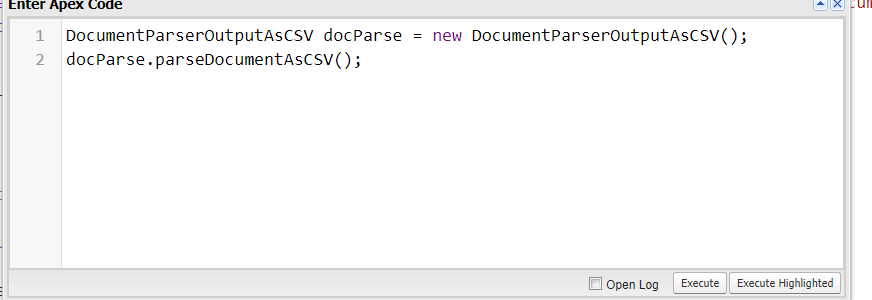
Step 5: Search Files
Now, open the App Launcher to view the output file.
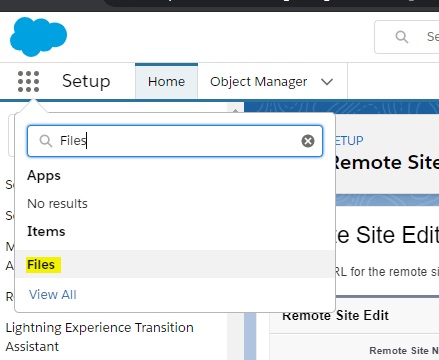
Step 6: Output CSV
Here’s the generated CSV output.
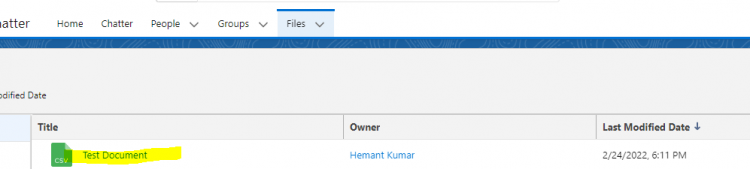
Source Code Files:
DocumentParserOutputAsCSV
public class DocumentParserOutputAsCSV {
String API_KEY = '***************';
static string SourceFileUrl = 'https://bytescout-com.s3-us-west-2.amazonaws.com/files/demo-files/cloud-api/document-parser/sample-invoice.pdf';
static String destinationFileName = 'Test Document';
@TestVisible
String jsonOutput;
public void parseDocumentAsCSV()
{
try
{
// Create HTTP client instance
Http http = new Http();
HttpRequest request = new HttpRequest();
// Set API Key
request.setHeader('x-api-key', API_KEY);
Boolean async = false;
String inline = 'true';
String profiles = '';
Boolean encrypt = false;
Boolean storeResult = false;
String generateCSVHead ='true';
// PDF document password. Leave empty for unprotected documents.
string Password = '';
// Prepare requests params as JSON
// See documentation: https://apidocs.pdf.co
// Create JSON payload
JSONGenerator gen = JSON.createGenerator(true);
gen.writeStartObject();
gen.writeStringField('url', SourceFileUrl);
gen.writeStringField('templateId', '1');
gen.writeStringField('outputFormat', 'CSV');
gen.writeStringField('generateCsvHeaders', generateCSVHead);
gen.writeBooleanField('async', async);
gen.writeBooleanField('encrypt', encrypt);
gen.writeStringField('inline', inline);
gen.writeStringField('password', password);
gen.writeEndObject();
// Convert dictionary of params to JSON
String jsonPayload = gen.getAsString();
// URL of 'documentparser' endpoint
string url = 'https://api.pdf.co/v1/pdf/documentparser';
request.setEndpoint(url);
request.setHeader('Content-Type', 'application/json;charset=UTF-8');
request.setMethod('POST');
request.setBody(jsonPayload);
// Execute request
HttpResponse response = http.send(request);
if(response.getStatusCode() == 200)
{
// Parse JSON response
Map<String, Object> json = (Map<String, Object>)JSON.deserializeUntyped(response.getBody());
if ((Boolean)json.get('error') == false)
{
jsonOutput = response.getBody();
saveDocument((String)json.get('body'));
System.debug('JSON :: '+ jsonOutput);
}
}
else
{
System.debug('Error Response ' + response.getBody());
System.Debug(' Status ' + response.getStatus());
System.Debug(' Status Code' + response.getStatusCode());
System.Debug(' Response String' + response.toString());
}
}
catch (Exception ex)
{
String errorBody = 'Message: ' + ex.getMessage() + ' -- Cause: ' + ex.getCause() + ' -- Stacktrace: ' + ex.getStackTraceString();
System.Debug(errorBody);
}
}
public static void saveDocument(String jsonResponse)
{
ContentVersion cv = new ContentVersion();
cv.Title = destinationFileName;
cv.PathOnClient = 'TestDocument.csv';
cv.VersionData = Blob.valueOf(jsonResponse);
cv.IsMajorVersion = true;
Insert cv;
}
}
DocumentParserOutputAsCSVTest
@isTest
private class DocumentParserOutputAsCSVTest {
@isTest static void testParseDocumentAsCSV()
{
Test.startTest();
Test.setMock(HttpCalloutMock.class, new DocumentParseHttpCalloutMock());
DocumentParserOutputAsCSV docParse = new DocumentParserOutputAsCSV();
docParse.parseDocumentAsCSV();
Test.stopTest();
}
@isTest static void testParseDocumentAsCSVForCatch()
{
DocumentParserOutputAsCSV docParse = new DocumentParserOutputAsCSV();
docParse.parseDocumentAsCSV();
}
public class DocumentParseHttpCalloutMock implements HttpCalloutMock {
public HTTPResponse respond(HTTPRequest request) {
// Create a fake response
HttpResponse response = new HttpResponse();
response.setHeader('Content-Type', 'application/json;charset=UTF-8');
response.setBody('{"body": "companyName,companyName2","pageCount": 1,"error": false,"status": 200,"name": "sample-invoice.csv","remainingCredits": 60804}');
response.setStatusCode(200);
return response;
}
}
}
Related Tutorials
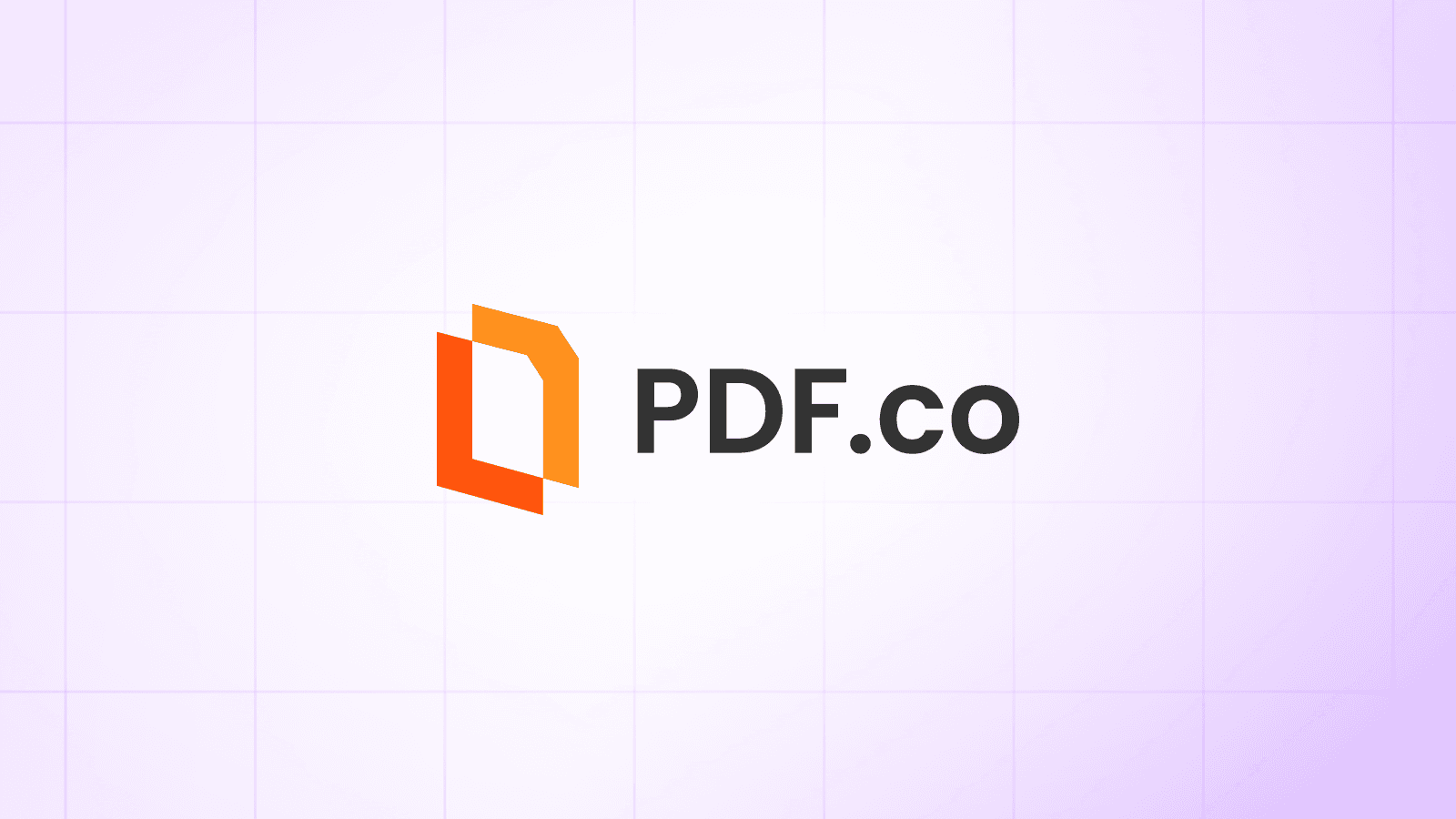
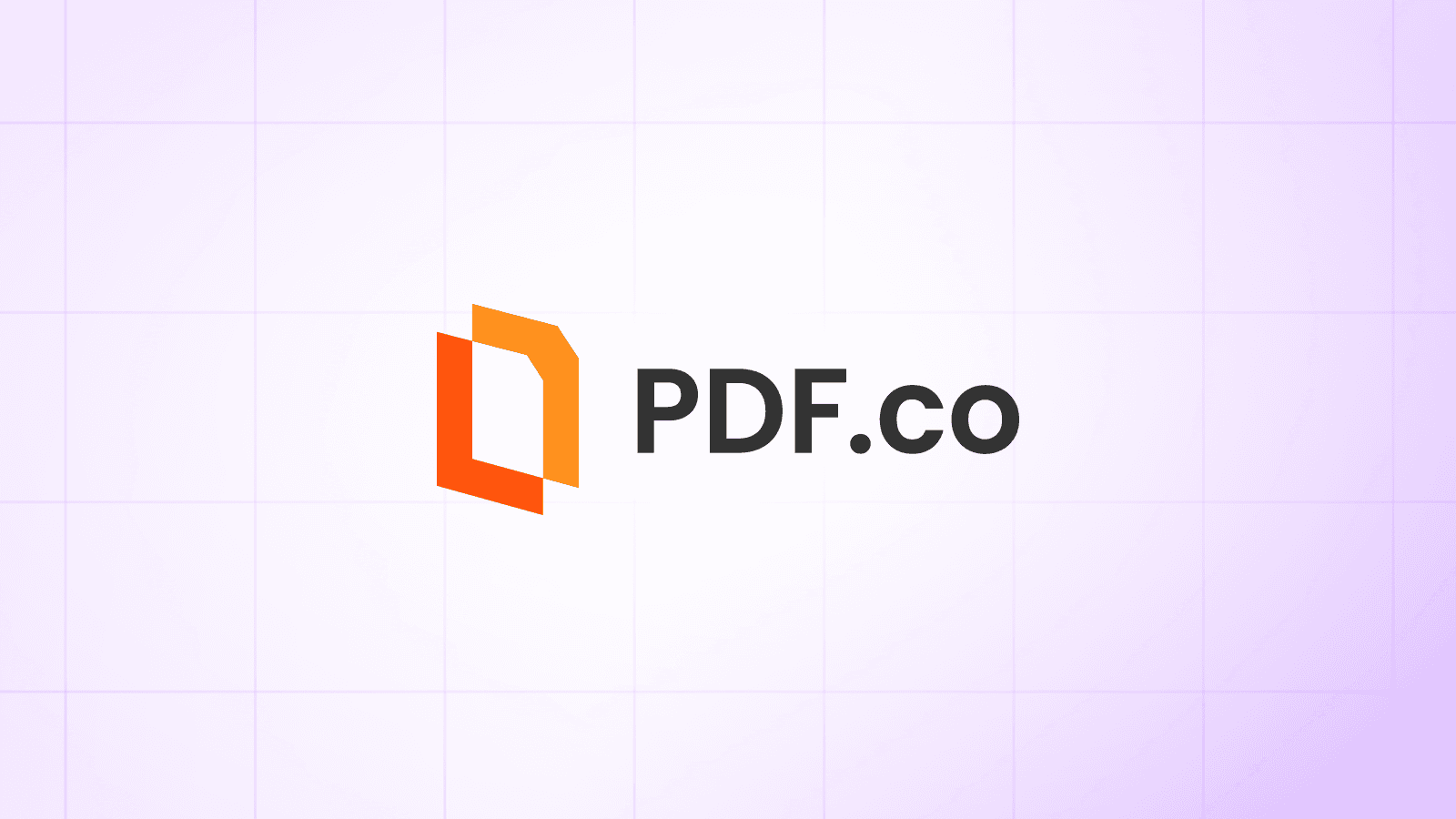
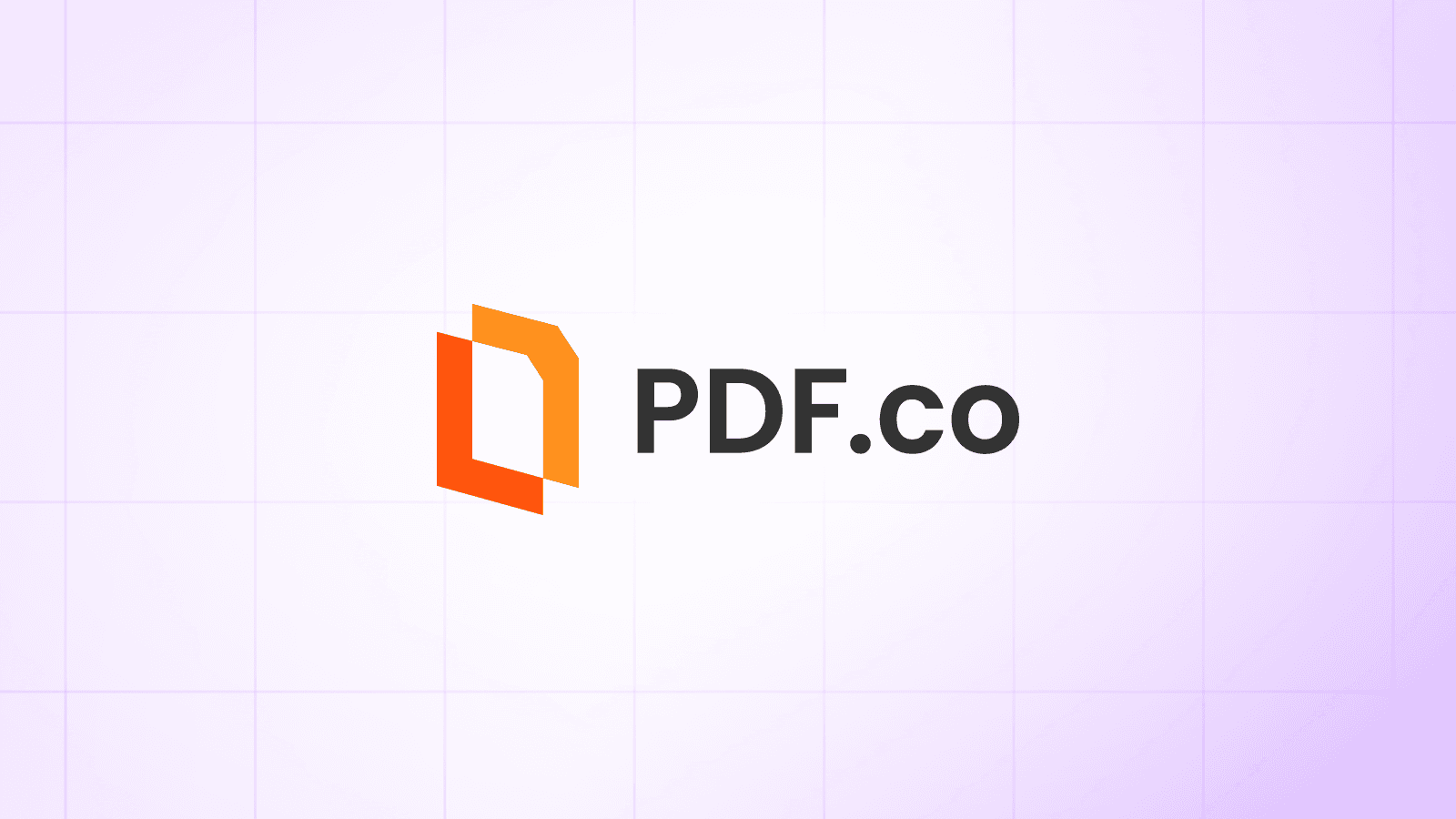
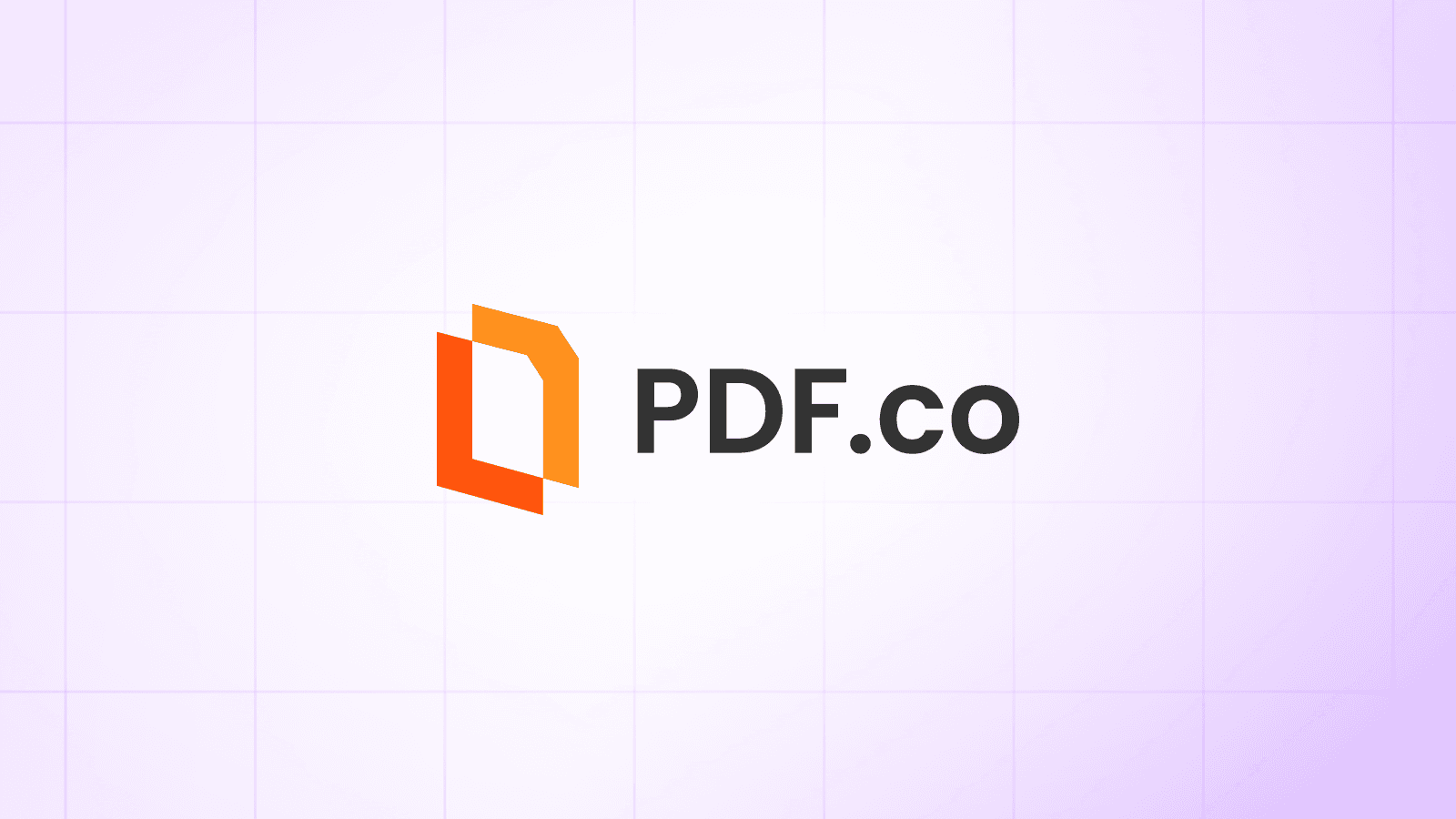