Read Different Barcode Types in C# using PDF.co
In this tutorial, you'll learn how to read various barcode types from a PDF using the PDF.co Barcode Reader Web API in C#.
PDF.co provides powerful tools for PDF processing, such as searching, merging, filling forms, and barcode reading. This guide will walk you through the steps to create a C# console application that extracts barcode data from a PDF.
Create New Project
First, open your terminal or command prompt and run the following command to create a new C# console project inside an "app" folder:
dotnet new console -o app
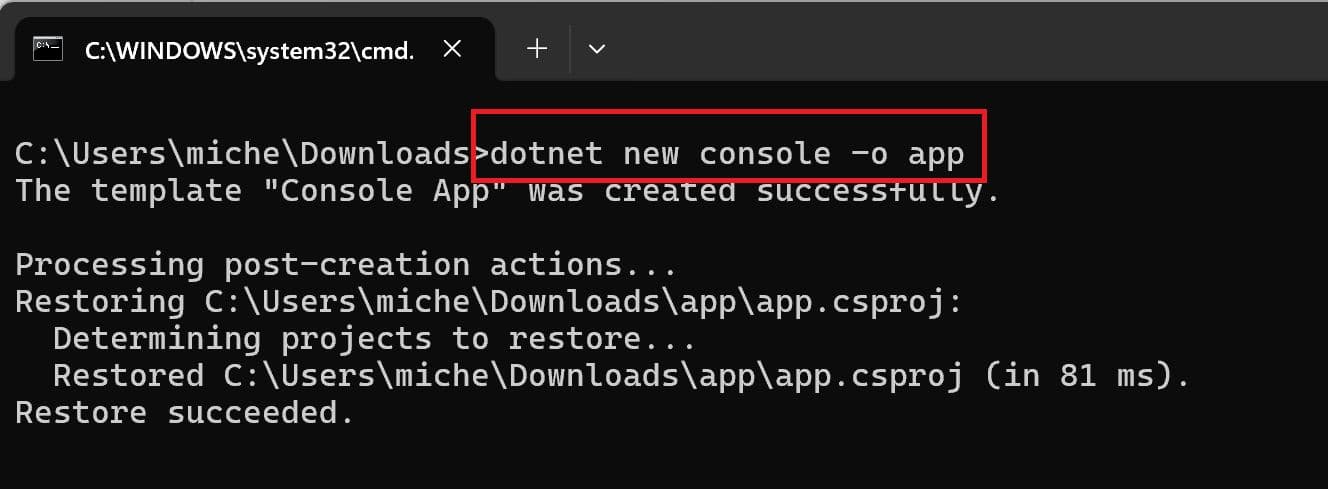
Open VSCode
Type cd app
to go to the folder and enter code .
to open VSCode.
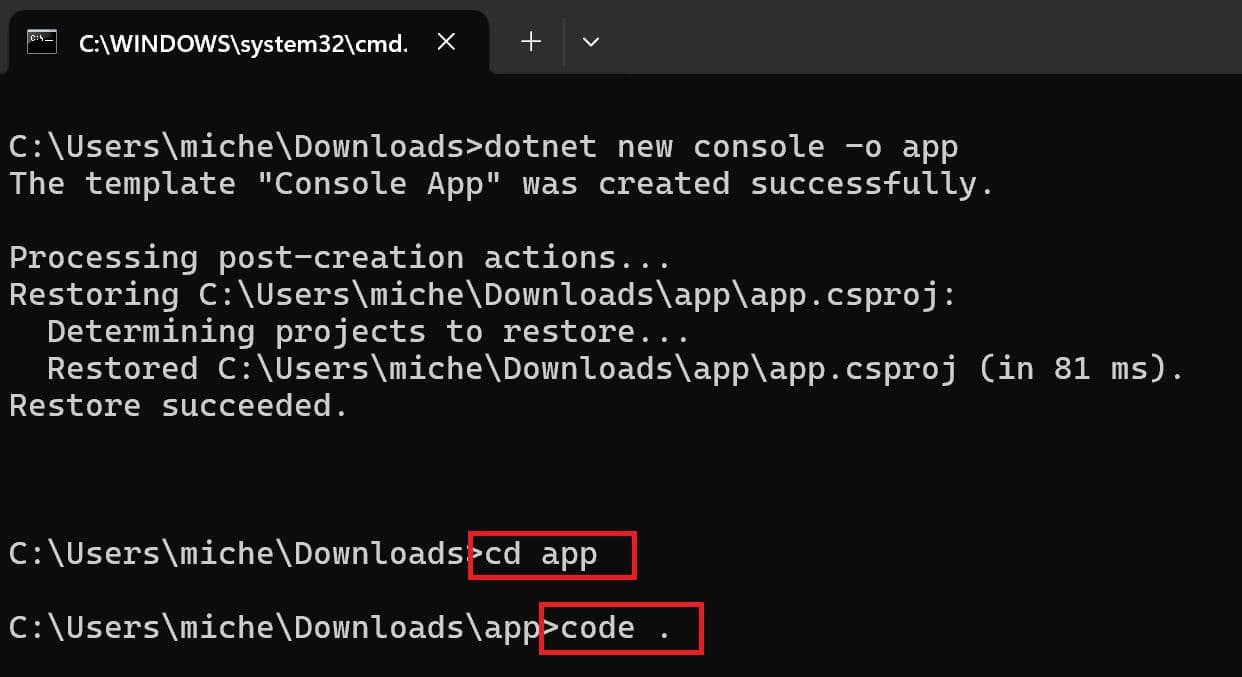
Add Source Code
Copy the Read Barcode from URL (Async) in C# source code from the PDF.co Code Sample Repository.
Add Package
Add the Newtonsoft.Json package, which helps with JSON handling, by running: dotnet add package Newtonsoft.Json
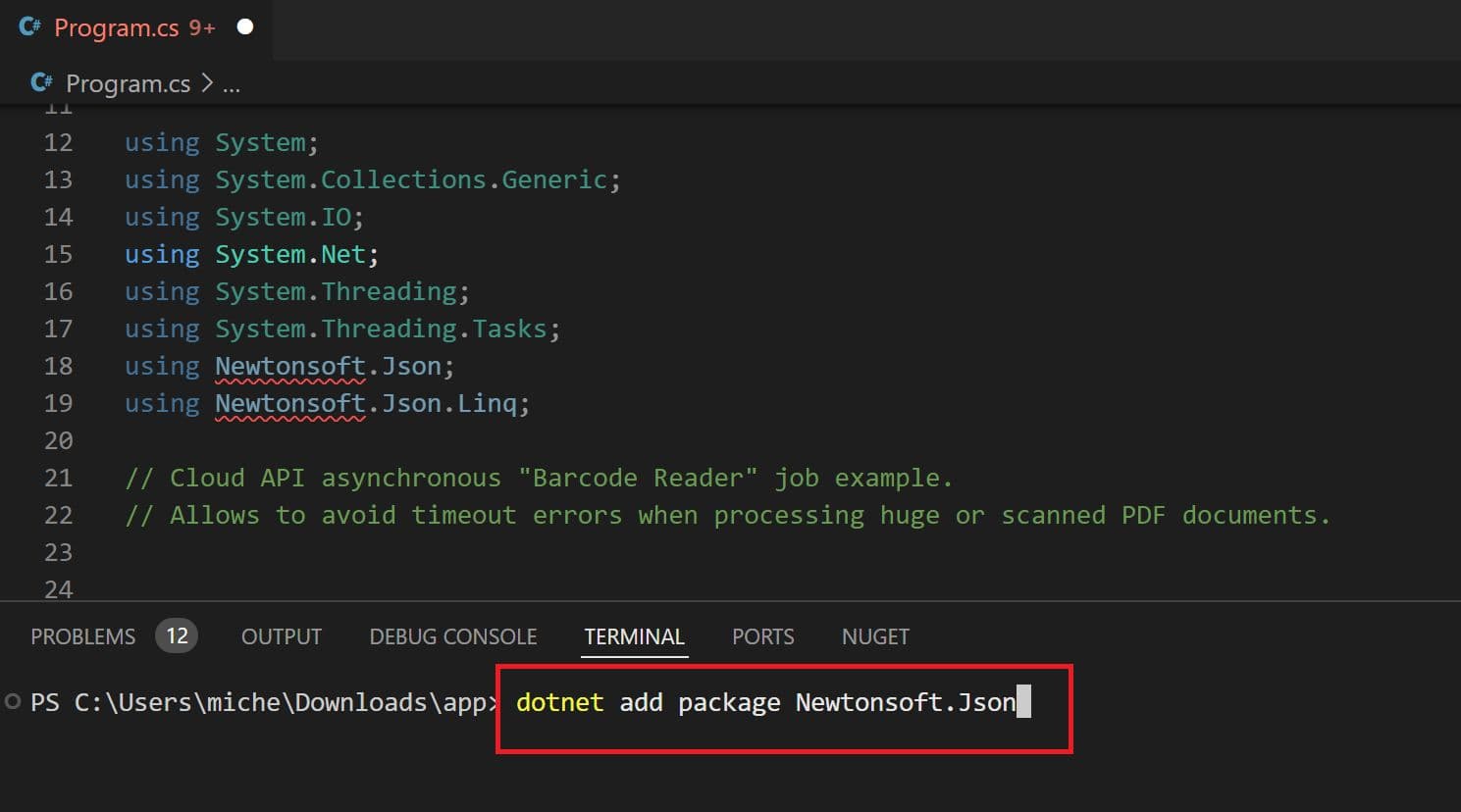
Add API Key
Now, let’s add our PDF.co API Key in line 31. You can get your API Key from the PDF.co dashboard.

Add Source File
In line 35, you can find the PDF URL. If you’d like to test your own file, replace the sample link with your file URL.
If you prefer to work with a file from your local machine, use this code sample instead: Upload File and Read Barcode (C#)

Specify Barcode Type
In line 39, enter one or more barcode types you want to decode. You can find the full list of barcode types here.

Run Project
Execute the following command to run your C# console application: dotnet run
.
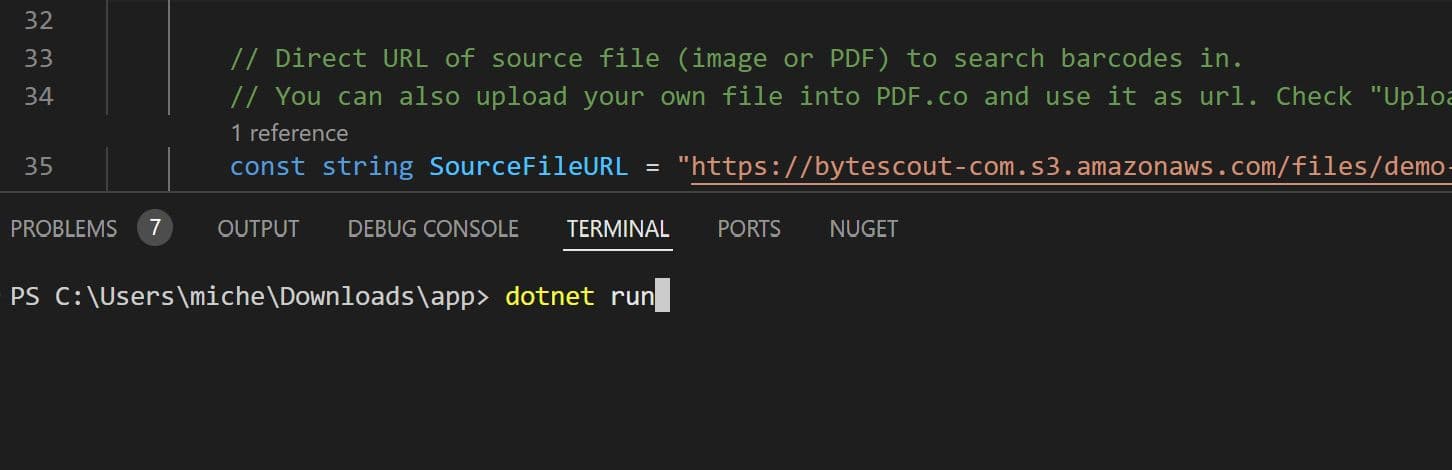
View the Decoded Barcode Values
Once the program runs, you will see the decoded barcode values displayed in the terminal.
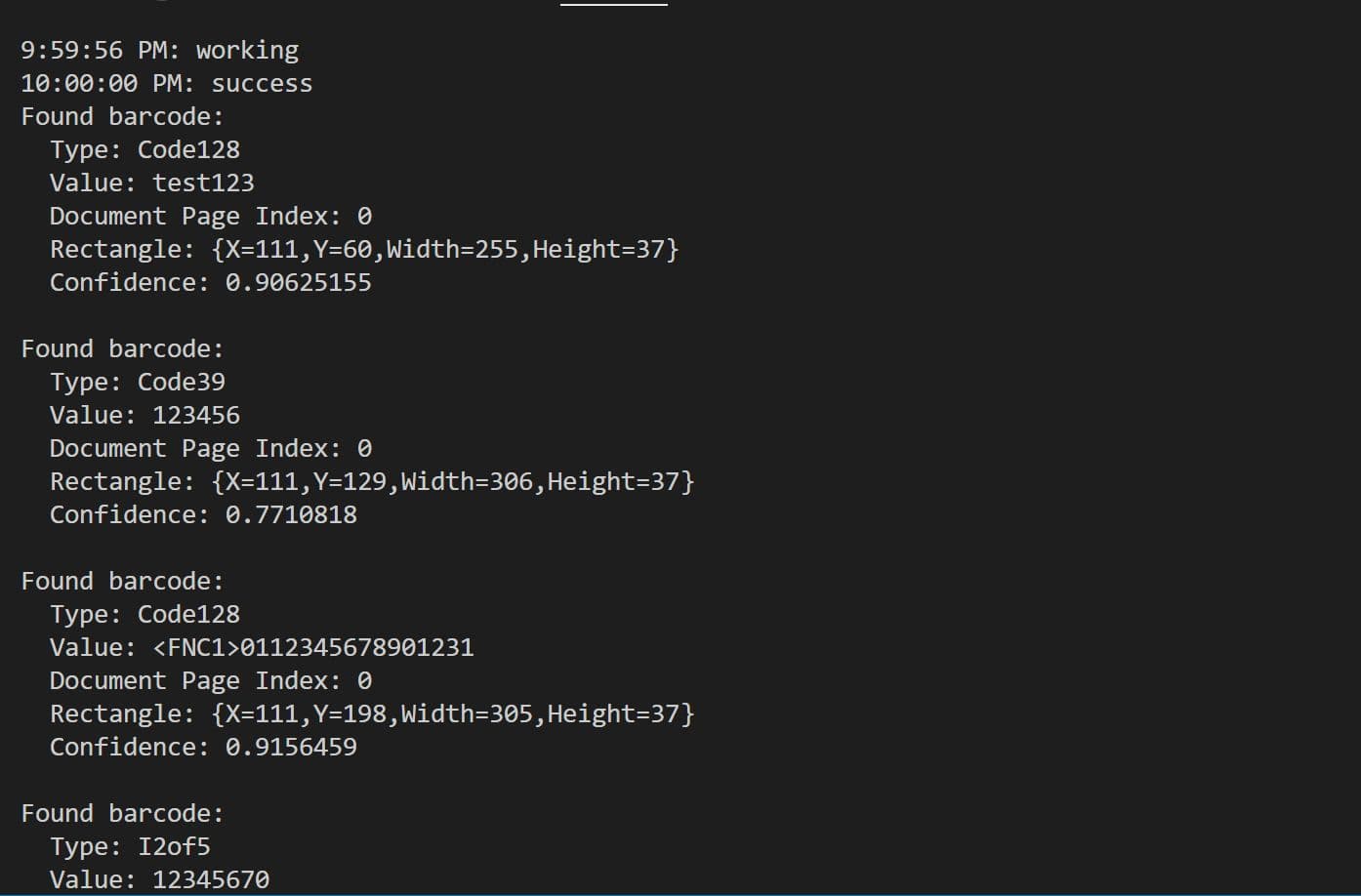
In this tutorial, you learned how to read different Barcode types from a PDF in C# using PDF.co Barcode Reader Web API. You also learned how to:
- Create a new C# project
- Install and use the Newtonsoft.Json package
- Add an API key and customize barcode reading settings
Now you're ready to integrate barcode reading into your C# applications!