How to Fill PDF Forms using PDF.co Web API in JavaScript
This tutorial and the sample source code explain filling a PDF form using the filling functionality from PDF.co Web API using Javascript programming language. The users can use PDF Form Filler API to interactively fill their respective PDF documents with their data and create new PDF forms using the same endpoint.
Features of PDF Form Filler API Endpoint
The PDF.co Web API provides tools to fill a form by benefiting from easy field-name mapping, e-signature support, and an on-premise API server. The PDF.co Form Filler API endpoint works extremely fast to fill out forms rather than manually handling them. The data to be filled can be from different sources such as spreadsheets, CRMs, and user input.
A PDF form can include text fields, checkboxes, radio buttons, and combo boxes. There is also an option to embed e-signature and other important image objects the document needs. Below is a detailed demo explaining these features to help users understand them more easily.
PDF.co Web API provides high security. Moreover, the API transmits the user’s documents and data files via encrypted connections. Users can learn more about the PDF.co API security here.
Endpoint Parameters for PDF Form Filler
Following are the parameters of the PDF Form Filler API.
- url: It is a required parameter which should be a string containing the form URL. When the user creates the request, the API takes the form from this parameter and modifies it. Moreover, the user can edit more than one form simultaneously by providing a comma-separated URL of the source files.
- httpusername: It is an optional parameter that takes the auth user name if it is needed to access the source file.
- httppassword: It is an optional parameter that takes the auth password if it is needed to access the source file.
- password: The user must provide the file password if he is editing the locked file.
- annotations: It is an optional parameter which is an array of objects. The users can use this parameter to add objects at the top of the PDF document. The users can read about this object’s parameters here.
- fields: It is an optional parameter that accepts the value arrays to update the editable fields. The users can read about this object’s parameters here.
- images: It is an optional parameter accepting image objects array to add images in the pdf form. The users can read about this object’s parameters here.
- inline: It is an optional parameter that accepts boolean values. The users can set it to “true” to get the resulting file’s URL directly in the output without enclosing it in the json object.
- Expiration: It is an optional parameter to set output link expiration time in minutes. Its default value is set to “60,” meaning one hour. The users can change the time limit according to their subscription plan.
- encrypt: It is an optional parameter whose value is false by default, and the users can encrypt the output file using this parameter.
- async: It is an optional parameter with boolean values. The users can make the processes run asynchronously using it.
- name: An optional parameter takes the string with the output file name.
- profiles: It is an optional parameter to set custom configuration.
Fill PDF Forms – Example Using Javascript
The following source code shows users how to fill a sample PDF form using the PDF.co Form Filler API. The sample code in Javascript shows how to fill a sample form such as IRS Form 4506. This form mainly consists of some text fields and checkboxes along with the signature in the form. The users can use the Get PDF Info tool to get the field names in an interactive PDF. The users can access it in the Helpers Tools menu in their respective PDF.co accounts. A direct link to the feature is here.
Moreover, the users can upload their PDF documents to get critical information about their respective documents, such as the text fields, edit boxes, checkboxes, page numbers, and the location of every field to help the filling process efficiently.
The code contains IRS Form 4506 as an example here. The user needs to provide an API key generated by the PDF.co login and the pdf file URL in the API request for the API to work. Moreover, the pdf.co API returns the resulting or edited file URL from which the user can download the file and keep it on his local storage. The result.pdf file contains the updated document after executing the code and fills the document with the required necessary information.
Sample Code Snippet for PDF Form Filling
Following is an example code to fill PDF form using PDF.co Web API:
var https = require("https");
var path = require("path");
var fs = require("fs");
// The authentication key (API Key).
const API_KEY = "********************************";
// Direct URL of source PDF file
const SourceFileUrl = "https://www.irs.gov/pub/irs-pdf/f4506t.pdf";
// Destination PDF file name
const DestinationFile = "./result.pdf";
// Prepare request to `PDF Edit` API endpoint
var queryPath = `/v1/pdf/edit/add`;
//set it to true to run the process asynchronously
const async = false;
// Form field data
var fields = [
{
"fieldName": "topmostSubform[0].Page1[0].f1_1[0]",
"pages": "0",
"text": "John A."
},
{
"fieldName": "topmostSubform[0].Page1[0].f1_02[0]",
"pages": "0",
"text": "Doe"
},
{
"fieldName": "topmostSubform[0].Page1[0].f1_03[0]",
"pages": "0",
"text": "XYZ"
},
{
"fieldName": "topmostSubform[0].Page1[0].f1_4[0]",
"pages": "0",
"text": "123456789"
},
{
"fieldName": "topmostSubform[0].Page1[0].f1_5[0]",
"pages": "0",
"text": "Las Vegas, Nevada"
},
{
"fieldName": "topmostSubform[0].Page1[0].f1_6[0]",
"pages": "0",
"text": "ABCDE"
},
{
"fieldName": "topmostSubform[0].Page1[0].customer_file_number[0]",
"pages": "0",
"text": "987654321"
},
{
"fieldName": "topmostSubform[0].Page1[0].c1_1[1]",
"pages": "0",
"text": "True"
},
{
"fieldName": "topmostSubform[0].Page1[0].f1_24[0]",
"pages": "0",
"text": "5"
},
{
"fieldName": "topmostSubform[0].Page1[0].f1_25[0]",
"pages": "0",
"text": "01"
},
{
"fieldName": "topmostSubform[0].Page1[0].f1_26[0]",
"pages": "0",
"text": "20"
}
];
// * Fill forms *
// JSON payload for api request
var jsonPayload = JSON.stringify({
name: path.basename(DestinationFile),
url: SourceFileUrl,
async: async,
fields: fields
});
var reqOptions = {
host: "api.pdf.co",
method: "POST",
path: queryPath,
headers: {
"x-api-key": API_KEY,
"Content-Type": "application/json",
"Content-Length": Buffer.byteLength(jsonPayload, 'utf8')
}
};
// Send request
var postRequest = https.request(reqOptions, (response) => {
response.on("data", (d) => {
// Parse JSON response
var data = JSON.parse(d);
if (data.error == false) {
// Download the PDF file
var file = fs.createWriteStream(DestinationFile);
https.get(data.url, (response2) => {
response2.pipe(file).on("close", () => {
console.log(`Generated PDF file saved to '${DestinationFile}' file.`);
});
});
}
else {
// Service reported error
console.log(data.message);
}
});
}).on("error", (e) => {
// Request error
console.error(e);
});
// Write request data
postRequest.write(jsonPayload);
postRequest.end();
Filled PDF Form – Output
Below is the screenshot of the edited form:
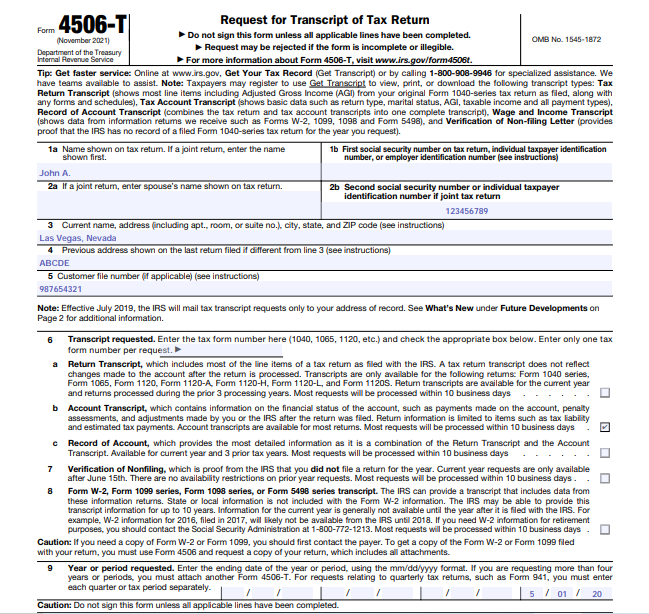
Sample Guide to Fill PDF Forms Using PDF.co Web API
- The users must import the necessary packages to send API requests and download the resulting PDF file. In this scenario, the required packages are “path,” “https,” and “fs.”
- After logging in to the PDF.co website, the users can obtain their API key after logging in to the PDF.co website to access their Web API. As the users can not send a direct request, they have to use this specific API key as an access token in the header for authentication.
- The best practice is to use this API key in variable declaration. The users will have to change only the variable and can edit the whole file easily without going into the code details.
- Declare and initialize the source file, destination file, and query path variables.
- Upload the relevant PDF document using the Helper Tools menu and get the field mapping. Pick the required fields from the information and verify the page numbers and field locations to fill the required fields in the form.
- Moreover, check the fields’ width to put only the critical information according to the limited width to avoid inconvenience.
- Provide the relevant information in the payload—for example, the field names, page numbers, and the information to be added. Moreover, the users can add image objects in the payload to include signatures in the PDF form.
- If the file is large, the users should use the “async” parameter to run the process asynchronously for smooth working.
- Send the POST request to the API endpoint and wait for its response. If there is no error, then use javascript’s file stream to download the file and store it on the local storage. Otherwise, print the error message on the console so the user can understand and eradicate it. The users can opt not to download the file, keep it online, and use the resulting file’s URL in the API response for viewing it.
Related Tutorials
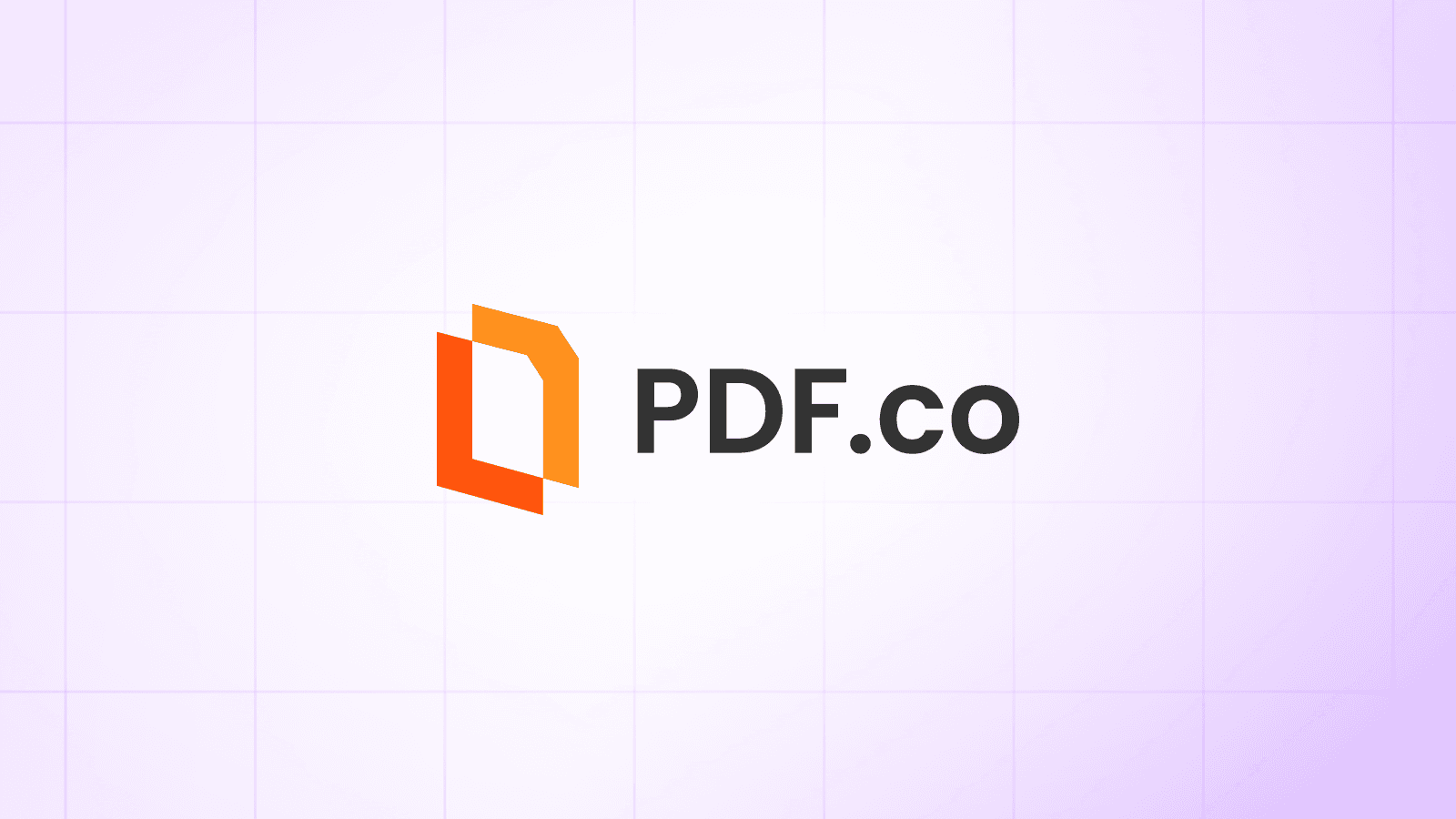
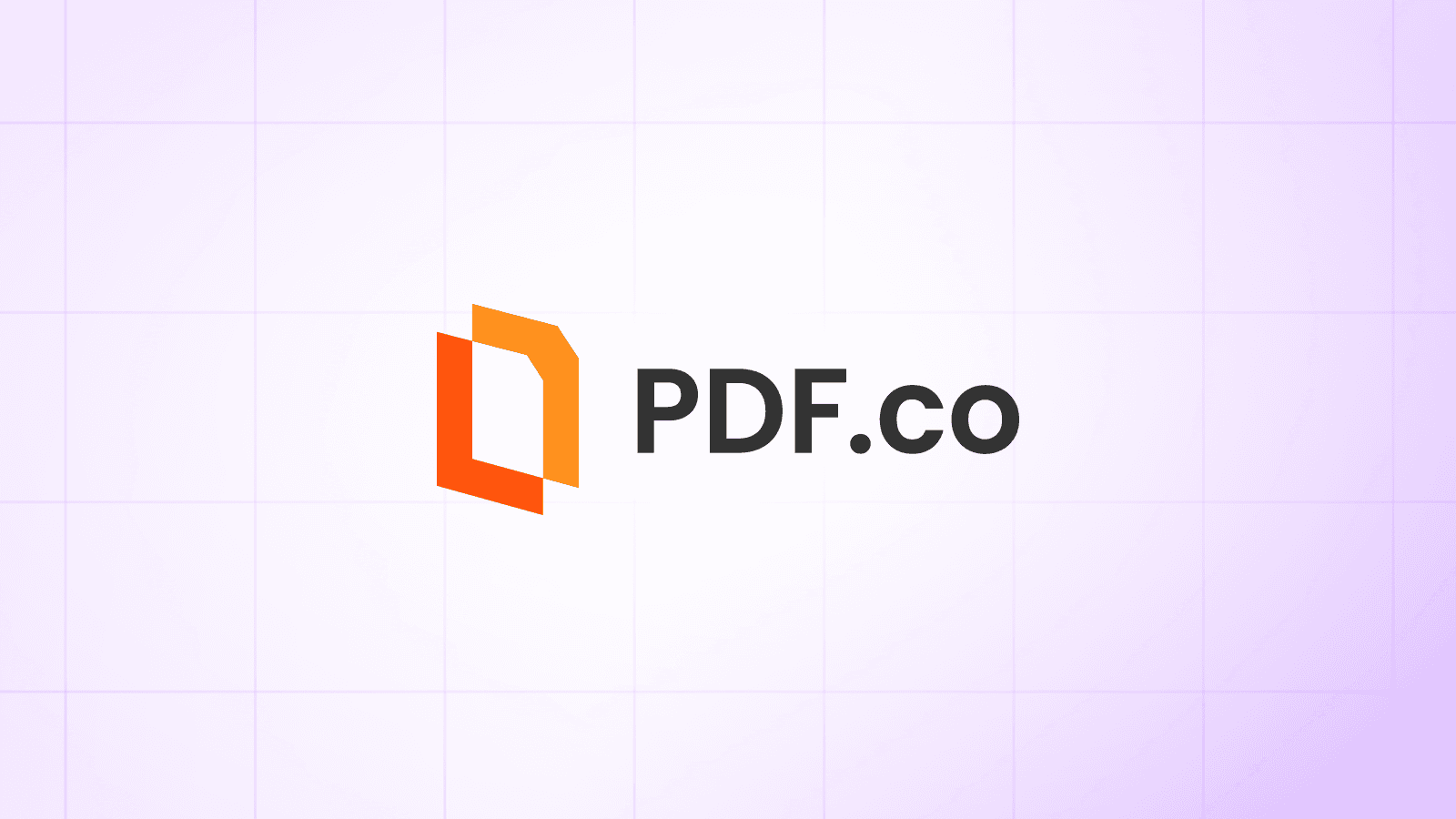
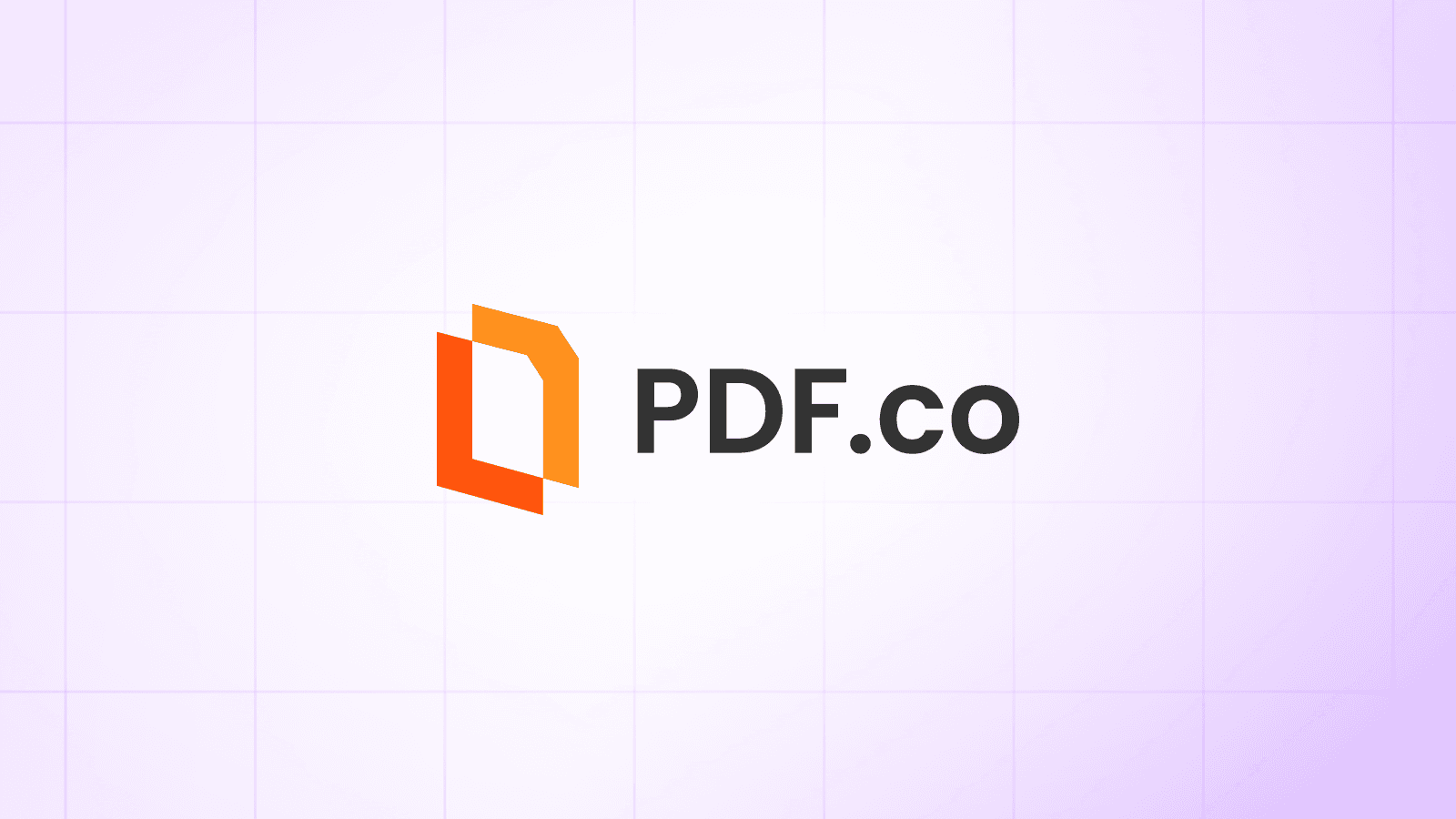
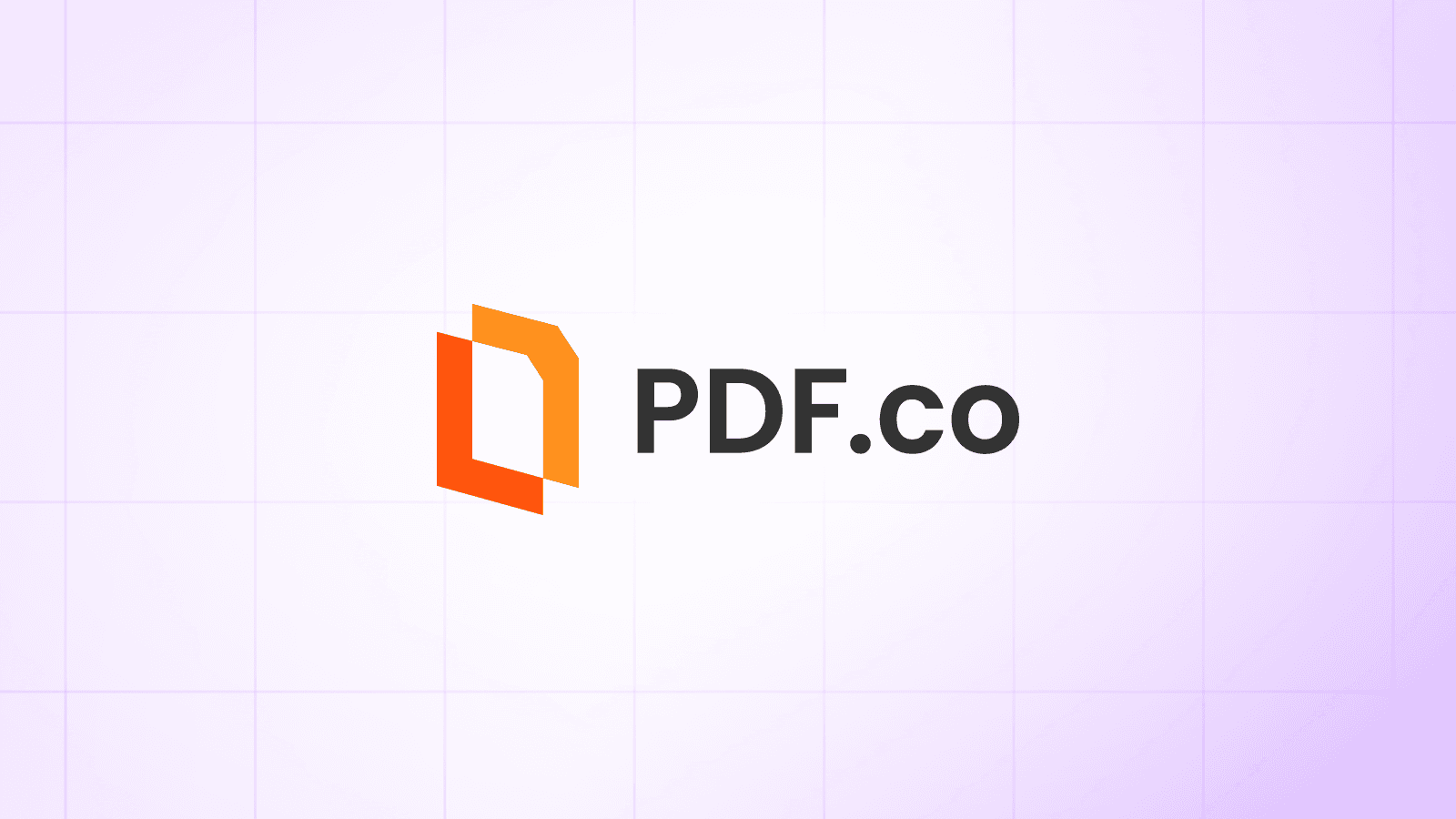