Add Text and Images to PDF with SharePoint and PDF.co
Sep 2, 2024·8 Minutes Read
In this short article, we’ll demonstrate source code for how to add Text or Images in PDF using SharePoint and PDF.co. Without any delay let’s jump to source code.
Add Text and Images to PDF – Source Code Snippets
Check out the markup for Web Part visual control.
VisualWebPart1UserControl.ascx
<%@ Assembly Name="$SharePoint.Project.AssemblyFullName$" %>
<%@ Assembly Name="Microsoft.Web.CommandUI, Version=15.0.0.0, Culture=neutral, PublicKeyToken=71e9bce111e9429c" %>
<%@ Register Tagprefix="SharePoint" Namespace="Microsoft.SharePoint.WebControls" Assembly="Microsoft.SharePoint, Version=15.0.0.0, Culture=neutral, PublicKeyToken=71e9bce111e9429c" %>
<%@ Register Tagprefix="Utilities" Namespace="Microsoft.SharePoint.Utilities" Assembly="Microsoft.SharePoint, Version=15.0.0.0, Culture=neutral, PublicKeyToken=71e9bce111e9429c" %>
<%@ Register Tagprefix="asp" Namespace="System.Web.UI" Assembly="System.Web.Extensions, Version=4.0.0.0, Culture=neutral, PublicKeyToken=31bf3856ad364e35" %>
<%@ Import Namespace="Microsoft.SharePoint" %>
<%@ Register Tagprefix="WebPartPages" Namespace="Microsoft.SharePoint.WebPartPages" Assembly="Microsoft.SharePoint, Version=15.0.0.0, Culture=neutral, PublicKeyToken=71e9bce111e9429c" %>
<%@ Control Language="C#" AutoEventWireup="true" CodeBehind="VisualWebPart1UserControl.ascx.cs" Inherits="AddTextAndImagesToPDFWebPart.VisualWebPart1.VisualWebPart1UserControl" %>
<br />
<asp:Button ID="StartButton" runat="server" OnClick="StartButton_Click" Text="Start" style="width: 610px; padding-left: 0px; margin-left: 0px; padding-right: 0px; padding-right: 0px;"/>
<br />
<br />
Log<br />
<asp:TextBox ID="LogTextBox" runat="server" Height="300px" TextMode="MultiLine" Width="600px"></asp:TextBox>
<br />
This is code behind for Web Part User Control.
VisualWebPart1UserControl.ascx.cs
using Microsoft.SharePoint;
using Newtonsoft.Json.Linq;
using System;
using System.Globalization;
using System.IO;
using System.Net;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
namespace AddTextAndImagesToPDFWebPart.VisualWebPart1
{
public partial class VisualWebPart1UserControl : UserControl
{
public SPWeb CurrentWeb { get; set; }
// The authentication key (API Key).
// Get your own by registering at https://app.pdf.co/documentation/api
string API_KEY = Utils.API_KEY;
// Direct URL of source PDF file.
// You can also upload your own file into PDF.co and use it as url. Check "Upload File" samples for code snippets: https://github.com/bytescout/pdf-co-api-samples/tree/master/File%20Upload/
const string SourceFileUrl = "https://bytescout-com.s3.amazonaws.com/files/demo-files/cloud-api/pdf-edit/sample.pdf";
// Comma-separated list of page indices (or ranges) to process. Leave empty for all pages. Example: '0,2-5,7-'.
const string Pages = "";
// PDF document password. Leave empty for unprotected documents.
const string Password = "";
// Destination PDF file name
const string DestinationFile = "result.pdf";
const string DestinationFileImage = "result_image.pdf";
const string DestinationLibName = "Shared Documents";
// Text annotation params
const string Type = "annotation";
const int X = 400;
const int Y = 600;
const string Text = "APPROVED";
const string FontName = "Times New Roman";
const float FontSize = 24;
const string FontColor = "FF0000";
// Image params
private const int X1 = 400;
private const int Y1 = 20;
private const int Width1 = 119;
private const int Height1 = 32;
private const string ImageUrl = "https://bytescout-com.s3.amazonaws.com/files/demo-files/cloud-api/pdf-edit/logo.png";
protected void Page_Load(object sender, EventArgs e)
{
}
protected void StartButton_Click(object sender, EventArgs e)
{
// Create standard .NET web client instance
ServicePointManager.Expect100Continue = true;
ServicePointManager.SecurityProtocol = SecurityProtocolType.Tls12;
WebClient webClient = new WebClient();
// Set API Key
webClient.Headers.Add("x-api-key", API_KEY);
// * Add text annotation *
// Prepare requests params as JSON
// See documentation: https://apidocs.pdf.co/04-pdf-add-text-signatures-and-images-to-pdf
string jsonPayload = $@"{{
""name"": ""{DestinationFile}"",
""url"": ""{SourceFileUrl}"",
""password"": ""{Password}"",
""annotations"": [
{{
""x"": {X},
""y"": {Y},
""text"": ""{Text}"",
""fontname"": ""{FontName}"",
""size"": ""{FontSize.ToString(CultureInfo.InvariantCulture)}"",
""color"": ""{FontColor}"",
""pages"": ""{Pages}""
}}
],
""images"": [
{{
""url"": ""{ImageUrl}"",
""x"": {X1},
""y"": {Y1},
""width"": {Width1},
""height"": {Height1},
""pages"": ""{Pages}""
}}
]
}}";
try
{
// URL of "PDF Edit" endpoint
string url = "https://api.pdf.co/v1/pdf/edit/add";
// Execute POST request with JSON payload
string response = webClient.UploadString(url, jsonPayload);
LogTextBox.Text += String.Format("Add text \"{0}\" to pdf file \"{1}\". \n", Text, SourceFileUrl);
LogTextBox.Text += String.Format("Add image \"{0}\" to pdf file. \n", ImageUrl);
// Parse JSON response
JObject json = JObject.Parse(response);
if (json["error"].ToObject() == false)
{
// Get URL of generated PDF file
string resultFileUrl = json["url"].ToString();
// Download generated PDF file
var retData = webClient.DownloadData(resultFileUrl);
//Upload file to SharePoint document linrary
//Read create stream
using (MemoryStream stream = new MemoryStream(retData))
{
//Get handle of library
SPFolder spLibrary = CurrentWeb.Folders[DestinationLibName];
//Replace existing file
var replaceExistingFile = true;
//Upload document to library
SPFile spfile = spLibrary.Files.Add(DestinationFile, stream, replaceExistingFile);
spLibrary.Update();
}
LogTextBox.Text += String.Format("Generated PDF file saved as \"{0}\\{1}\" file. \n", DestinationLibName, DestinationFile);
}
else
{
LogTextBox.Text += json["message"].ToString() + " \n";
}
}
catch (Exception ex)
{
LogTextBox.Text += ex.ToString() + " \n";
}
finally
{
webClient.Dispose();
}
LogTextBox.Text += "\n";
LogTextBox.Text += "Done...\n";
}
}
}
Source Code at GitHub
You can explore the full source code for this sample at this GitHub link.
Add Text and Images to PDF – Sample Screenshots
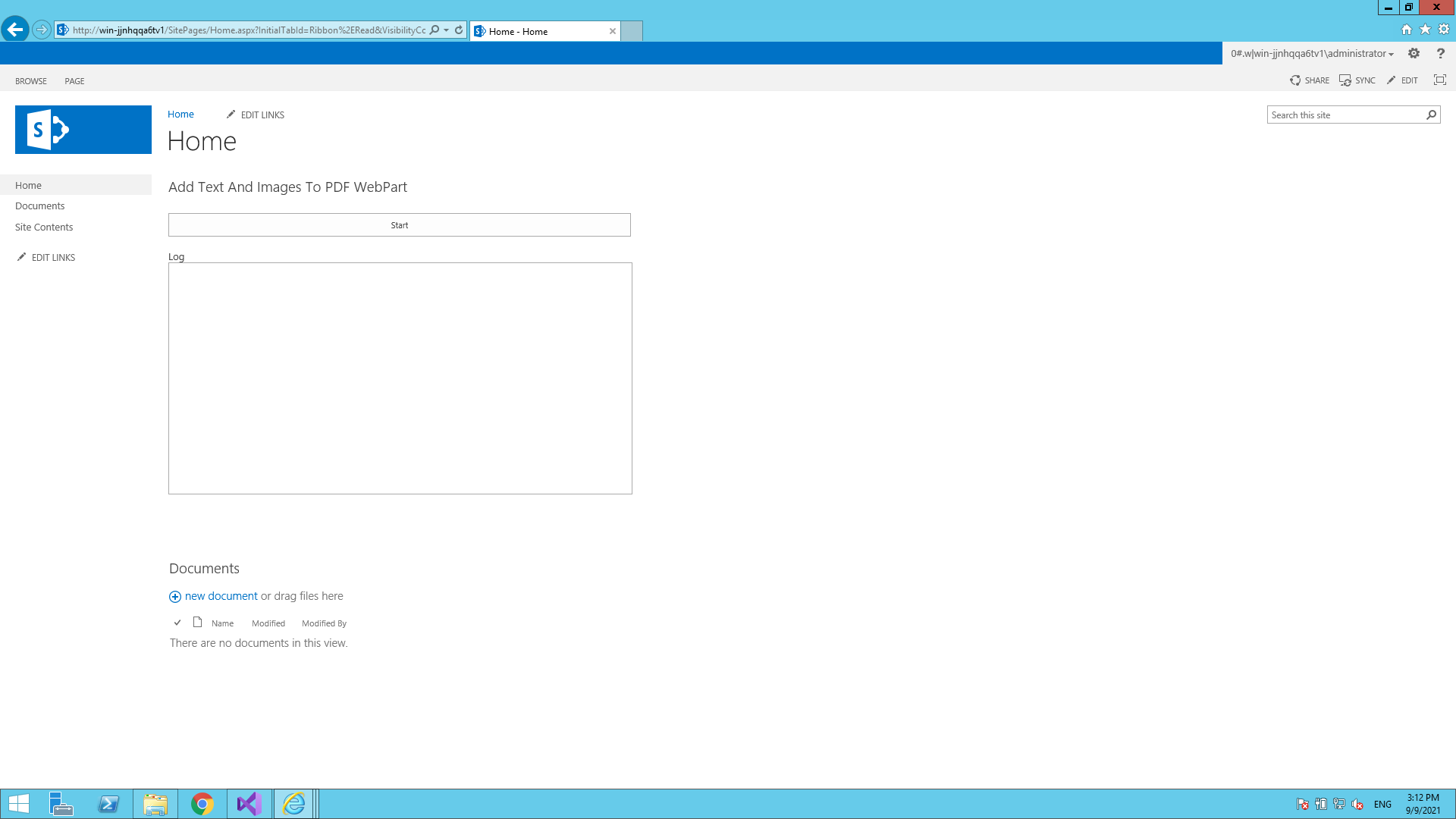
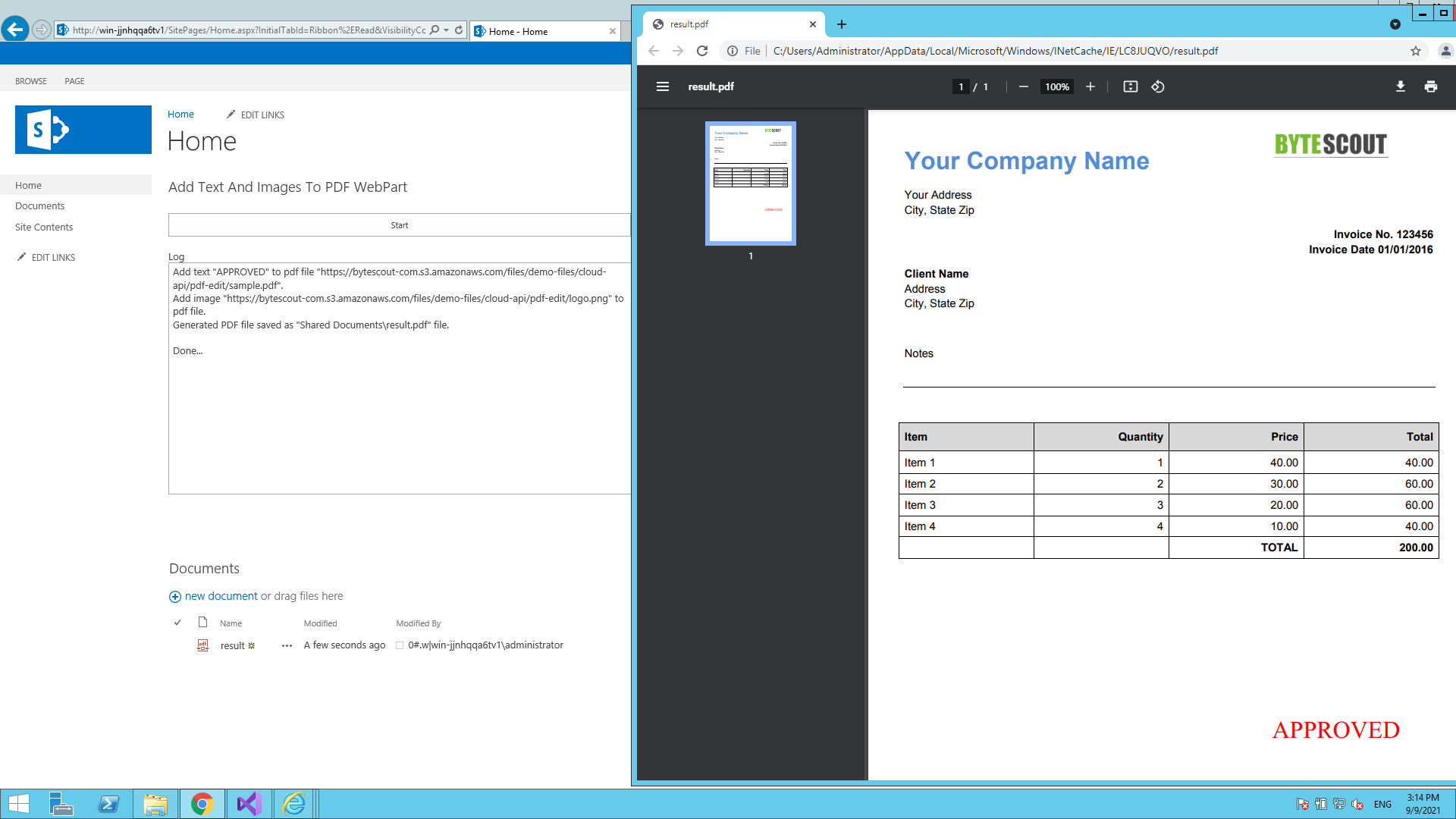
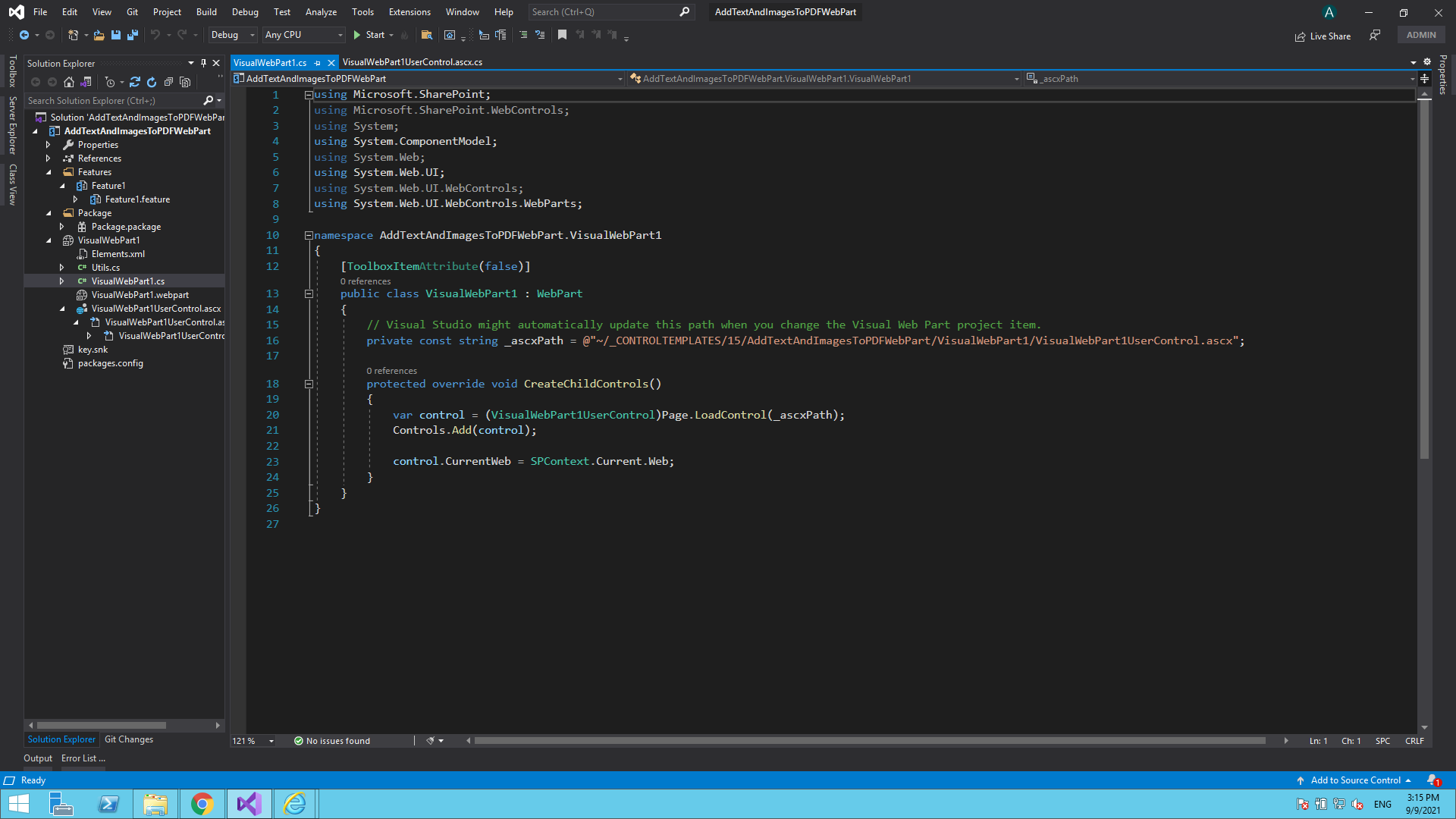
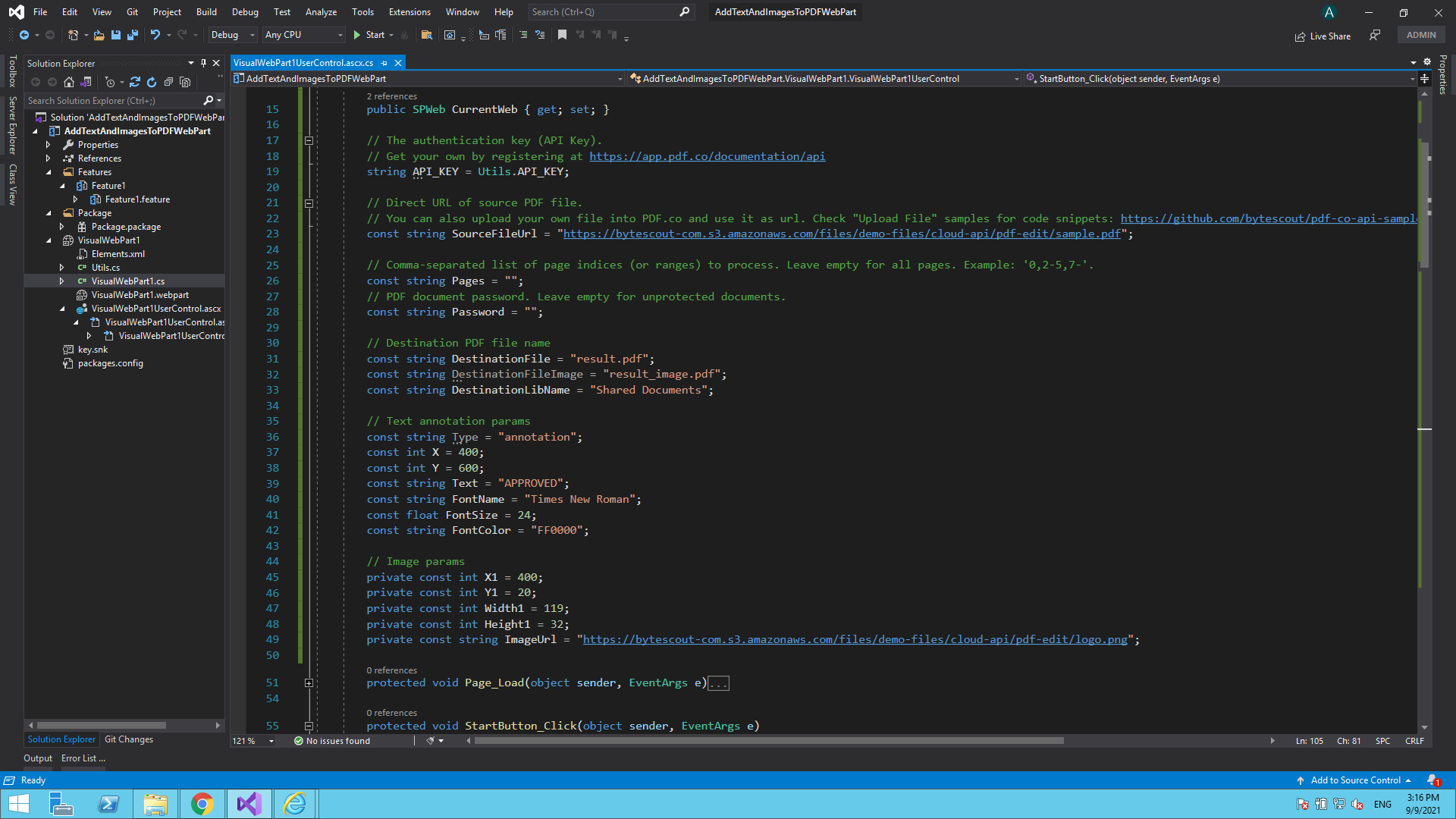
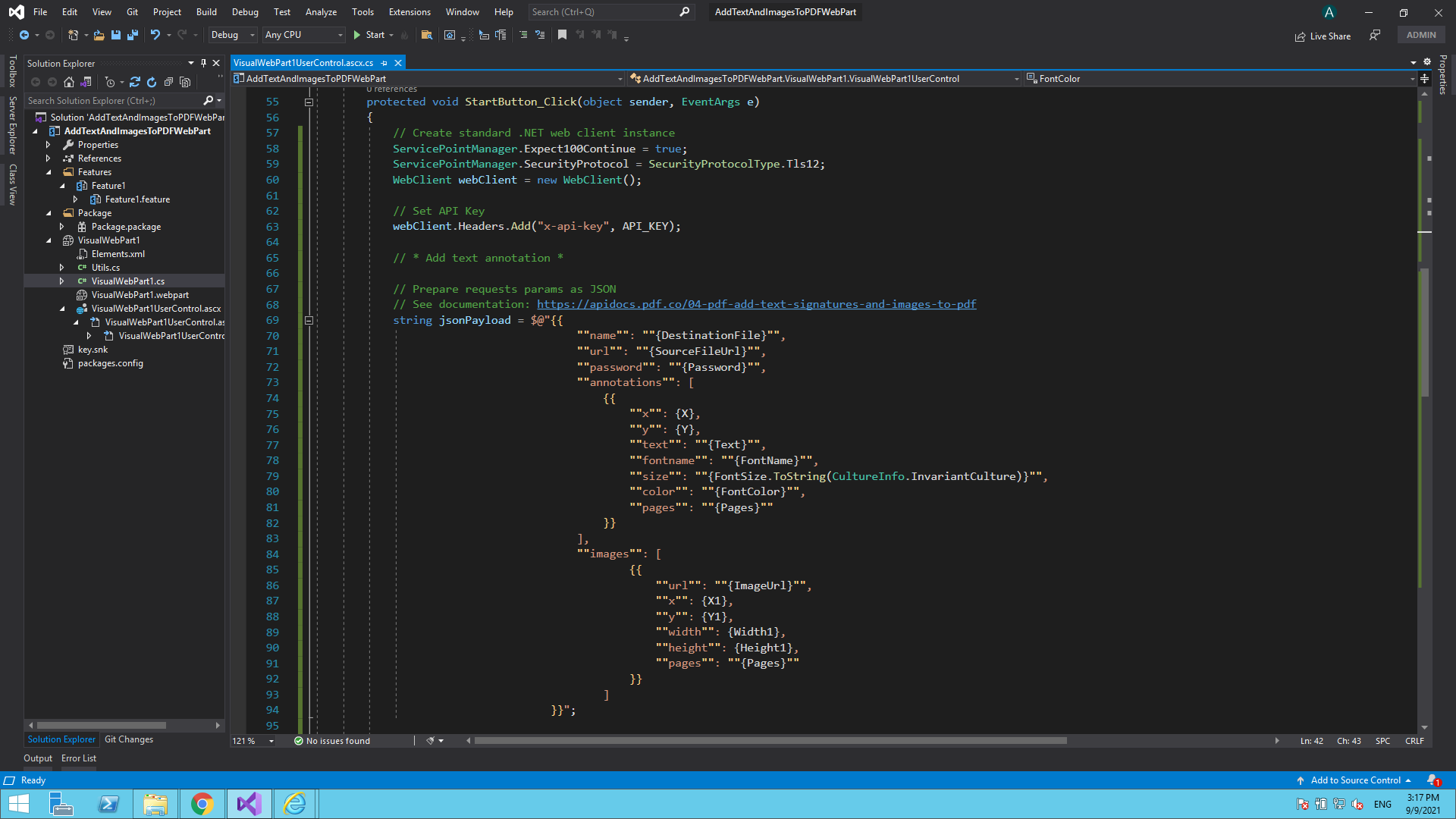
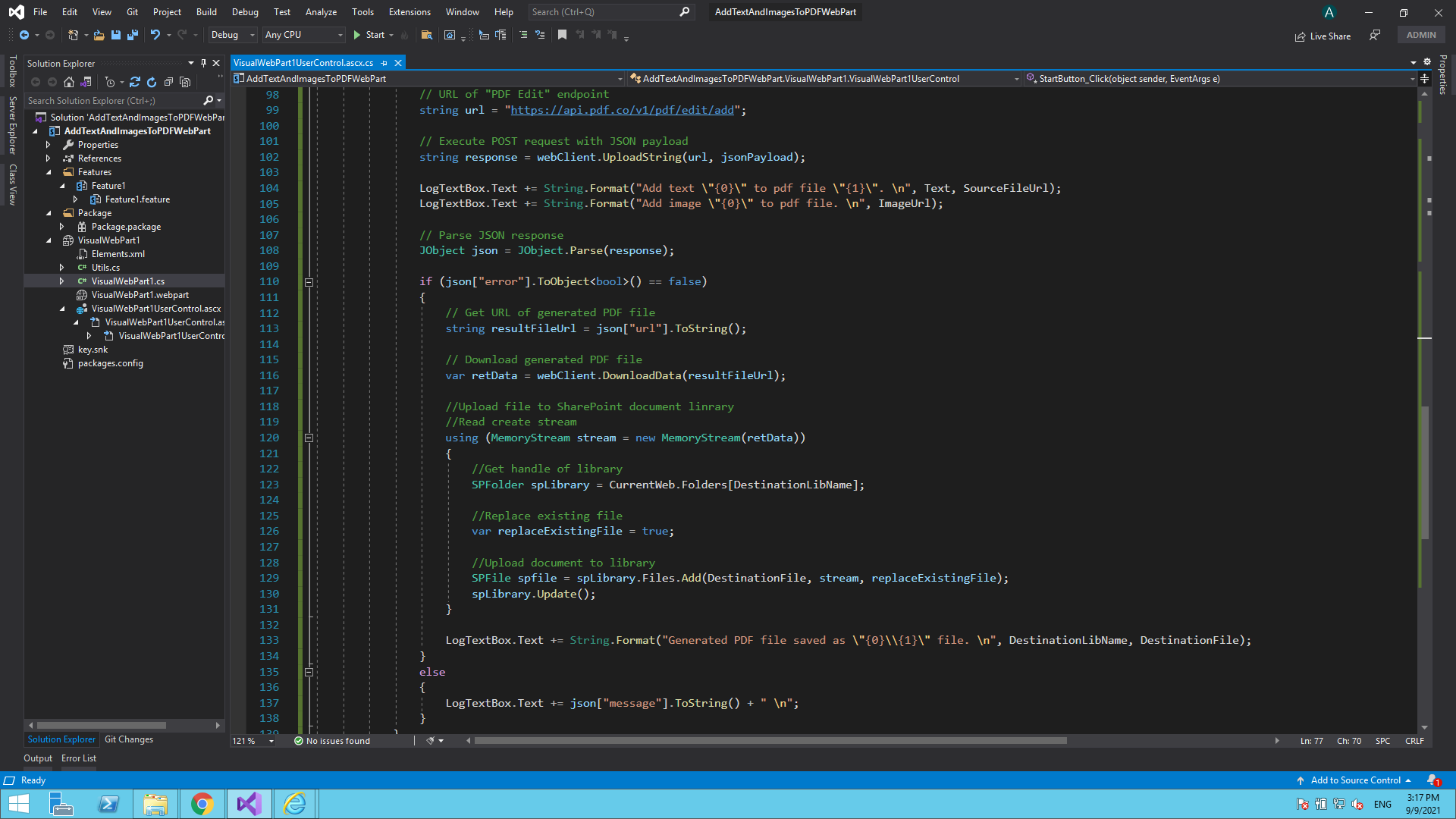
I hope this tutorial and code snippet is useful to you. Please try it yourself to get to know more. Thank You!
Video Guide
Related Tutorials
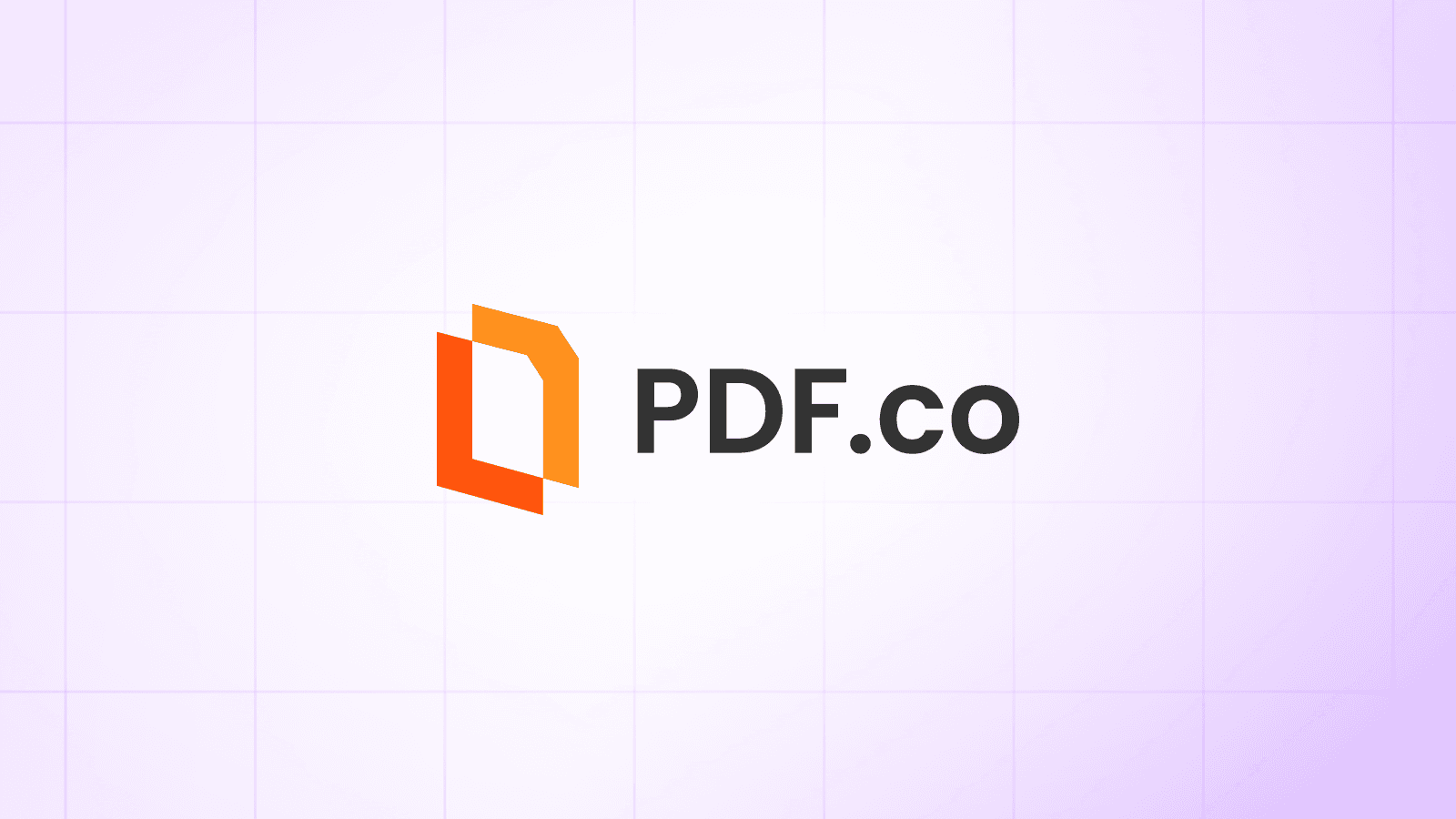
How to Split PDF in Google Drive Folder with Google Apps Script and PDF.co
Sep 2, 2024·6 Minutes Read
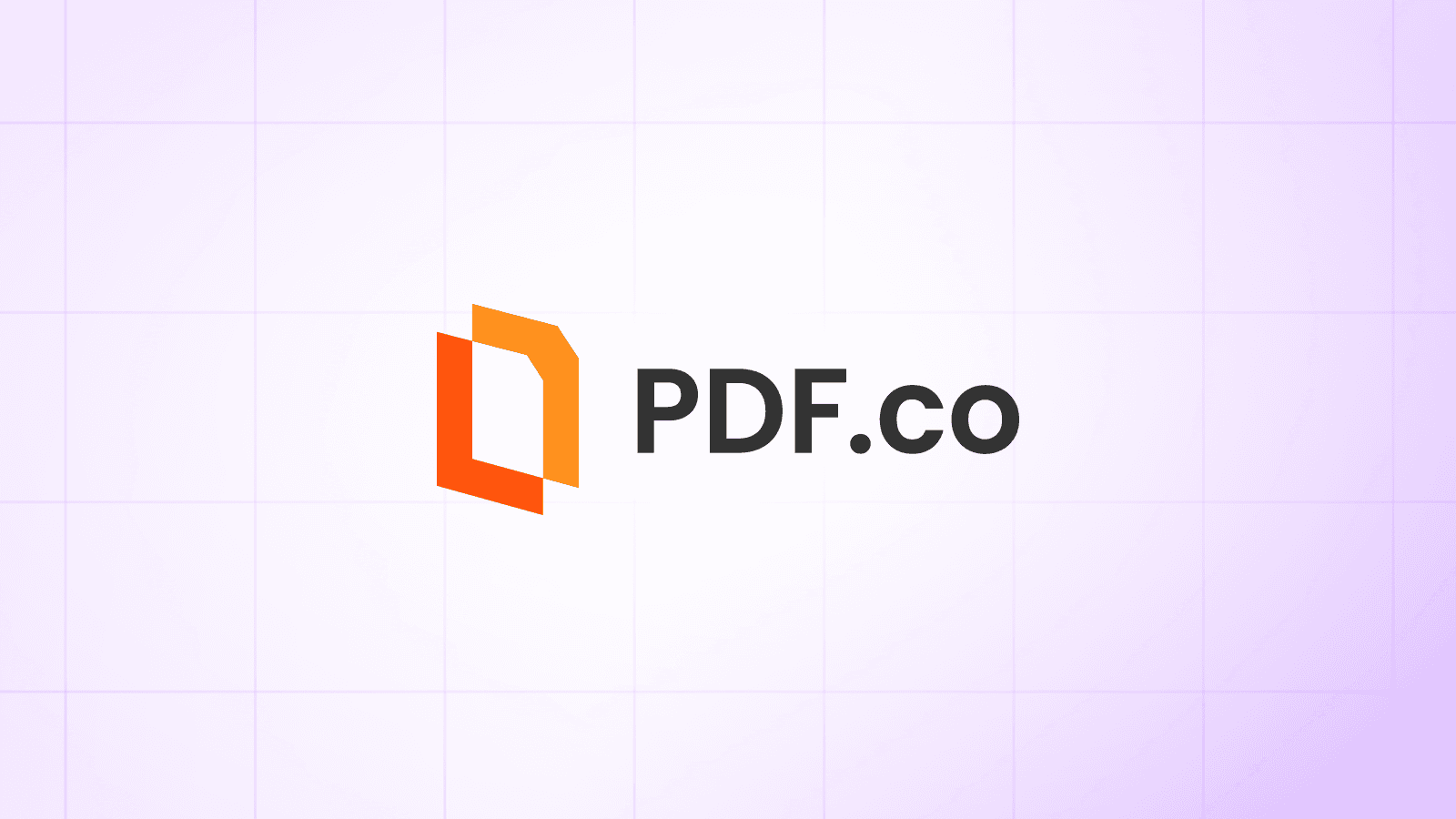
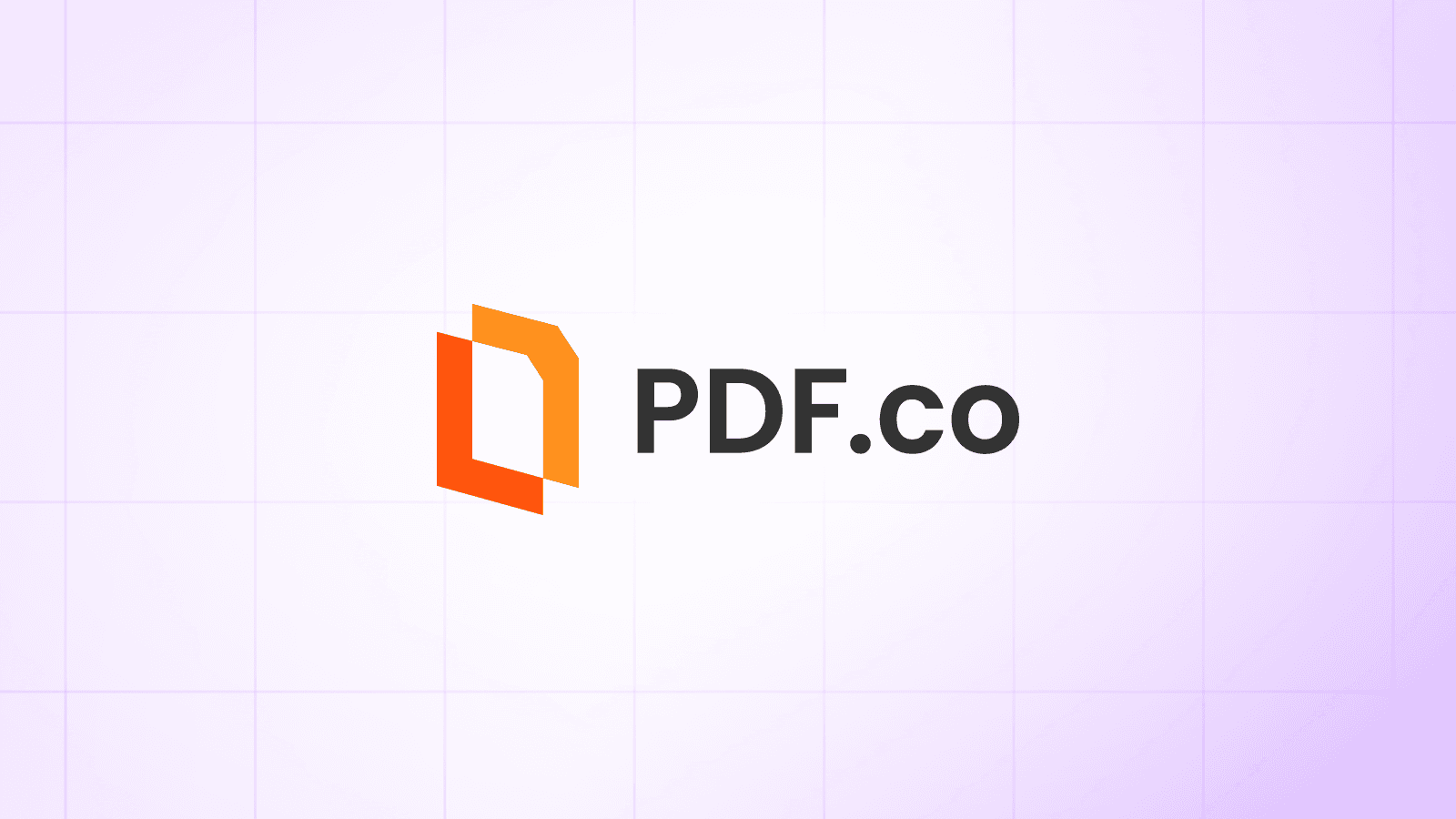
Create PDF with JavaScript using HTML Invoice Template
Sep 2, 2024·15 Minutes Read
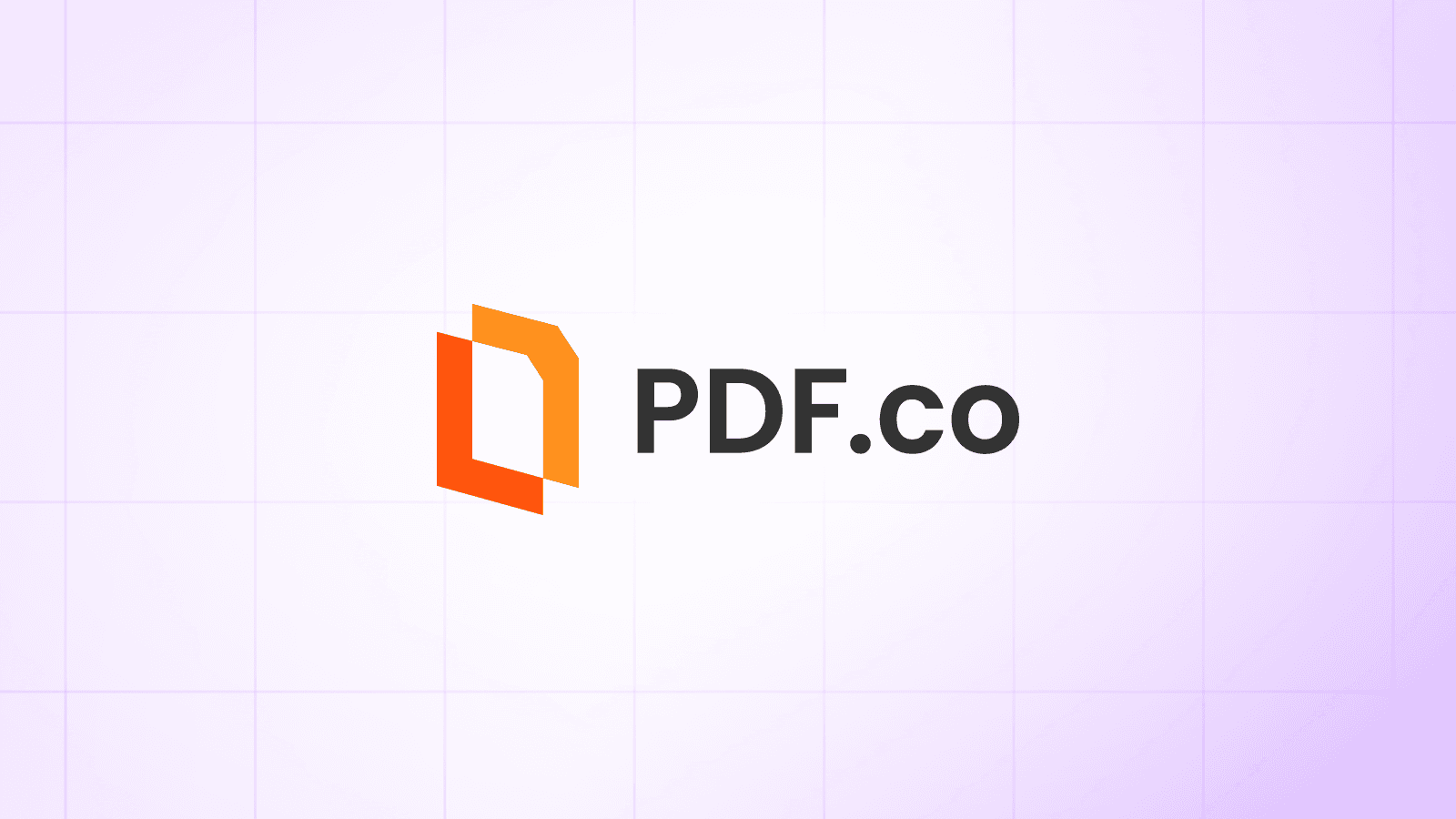
How to Automatically Add New Wufoo entries to PDF using PDF.co and Zapier
Sep 2, 2024·5 Minutes Read