How to Delete Pages from PDF in Salesforce using PDF.co
In this step-by-step guide, we’ll be reviewing how to delete pages from the PDF document in Salesforce apex using PDF.co.
IN THIS TUTORIAL
Step 1: Remote Site Settings
Create two remote site settings in the Salesforce like below. The first setting is for the variable name “tempFilesAWS” which will hold the value “https://pdf-temp-files.s3.amazonaws.com”.
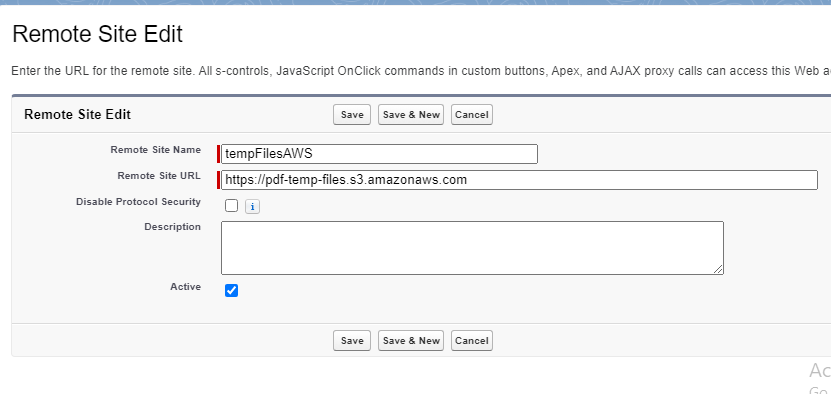
Next will be for the variable name “pdfAPI” which will hold PDF.co API base URL “https://api.pdf.co”.
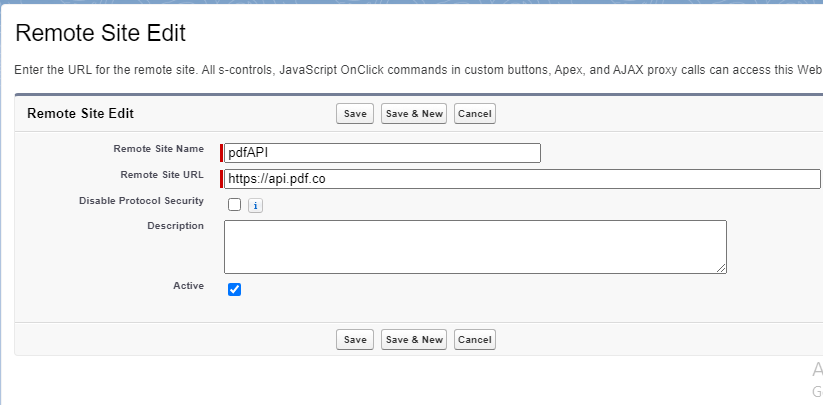
Step 2: Create APEX Class
In this step, we’ll be creating an APEX class in salesforce. For that, we have to open the “Developer Console”.
Developer Console can be opened by clicking on the setting icon in the top right corner.
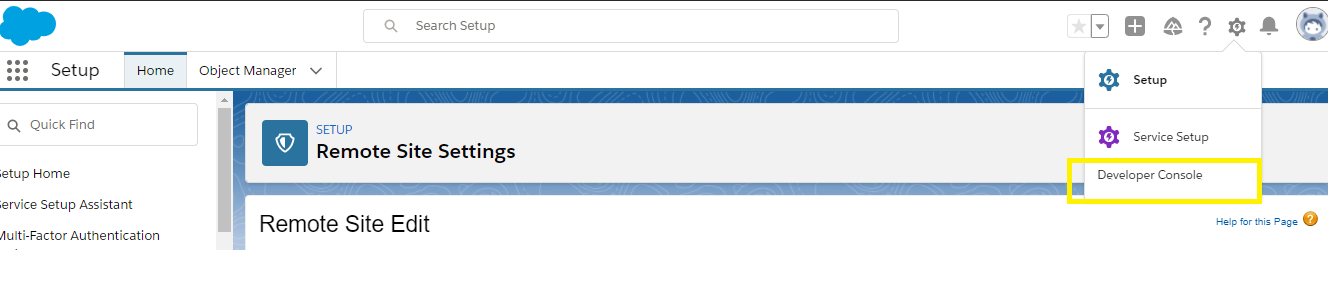
Now that we have “Developer Console” open, we can create an apex class from the “File => New => Apex Class” menu.
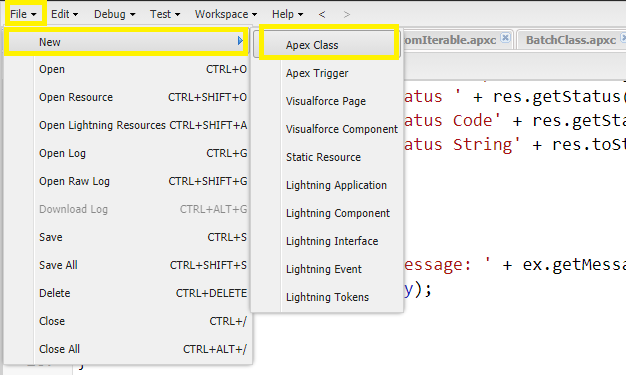
We can name the apex class as “DeletePagesFromPDF” and click “OK”.
Here, we will write the Apex source code which will perform the deletion of PDF pages. The source code is as follows.
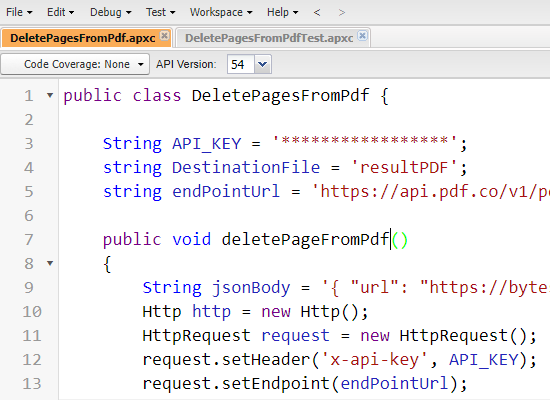
public class DeletePagesFromPdf {
String API_KEY = '*****************';
string DestinationFile = 'resultPDF';
string endPointUrl = 'https://api.pdf.co/v1/pdf/edit/delete-pages';
public void deletePageFromPdf()
{
String jsonBody = '{ "url": "https://bytescout-com.s3.amazonaws.com/files/demo-files/cloud-api/pdf-split/sample.pdf", "pages": "1-2", "name": "result.pdf", "async": false }';
Http http = new Http();
HttpRequest request = new HttpRequest();
request.setHeader('x-api-key', API_KEY);
request.setEndpoint(endPointUrl);
request.setHeader('Content-Type', 'application/json');
request.setMethod('POST');
request.setBody(jsonBody);
HttpResponse response = http.send(request);
Map<String, Object> json = (Map<String, Object>)JSON.deserializeUntyped(response.getBody());
try
{
if(response.getStatusCode() == 200)
{
if ((Boolean)json.get('error') == false)
{
System.debug('response.getBody() :: '+response.getBody());
// Get URL of generated PDF file
String resultFileUrl =(String)json.get('url');
// Download generated PDF file
downloadFile(resultFileUrl, DestinationFile);
System.debug('Generated PDF file saved as \'{0}\' file.'+ DestinationFile);
}
}
else
{
System.debug('Error Response ' + response.getBody());
System.Debug(' Status ' + response.getStatus());
System.Debug(' Status Code' + response.getStatusCode());
System.Debug(' Response String' + response.toString());
}
}
catch (Exception ex)
{
String errorBody = 'Message: ' + ex.getMessage() + ' -- Cause: ' + ex.getCause() + ' -- Stacktrace: ' + ex.getStackTraceString();
System.Debug(errorBody);
}
}
@TestVisible
private static void downloadFile(String extFileUrl, String DestinationFile)
{
Http h = new Http();
HttpRequest req = new HttpRequest();
extFileUrl = extFileUrl.replace(' ', '%20');
req.setEndpoint(extFileUrl);
req.setMethod('GET');
req.setHeader('Content-Type', 'application/pdf');
req.setCompressed(true);
req.setTimeout(60000);
//Now Send HTTP Request
HttpResponse res = h.send(req);
if(res.getStatusCode() == 200)
{
blob fileContent = res.getBodyAsBlob();
ContentVersion conVer = new ContentVersion();
conVer.ContentLocation = 'S'; // to use S specify this document is in Salesforce, to use E for external files
conVer.PathOnClient = DestinationFile + '.pdf'; // The files name, extension is very important here which will help the file in preview.
conVer.Title = DestinationFile; // Display name of the files
conVer.VersionData = fileContent;
insert conVer;
System.Debug('Success');
}
else
{
System.debug('Error Response ' + res.getBody());
System.Debug(' Status ' + res.getStatus());
System.Debug(' Status Code' + res.getStatusCode());
System.Debug(' Response String' + res.toString());
}
}
}
Please note that we have to replace the value of the “API_KEY” variable with PDF.co API Key. As you have noticed in the source code, the PDF.co API key is passed in the request header. This API key is useful for the authentication of PDF.co requests.
This API Key can be obtained by signing up at PDF.co.
Like we created the main apex class for “DeletePagesFromPDF”, we can also create a test apex class named “DeletePagesFromPdfTest”. In this test class, we can write the following unit test code.
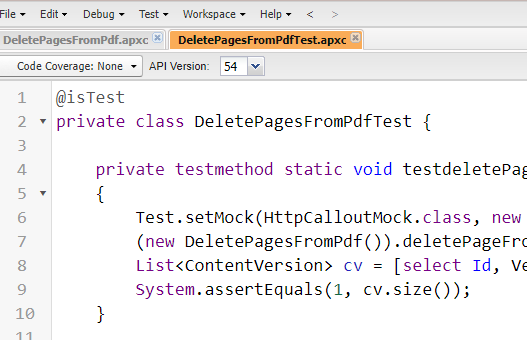
@isTest
private class DeletePagesFromPdfTest {
private testmethod static void testdeletePageFromPdf()
{
Test.setMock(HttpCalloutMock.class, new DeletePagesFromPdfTest.DeletePageMock());
(new DeletePagesFromPdf()).deletePageFromPdf();
List cv = [select Id, VersionData from ContentVersion];
System.assertEquals(1, cv.size());
}
private testmethod static void testdeletePageFromPdfException()
{
Test.setMock(HttpCalloutMock.class, new DeletePagesFromPdfTest.DeletePageMockForExp());
(new DeletePagesFromPdf()).deletePageFromPdf();
List cv = [select Id from ContentVersion];
System.assertEquals(0, cv.size());
}
public class DeletePageMock implements HttpCalloutMock {
public HTTPResponse respond(HTTPRequest req) {
HttpResponse res = new HttpResponse();
String testBody = '{ "url": "https://pdf-temp-files.s3.amazonaws.com/d15e5b2c89c04484ae6ac7244ac43ac2/result.pdf", "pageCount": 2, "error": false, "status": 200, "name": "result.pdf", "remainingCredits": 60100 } ';
res.setHeader('Content-Type', 'application/json');
res.setBody(testBody);
res.setStatusCode(200);
return res;
}
}
public class DeletePageMockForExp implements HttpCalloutMock {
public HTTPResponse respond(HTTPRequest req) {
HttpResponse res = new HttpResponse();
String testBody = '{ "url1111": "https://pdf-temp-files.s3.amazonaws.com/d15e5b2c89c04484ae6ac7244ac43ac2/result.pdf", "pageCount": 2, "error": false, "status": 200, "name": "result.pdf", "remainingCredits": 60100 } ';
res.setHeader('Content-Type', 'application/json');
res.setBody(testBody);
res.setStatusCode(200);
return res;
}
}
}
Step 3: Test Code
Up to this step, we have written all source code. We’ll be proceeding with testing it now. Open the execute anonymous window and write executor code there.
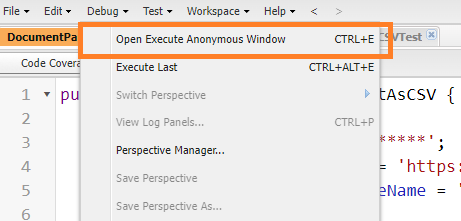
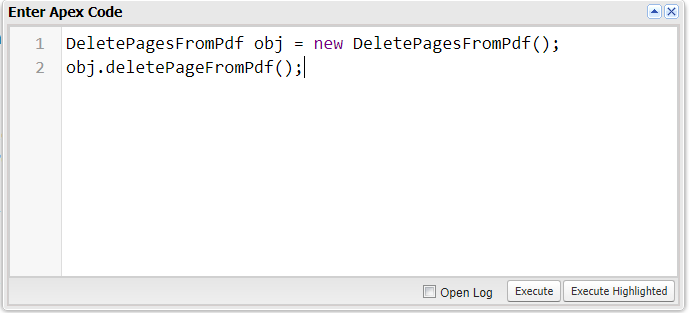
DeletePagesFromPdf obj = new DeletePagesFromPdf();
obj.deletePageFromPdf();
Click on the “Execute” button which will run the source code.
Step 4: Verify Code
Let’s verify how the source code works. This code will eventually create a new PDF with deleted pages, hence we need to check the newly created file. Open “Files” from “App Launcher”.
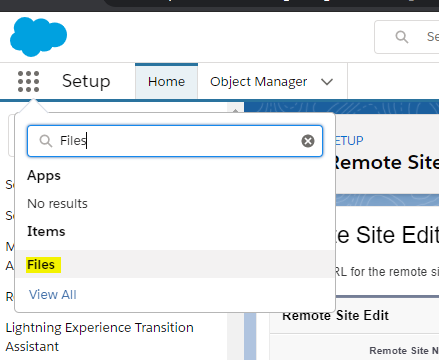
In the file listing window, we’re able to find the result PDF file.

Useful Resources
Please try this sample on your own and evaluate the results for a better understanding. Thank you!
Video Guide
Related Tutorials
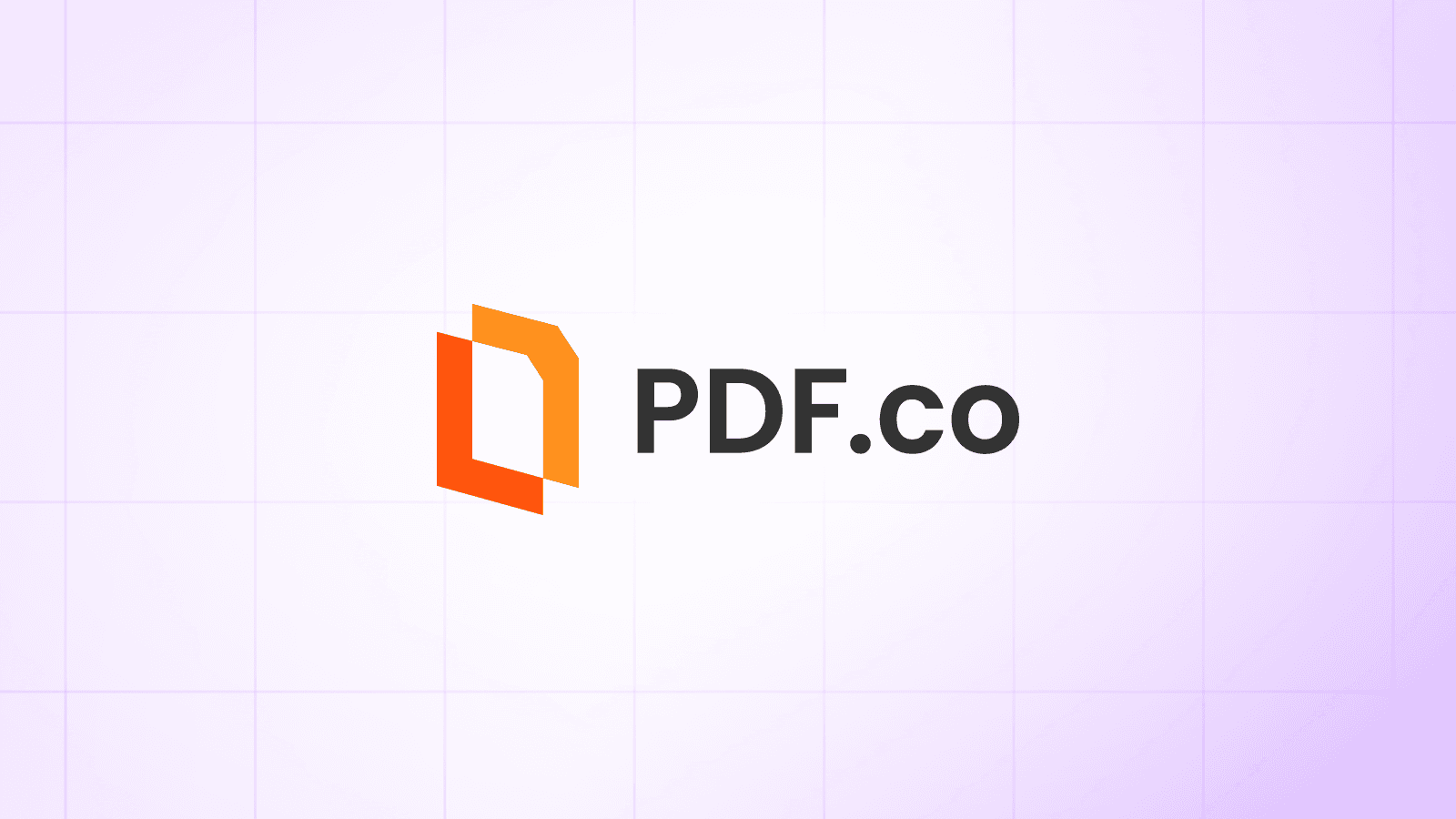
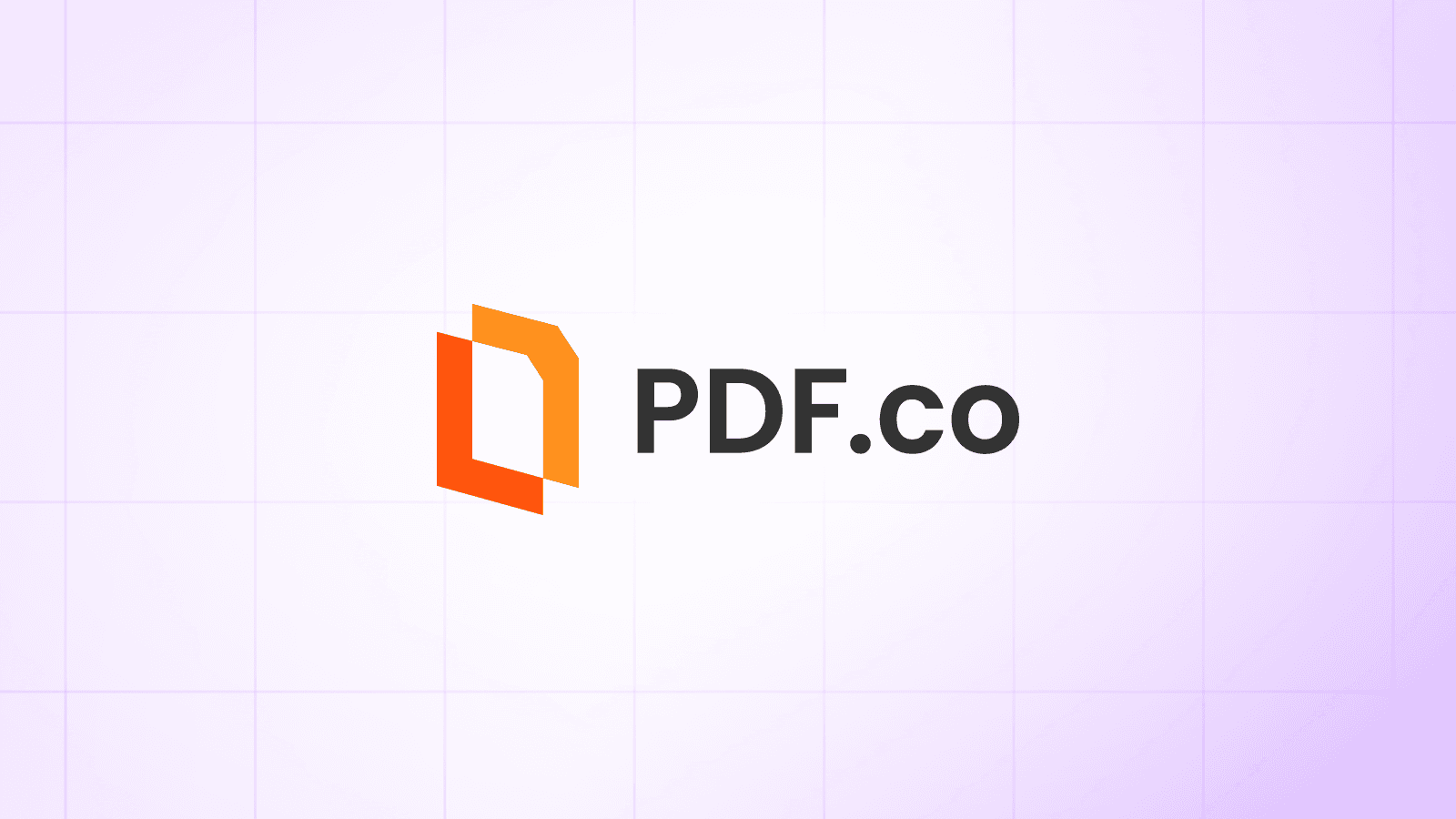
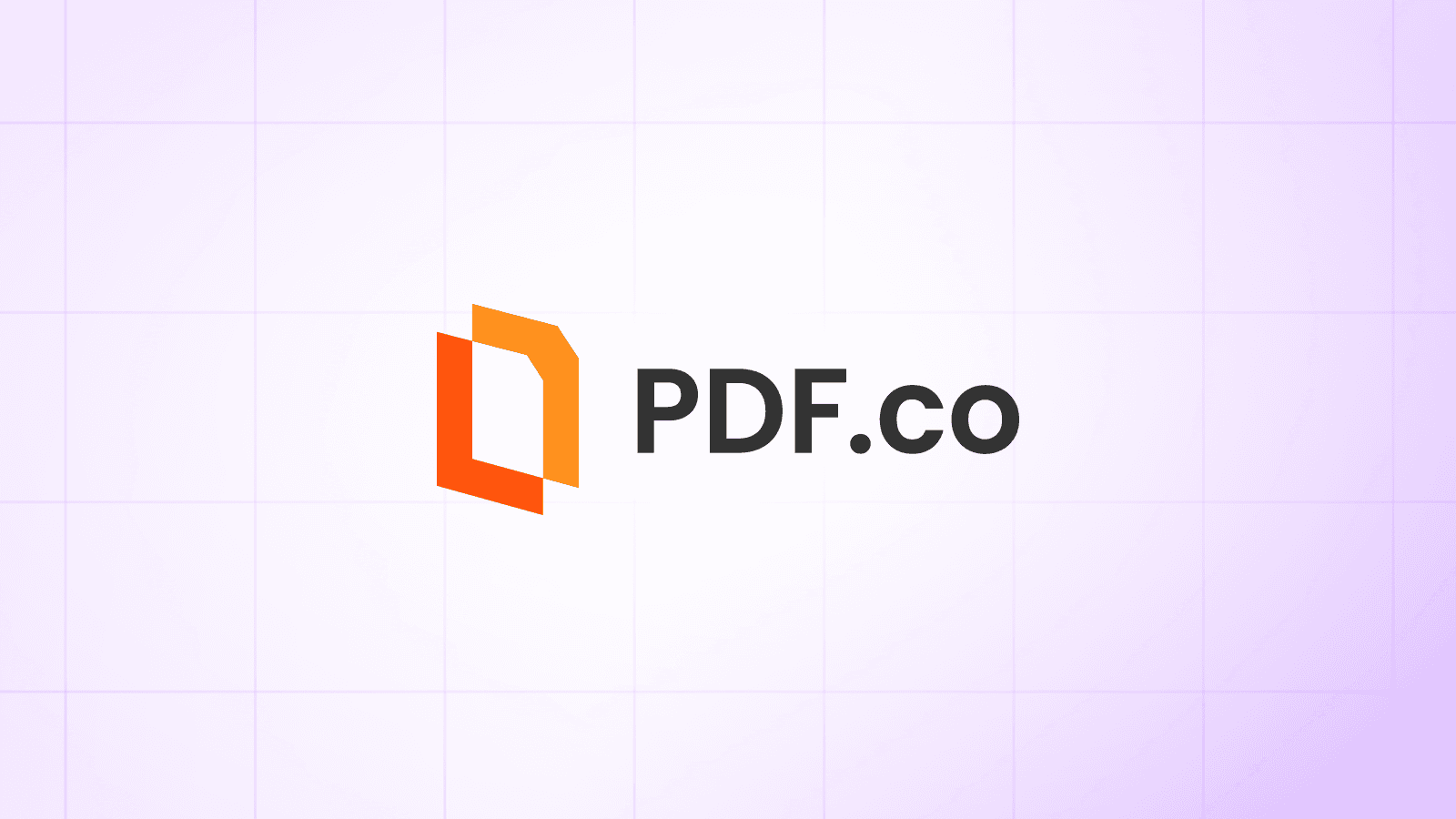
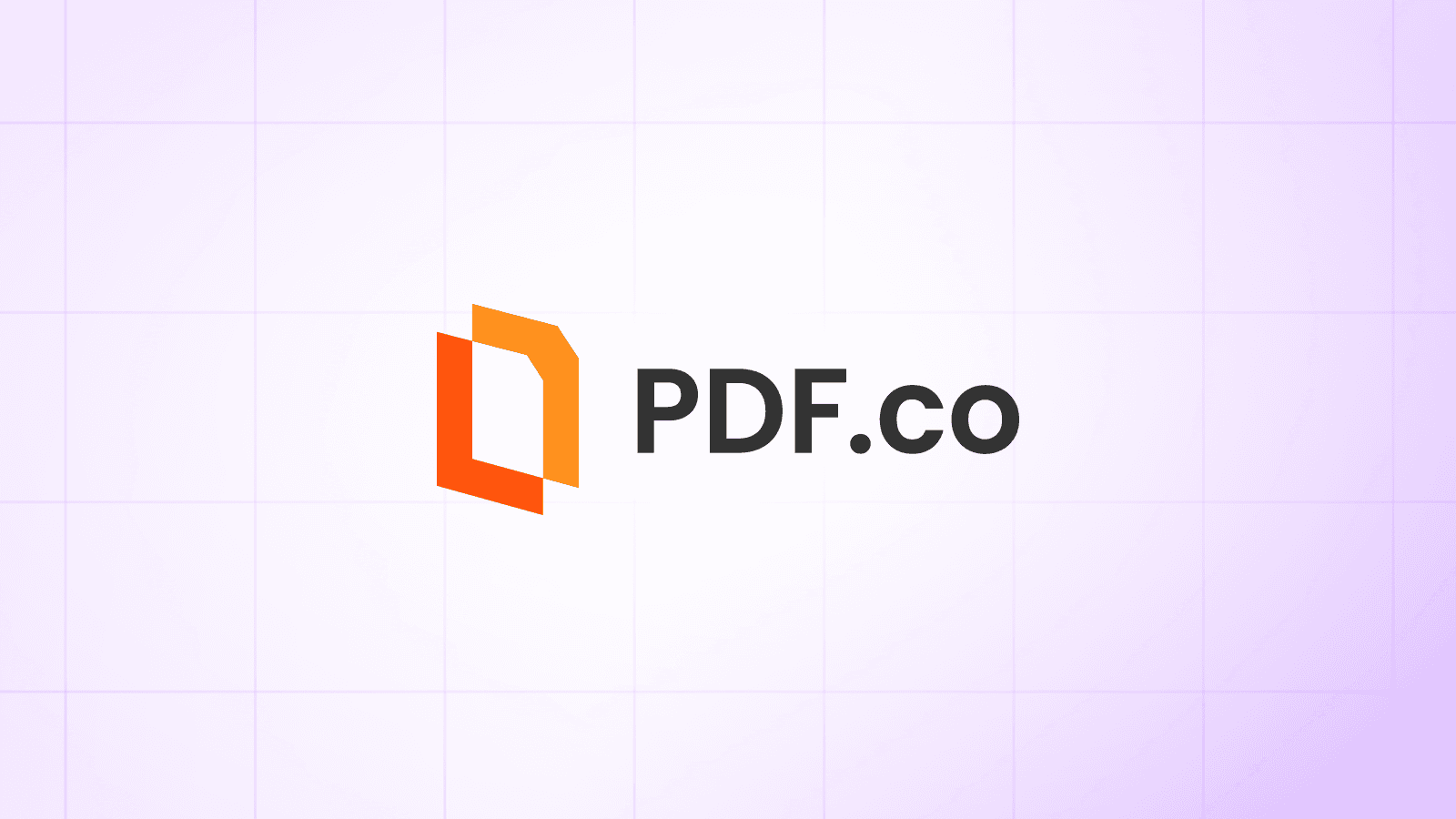