By including the async: true
parameter in the payload, the code can be adapted to utilize asynchronous processing. This enables non-blocking execution, meaning you don’t need to wait for the operation to complete immediately. Instead, the response will provide a job ID and a status URL. You can use the status URL to check the progress of the operation or set up webhooks to be notified once the task is complete. This approach is especially useful for handling large files or bulk operations efficiently.
How to Delete Pages from PDF using PDF.co Web API
This tutorial and the sample source code explain how to delete any page from a PDF document using JavaScript. This tutorial provides a complete process of deleting PDF pages using the PDF.co Web API.
Asynchronous processing allows you to handle large files or long-running tasks without waiting for the process to complete in a single API call. Below are images of our sample source files and the single PDF output.
For more details on asynchronous processing, refer to the PDF.co Documentation.
IN THIS TUTORIAL
Features of PDF.co Web API
The PDF.co Web API makes it easy to analyze and edit PDF documents using your preferred programming language. It allows you to modify PDF files, including analyzing and deleting pages. The API operates on a highly secure system, transmitting data through an encrypted connection to ensure your files are protected. By automating the document editing process, the API helps save both time and money.
With its asynchronous mode, you can process documents without needing to wait for operations to finish immediately. This feature improves scalability and efficiency, especially when handling large batches of files.
Below, you’ll find complete details about how to use the PDF.co Web API to delete pages, along with a demo and sample code that showcase these features in action.
Endpoint Parameters
Below is a brief explanation of the endpoint parameters of the PDF.co Web API to help you understand their functionality:
url
: (Required) Specifies the URL of the source file. The platform supports publicly accessible URLs, including those from Dropbox, Google Drive, and PDF.co’s built-in file storage.httpusername
: (Optional) Provides the username for authentication if the source URL requires it.httppassword
: (Optional) Contains the password for authentication if the source URL requires it.pages
: (Optional) Indicates which pages to delete from the PDF. You can specify:- A single page (e.g., 1)
- A range of pages using a dash (e.g., 1-3)
- Multiple pages or ranges separated by commas (e.g., 1,3,5-7).
- If left empty, all pages in the PDF will be deleted. This parameter must be provided as a string.
name
: (Optional) Allows you to name the output file. This parameter must be a string.expiration
: (Optional) Sets the expiration time for the output URL in PDF.co’s temporary file storage.profiles
: (Optional) Configures custom settings. This parameter must be a string.async
: (Optional) When set totrue
, the process runs asynchronously. The response will include a job ID and a status URL. You can use the status URL to check progress or configure webhooks for task completion notifications.
Example Using JavaScript
Below is the source code to demonstrate how you can delete specific pages from a sample PDF document using the PDF.co Web API. The example is written in JavaScript and shows how to efficiently edit a PDF document using some of the API parameters.
You can specify the URL of any document you wish to edit, ensuring efficient and secure alterations through this API. To complete this process, follow these steps:
- Provide your API Key: The PDF.co platform generates an API key for you upon logging in. Include this key in your program.
- Specify the URL of the PDF Document: Add the URL for the document you want to edit in the API request.
- Resulting File: After executing the program, the PDF.co API generates a resulting file. You can either download this file or visit the provided URL to see the changes made to the PDF document.
The sample code below illustrates how to use these features effectively.
Sample Code Snippet
The following source code contains the program to delete pages from a PDF. You can view the full code here.
// Requiring modules
const express = require('express');
const axios = require('axios');
// Creating express object
const app = express();
const options = {
method: 'POST',
url: 'https://api.pdf.co/v1/pdf/edit/delete-pages',
headers: {
'x-api-key': '*******************************************************',
'Content-Type': 'application/json',
},
data: {
url: 'https://juventudedesporto.cplp.org/files/sample-pdf_9359.pdf',
pages: '1-3',
name: 'result.pdf',
async: true // Enable asynchronous processing
}
};
// Sending the request
axios(options)
.then((response) => {
console.log(response.data);
// In async mode, check for job ID and status URL in the response
})
.catch((error) => {
console.error(error);
});
Sample Code Walkthrough
Following is the step by step explanation of the sample source code:
Importing Required Modules
- Express: A Node.js framework for building web applications, aiding in server-side logic and networking.
- Axios: A Node.js module that simplifies making HTTP calls.
These modules are included using the require
function, which loads essential files such as Node.js, community, and local modules.
Setting Up API Access
- Use the
require
parameter to include theexpress
andaxios
modules in your application. - Specify the request method (e.g., POST), the API endpoint URL, and your API key.
- After logging into the PDF.co platform, you can obtain your unique API key, which acts as an access token for authentication. Add this key in the header of your request.
Providing Data for Alteration
Define the payload with relevant information:
- The URL of the document to be edited.
- The pages to delete (specified as a string).
- The name of the resulting file.
Adding Asynchronous Processing
Include the async: true
parameter in the payload to enable asynchronous processing. This allows the operation to run without blocking other tasks.
Sending the Request and Handling the Response
- Make the API call using
axios
and handle the response. - In asynchronous mode, the response includes a
jobId
and astatus
URL. - Use the
status
URL to monitor progress. You can either poll this URL or set up a webhook to be notified when the task is complete.
Downloading the Altered File
- Once the process is complete, retrieve the resulting file using the provided URL.
- You can either:
- View the edited document online using the resulting URL.
- Write a custom function to download the file effectively from the response URL.
Error Handling
If the request is unsuccessful, review and modify the program to fix errors and achieve the desired output.
Running the Application
Optionally, configure the application to run on a specific port number and handle GET requests if needed.
Output
Sample PDF Document
Following is the beginning of the sample document for this example:
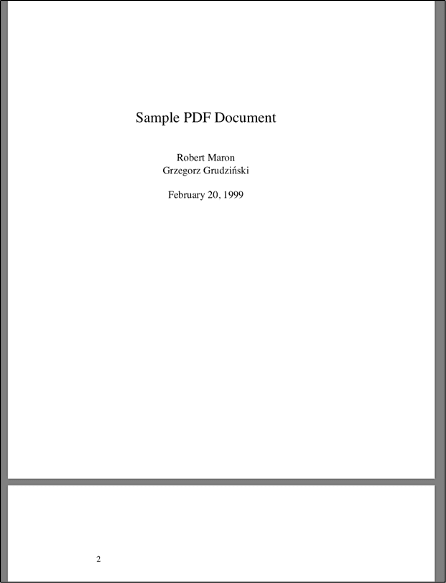
Output PDF Document
Below is the screenshot of the edited sample document after deleting pages 1, 2, and 3 for visualization:
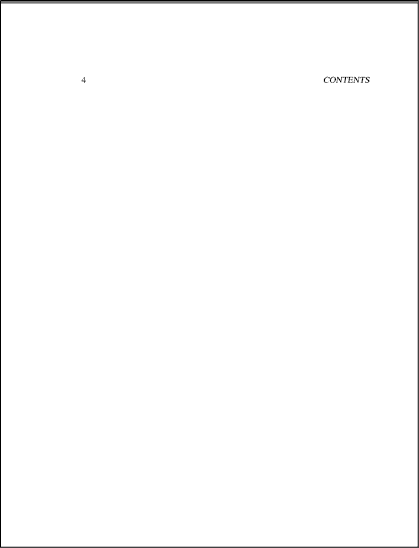
In this tutorial, you learned how to delete specific pages from a PDF document using JavaScript. The tutorial provided source code, a demo, and a step-by-step explanation of the process, showcasing how to use the PDF.co Web API effectively. It also highlighted the benefits of asynchronous processing for improved efficiency. For more details, visit the PDF.co Documentation.
Related Tutorials
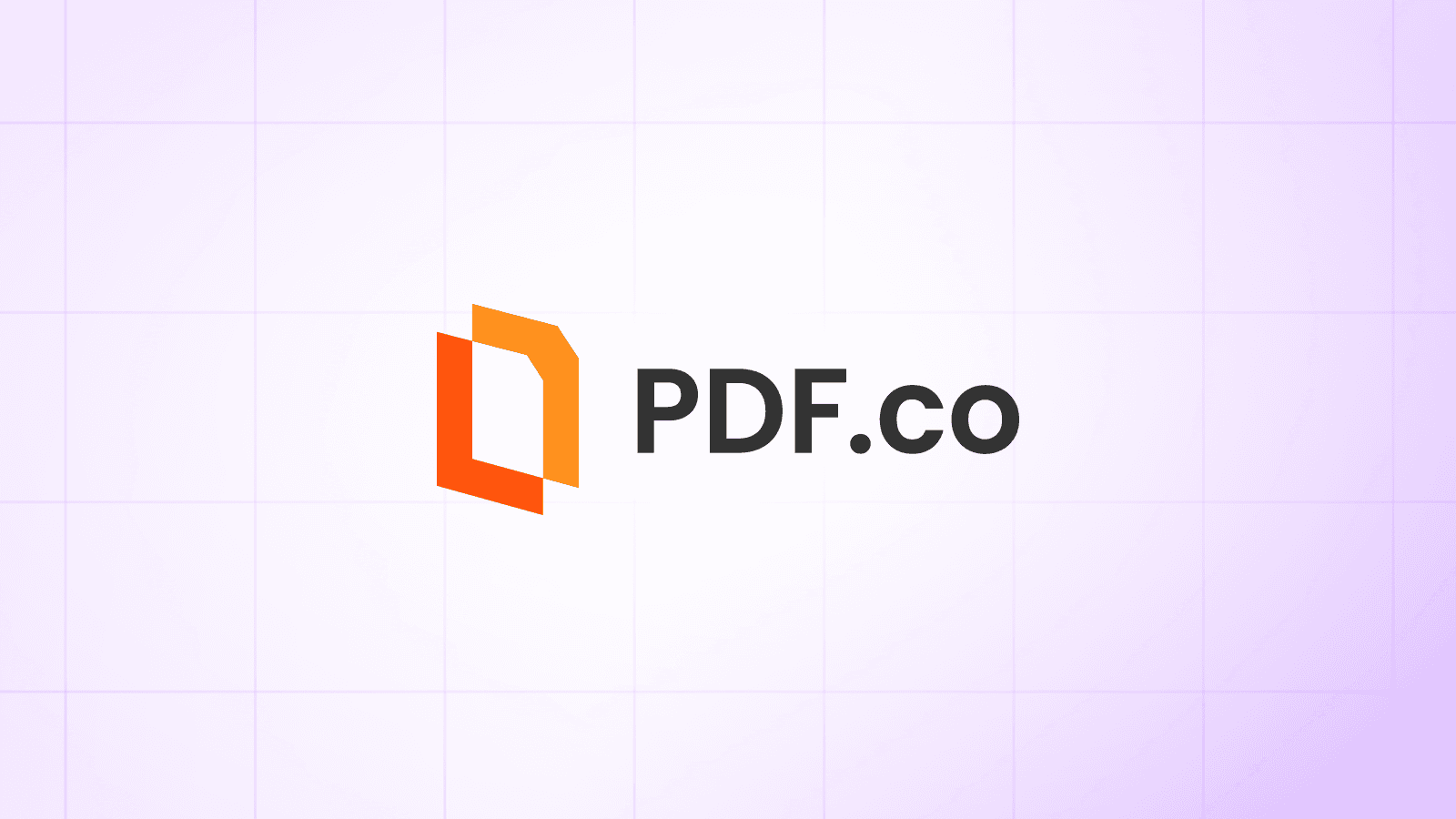
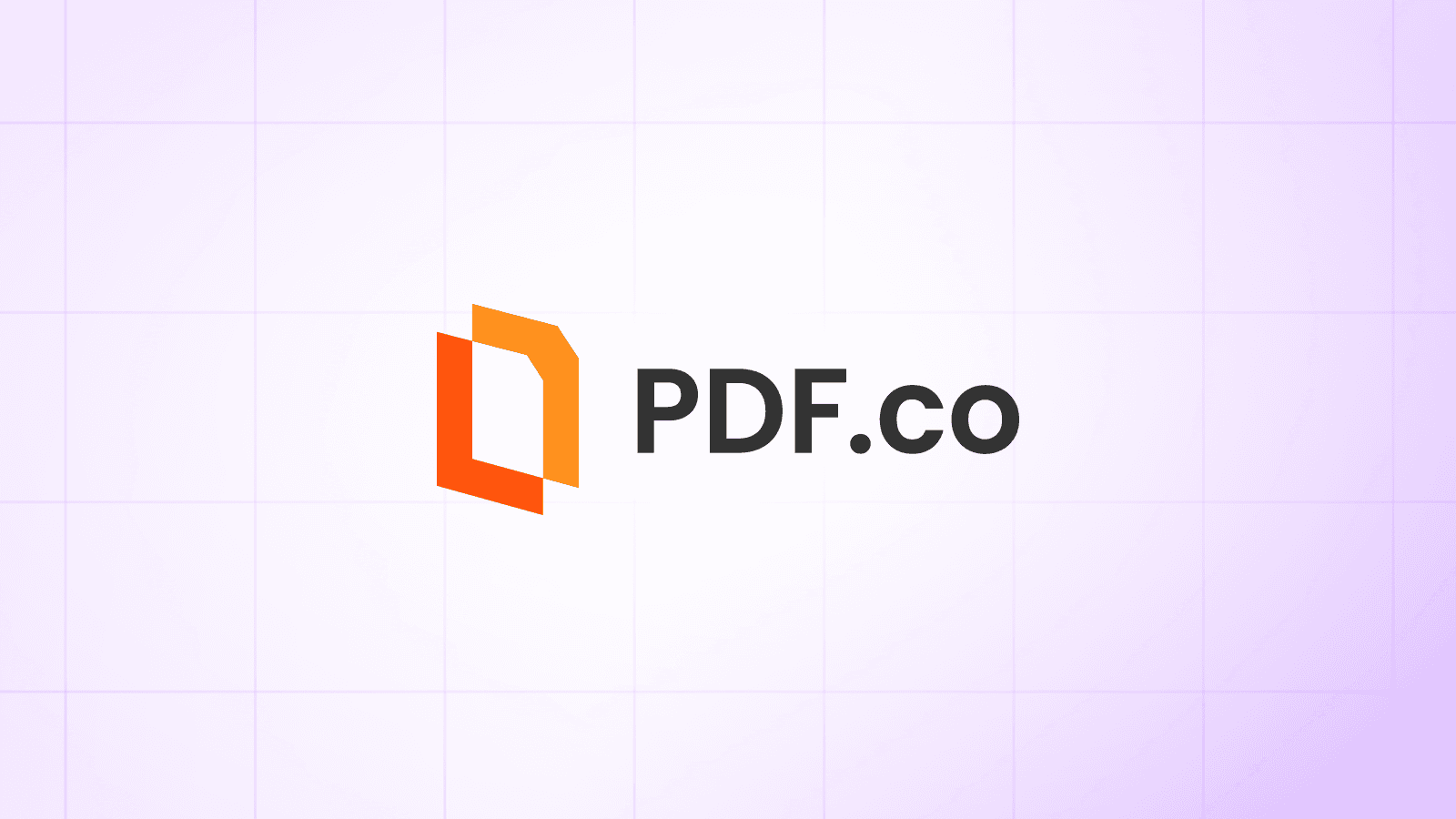
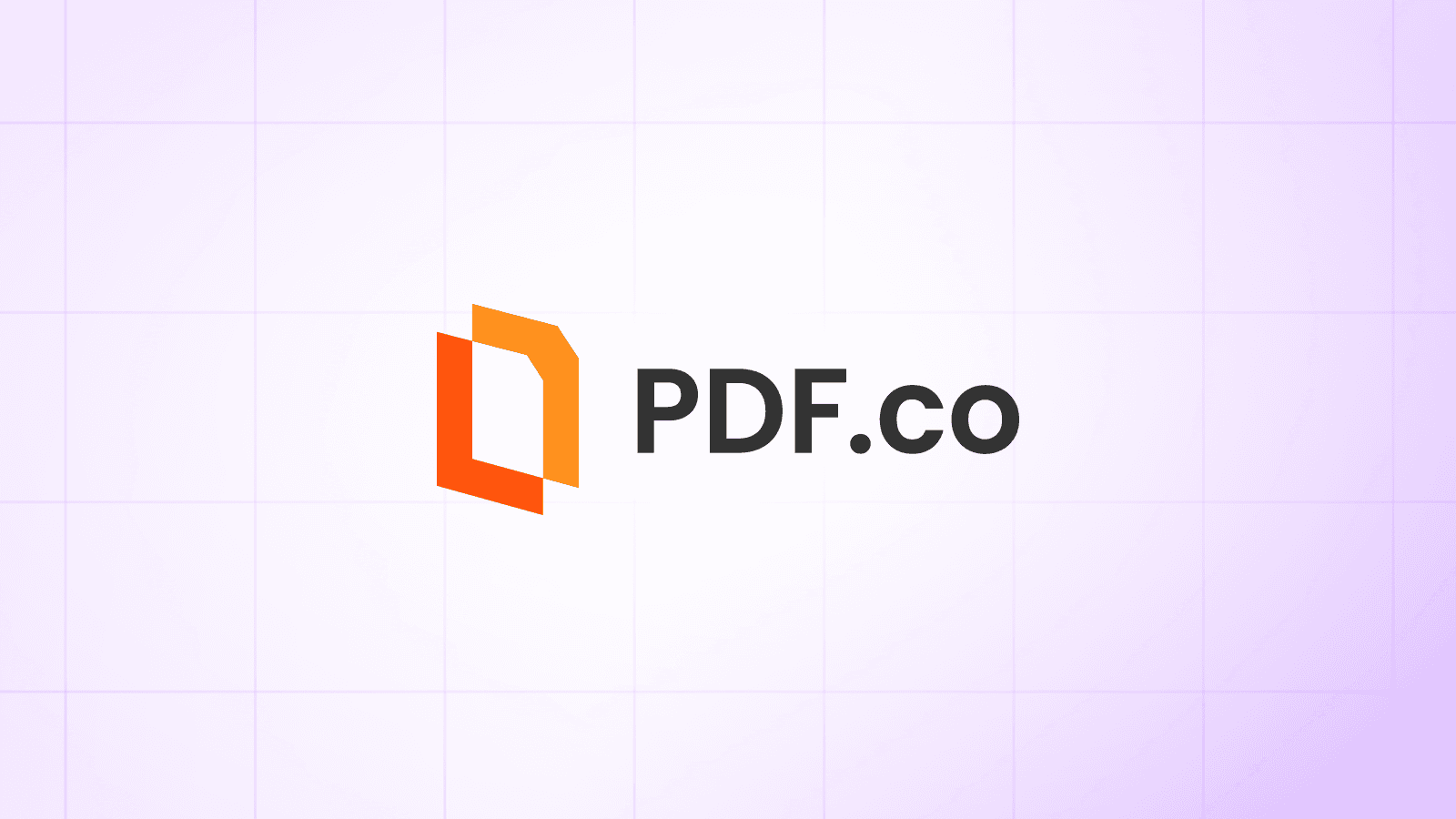
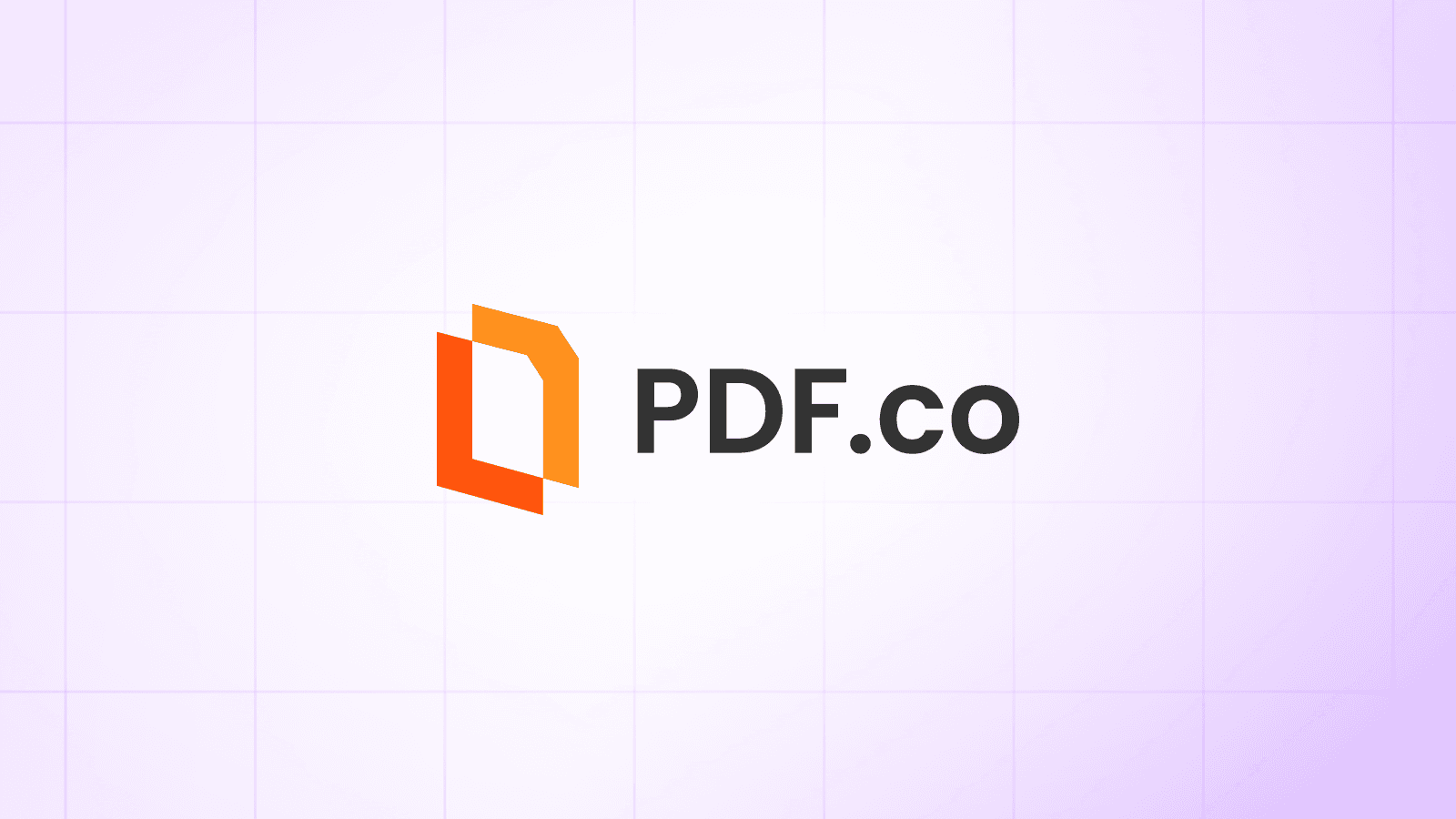