Solutions for Developers
PDF.co solutions for developers help boost workflows and decrease costs on manual labor, by data being structured, processed, and exported.
How PDF.co Can Help Developers
- No commitment, create free account to try how it works on your documents;
- Copy-paste code from thousands of source code samples for almost all programming languages and platforms;
- Enjoy customer support from experts focused on helping developers;
- Almost everything you may need for PDF on one single platform: from generating PDF to reading from unstructured documents;
- Detailed API documentation and a large knowledge base of tips and tricks.
Our biggest customers
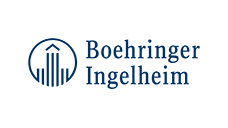
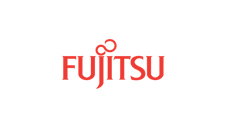
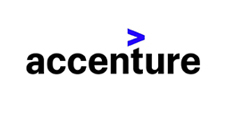
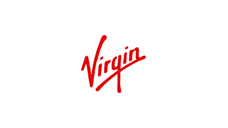
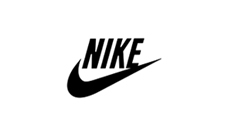
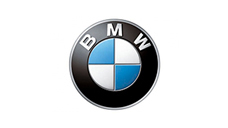
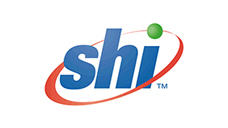
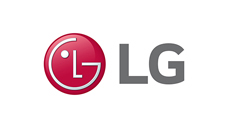
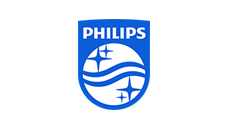
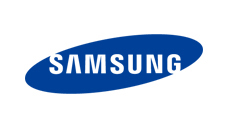
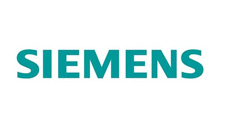
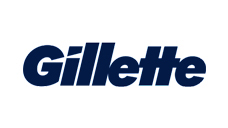
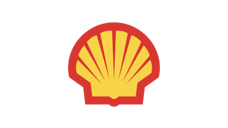
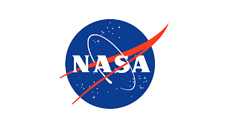
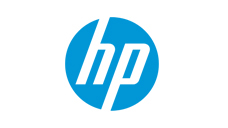
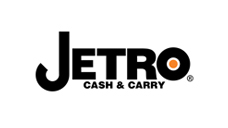
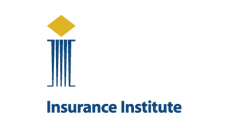
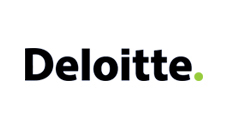
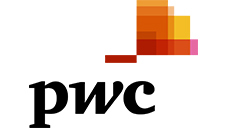
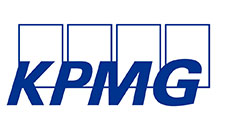
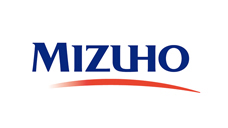
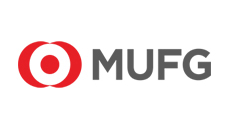
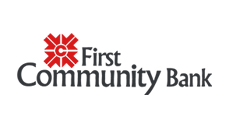
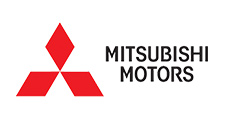
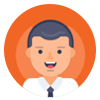
Thanks for your help, from a very happy customer!
I had this problem [Word Doc (on Dropbox) to PDF conversion] and you have responded swiftly and fixed it. I am so chuffed to get this part of my workflow working and really grateful for the extra credits (…the icing on the cake). Thanks for your help, from a very happy customer!
Allan W., Solutions Architect
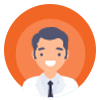
Finally found PDF.co and am very happy now!
I’ve been struggling with PDF documents [reading text from PDF documents and searching for a text inside], we use a lot of different ones, and it’s always been difficult to find one API and that can do everything we need at an affordable price. Well, I’ve just stumbled across PDF.co [PDF.co Document Parser and PDF text search], what a difference it’s made! I’m sure most of you know it, but if you don’t and you want to manipulate PDFs – check it out. I’m doing stuff automatically that I wasn’t able to do manually with Adobe Acrobat. I wish I’d found it earlier.
Howard L. J. (source: Facebook group)
Automate & Speed Up Workflows!